How to Change the Permissions of a File or a Directory in PHP
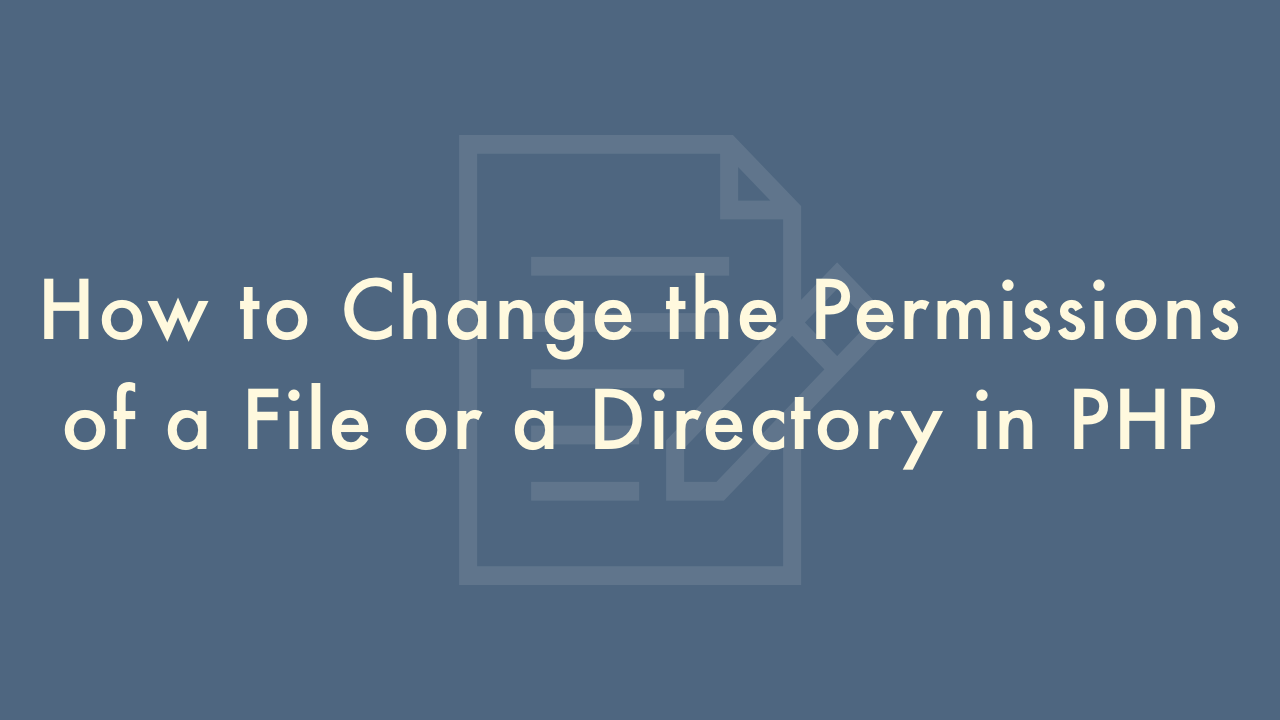
Contents
In this article, you will learn how to change the permissions of a file or a directory in PHP.
PHP chmod() Function
To change the permissions of a file or a directory in PHP, you can use the chmod() function.
The chmod() function returns TRUE on success and FALSE on failure.
Syntax:
bool chmod ( string $filename , int $mode );
Parameters:
The chmod() function takes two parameters:
$filename
: The path to the file or directory whose permissions you want to change. This parameter is required.$mode
: The new permissions to be set, represented as an octal number. This parameter is required.
Example:
Here’s an example to change the permissions of a file to 644 (readable and writable by owner, readable by others):
<?php
chmod("/path/to/file.txt", 0644);
?>
And here’s an example to change the permissions of a directory to 755 (readable, writable, and executable by owner, readable and executable by others):
<?php
chmod("/path/to/directory", 0755);
?>
It’s important to note that the chmod function may not work on all server configurations, depending on the server’s security settings. Also, the PHP process must have sufficient permissions to change the file permissions.