How to Log Errors and Warnings into a File in PHP
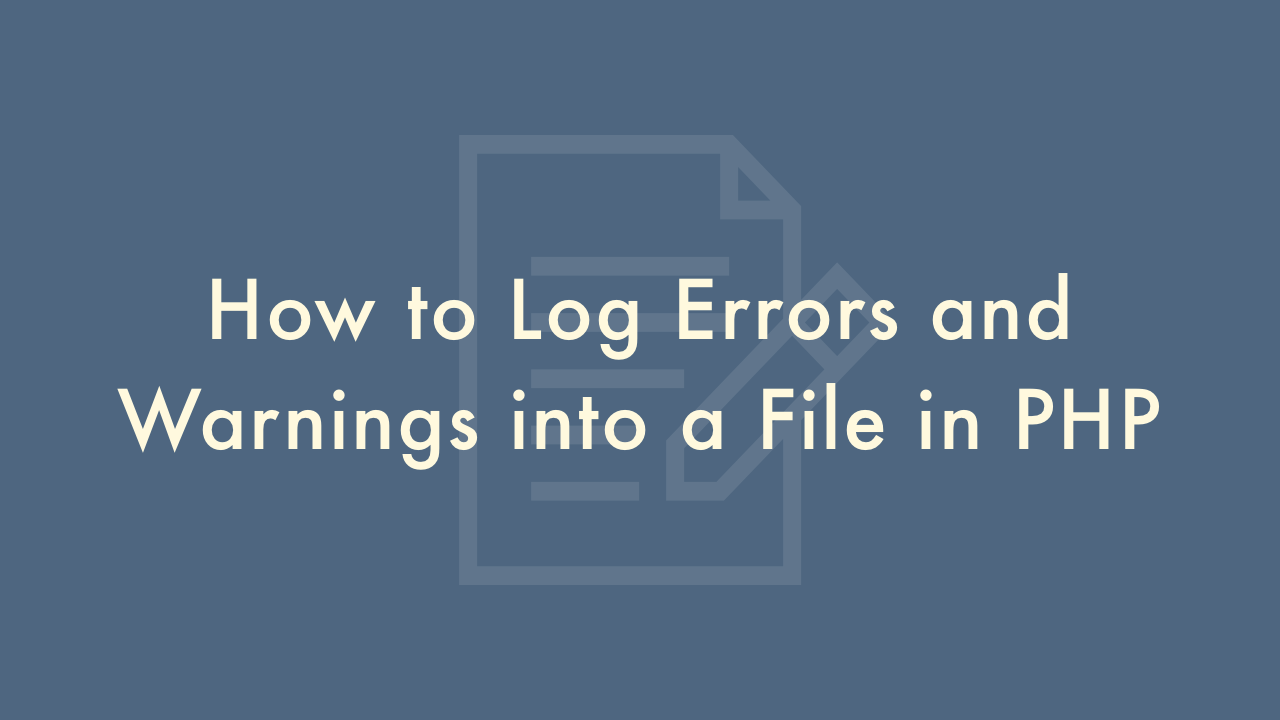
09/05/2021
Contents
In this article, you will learn how to log errors and warnings into a file in PHP.
Logging Errors in PHP
To log errors and warnings in PHP to a file, you can use the following code:
<?php
ini_set("log_errors", 1);
ini_set("error_log", "/path/to/error.log");
?>
This code sets the “log_errors” ini directive to “1”, which indicates that error messages should be logged, and sets the “error_log” directive to the path of the log file.
Logging Warnings in PHP
You can also use the following code to log warnings:
<?php
error_reporting(E_WARNING);
ini_set("display_errors", 0);
?>
This code sets the error reporting level to “E_WARNING” and the “display_errors” directive to “0”, which will turn off display of warnings and log them to the file specified by the “error_log” directive.
Here are some more details:
- The ini_set() function sets a configuration option for the duration of the script. The first parameter is the name of the configuration option, and the second parameter is the value to set it to.
- The error_reporting function sets which errors and warnings should be reported by PHP. The argument is a bitmask indicating which errors and warnings to report. The E_WARNING constant represents warnings.
- The error_log ini directive specifies the path of the log file to which error messages should be written. If this directive is not set, error messages will be written to the “syslog” on Unix systems or to the Windows event log on Windows systems.
- The display_errors ini directive controls whether error messages should be displayed to the user. If this directive is set to “0”, error messages will not be displayed, and will instead be logged to the file specified by the “error_log” directive.
- It’s recommended to set the “display_errors” directive to “0” in a production environment, as error messages can reveal sensitive information to attackers, such as the path of the script or the structure of the database. In a development environment, however, it may be helpful to set “display_errors” to “1” so that you can see error messages as soon as they occur.