How to Prevent Multiple Form Submissions in PHP
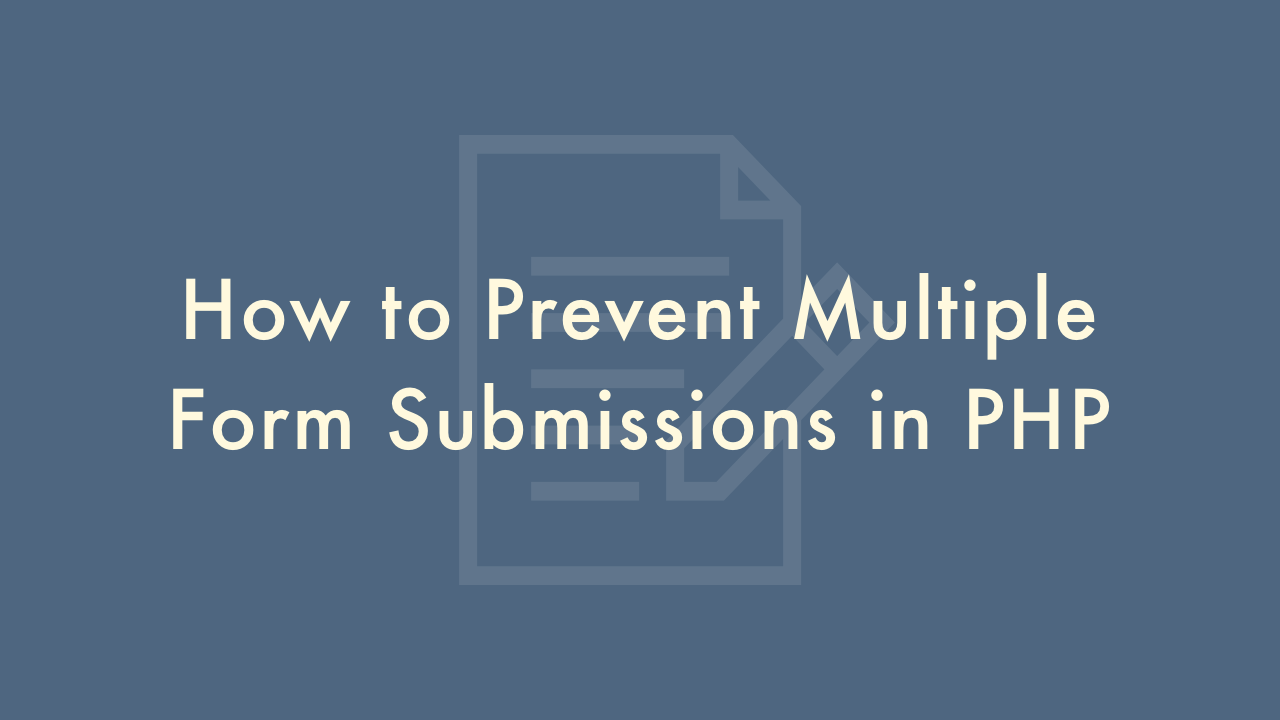
09/07/2021
Contents
In this article, you will learn how to prevent multiple form submissions in PHP.
Prevent Multiple Form Submissions
Preventing multiple form submissions in PHP can be done using the following methods:
- Token-Based Approach: Generate a unique token for each form, store it in the session, and check it against the submitted data. If the tokens match, process the form data, otherwise discard it.
- Timestamp-Based Approach: Store the current time as a timestamp in the session when the form is loaded and check it against the submitted data. If the submission is made within a specified time interval, process the form data, otherwise discard it.
- Lock and Unlock Approach: Set a session variable to lock the form when it is submitted and unlock it after processing the data. Check the status of the session variable before processing the form data.
Example code for the Token-Based Approach:
<?php
session_start();
if(isset($_POST['submit'])) {
if($_SESSION['token'] == $_POST['token']) {
// process form data
unset($_SESSION['token']);
} else {
// discard form data
echo "Invalid request";
}
} else {
// generate a new token and store it in the session
$_SESSION['token'] = md5(uniqid(rand(), true));
}
?>
<form action="" method="post">
<input type="hidden" name="token" value="<?php echo $_SESSION['token']; ?>">
<!-- form fields go here -->
<input type="submit" name="submit" value="Submit">
</form>