How to Set a Cookie in PHP
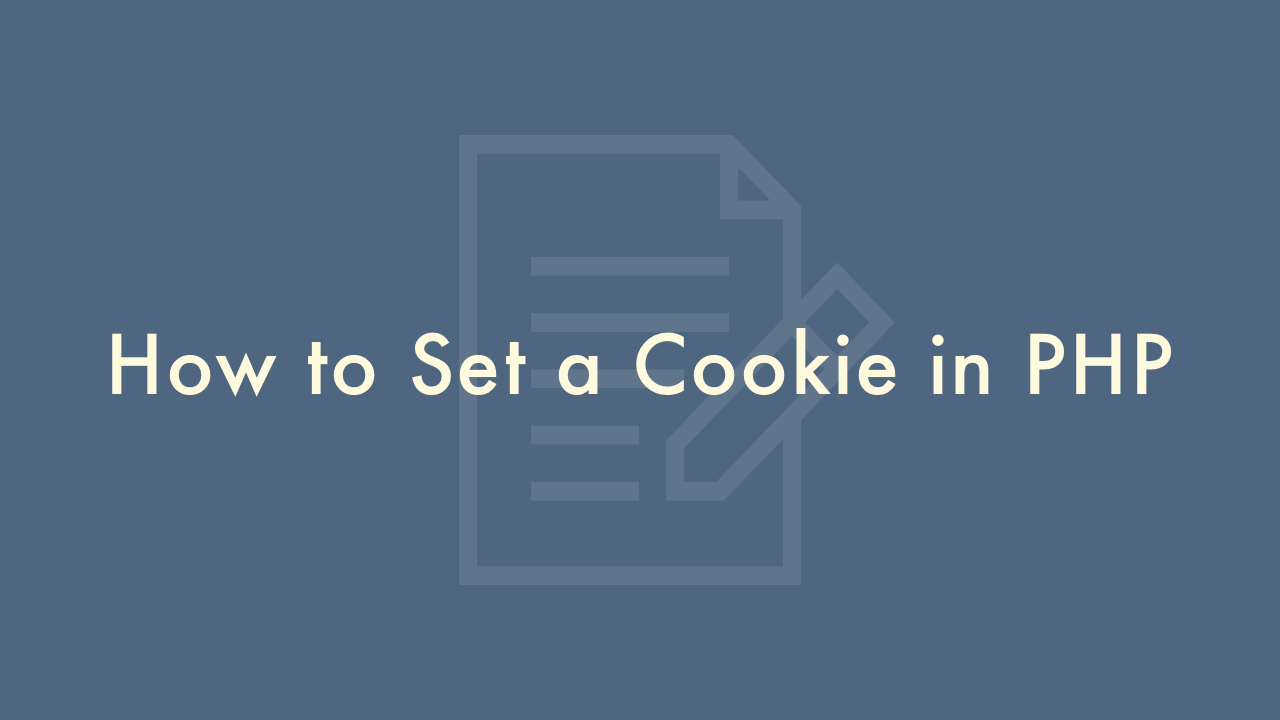
09/05/2021
Contents
In this article, you will learn how to Set a Cookie in PHP.
PHP setcookie() Function
To set a cookie in PHP, you can use the setcookie() function.
Here’s an example:
<?php
setcookie("cookie_name", "cookie_value", time() + (86400 * 30), "/");
?>
In this example, “cookie_name” is the name of the cookie, “cookie_value” is its value, “time() + (86400 * 30)” sets the cookie’s expiration time to 30 days from the current time, and “/” sets the cookie’s scope to the entire domain.
Here’s more information about the parameters of the setcookie function:
- “cookie_name”: This is the name of the cookie and is used to retrieve its value later.
- “cookie_value”: This is the value that will be stored in the cookie. You can store any string or number value in a cookie.
- “time() + (86400 * 30)”: This sets the expiration time of the cookie. The time() function returns the current timestamp, and “86400 * 30” is the number of seconds in 30 days. The cookie will be deleted after 30 days if the user doesn’t visit your website again before then.
- “/”: This sets the path on the server where the cookie will be available. The “/” means that the cookie will be available throughout the entire domain. You can restrict the cookie to a specific folder by changing this value.
After you have set a cookie, you can retrieve its value using the $_COOKIE superglobal array:
<?php
$value = $_COOKIE["cookie_name"];
?>
It is important to note that cookies can only be set before any output has been sent to the browser. This means that you must call the setcookie() function before any HTML or text has been sent.