How to Detect Mobile Device or Desktop in PHP
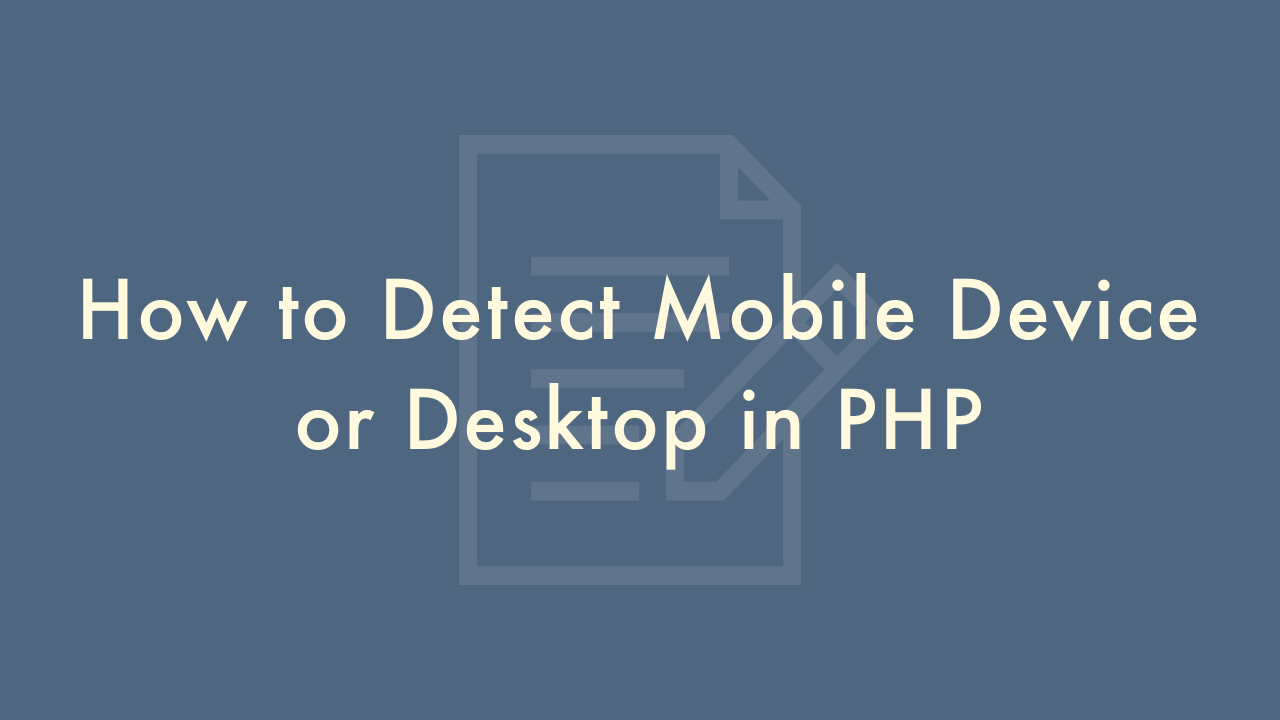
Contents
In this article, you will learn how to detect mobile device or desktop in PHP.
Detect Mobile Device or Desktop
You can use the $_SERVER[‘HTTP_USER_AGENT’] variable to determine the type of device that is accessing your website. The user agent string will contain information about the device’s operating system, browser, and version. You can use string matching to detect the presence of keywords that are commonly associated with mobile devices, and use that information to determine whether the user is on a mobile device or desktop computer.
Here is an example:
<?php
function isMobile() {
return preg_match("/(android|avantgo|blackberry|bolt|boost|cricket|docomo|fone|hiptop|mini|mobi|palm|phone|pie|tablet|up\.browser|up\.link|webos|wos)/i", $_SERVER["HTTP_USER_AGENT"]);
}
if (isMobile()) {
// User is on a mobile device
} else {
// User is on a desktop computer
}
?>
Keep in mind that user agent strings can be easily spoofed, so this method is not foolproof. But, it is a good starting point for detecting the device type.
The user agent string is a string of text that is sent by the browser to the server with each HTTP request. It contains information about the device and browser that is making the request. By checking the user agent string, you can determine the type of device that is accessing your website, and tailor your website’s content and functionality accordingly.
However, user agent strings are not standardized and can be easily spoofed, so relying solely on the user agent string to determine the device type is not always accurate. Some developers prefer to use other methods to detect the device type, such as checking the screen resolution or using JavaScript to detect the presence of certain features that are commonly associated with mobile devices.
If you want a more reliable method of detecting the device type, you can use a library like Mobile_Detect, which is a PHP class that uses a comprehensive set of detection rules to accurately determine the device type. This library is based on the user agent string, but it also uses other methods, such as checking the screen resolution, to provide a more reliable result.
It’s worth noting that mobile-first design is a popular approach, which means that the website is optimized for mobile devices first and then adjusted for desktop devices. In this approach, it’s not necessary to detect the device type, but rather to make the website responsive to different screen sizes and resolutions.