How to Use the PHP array_map() Function
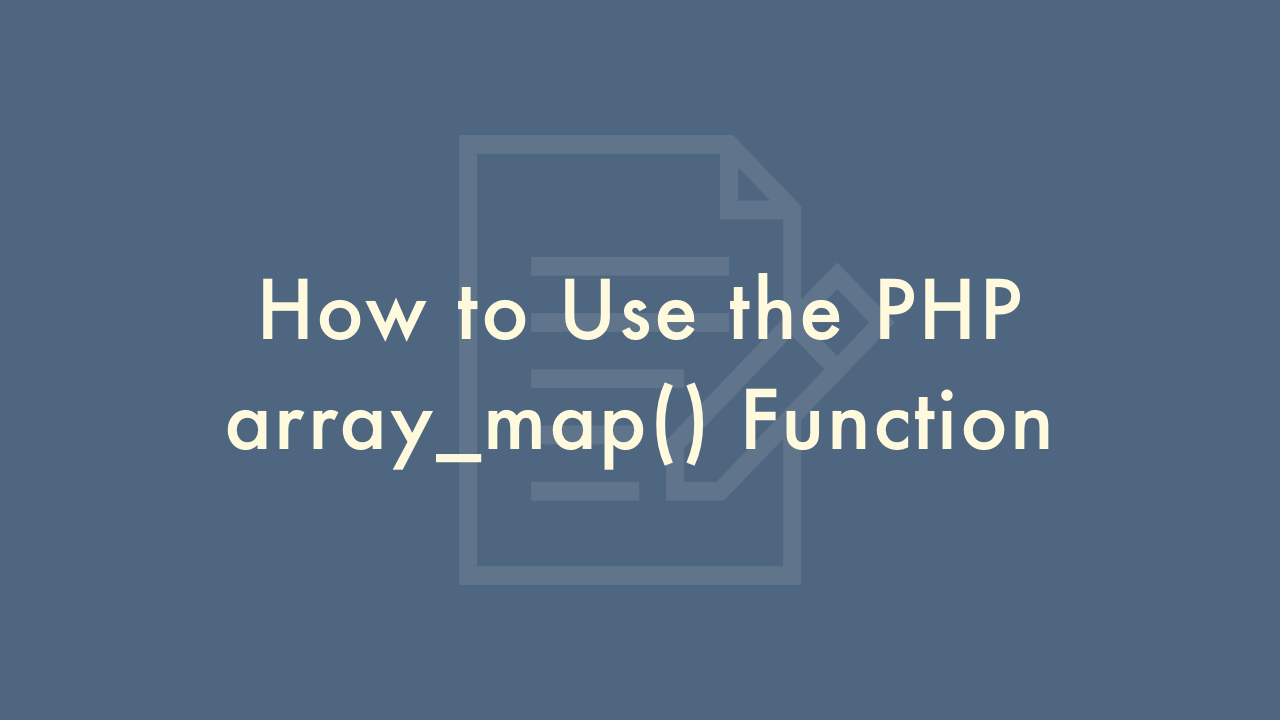
Contents
In this article, you will learn how to use the PHP array_map() function.
PHP array_map() Function
he array_map() function in PHP is used to apply a callback function to each element of an array. It returns an array with the same number of elements after applying the callback to each element.
Syntax:
array array_map ( callable $callback , array $array1 [, array $... ] )
Parameters:
$callback
: The function to apply to each element of the array. This can be an anonymous function, or a named function.$array1
: The first array to apply the function to.[, array $... ]
: Optional additional arrays. The callback function will be applied to corresponding elements of each array.
Example:
<?php
$numbers = [1, 2, 3, 4, 5];
$squared_numbers = array_map(function ($num) {
return $num * $num;
}, $numbers);
print_r($squared_numbers);
?>
Output:
Array
(
[0] => 1
[1] => 4
[2] => 9
[3] => 16
[4] => 25
)
The array_map() function can also be used to process multiple arrays by passing them as additional arguments after the first array. In this case, the callback function should take as many arguments as the number of arrays being passed to array_map(). The function will then be applied to the corresponding elements of each array.
Example:
<?php
$a = [1, 2, 3];
$b = [10, 20, 30];
$c = [100, 200, 300];
$result = array_map(function ($x, $y, $z) {
return $x + $y + $z;
}, $a, $b, $c);
print_r($result);
?>
Output:
Array
(
[0] => 111
[1] => 222
[2] => 333
)
Note that array_map() applies the callback function to the elements in the arrays in a one-to-one manner. If the arrays have different lengths, the function will only be applied to the elements present in the shortest array. The resulting array will have the same length as the shortest input array.