How to Remove Empty Array Elements in PHP
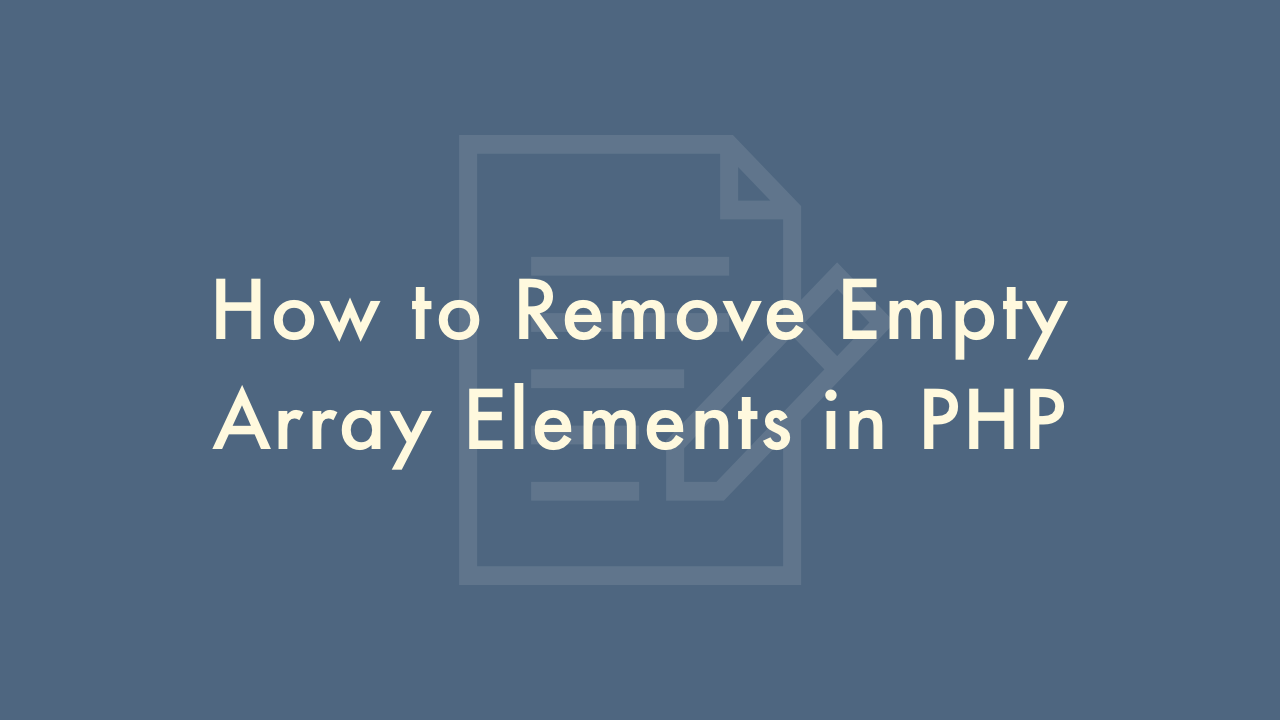
Contents
In this article, you will learn how to remove empty array elements in PHP.
PHP array_filter() Function
You can remove empty array elements in PHP using the array_filter() function.
Syntax:
array_filter(array $array [, callable $callback [, int $flag = 0]]) : array
Parameters:
The function takes an input array $array as its first parameter, which is the array to be filtered.
The second parameter, $callback, is an optional callback function that is used to determine which elements should be included in the filtered array. The function should return true for elements that should be included and false for elements that should be excluded. If no $callback is provided, all elements that evaluate to false will be excluded (i.e. 0, ”, false, null, [], and undefined).
The third parameter, $flag, is also optional and can be one of the following values:
- ARRAY_FILTER_USE_KEY: pass key as the only argument to callback instead of the value.
- ARRAY_FILTER_USE_BOTH: pass both value and key as arguments to callback instead of the value.
Remove empty array elements
Here’s an example:
<?php
$input = array("apple", "banana", "", "cherry");
$output = array_filter($input, 'strlen');
print_r($output);
?>
The above code will output:
Array
(
[0] => apple
[1] => banana
[3] => cherry
)
In this example, the array_filter function is used to filter out all elements in the $input array that have a length of 0, effectively removing all empty elements.
The array_filter function in PHP filters the elements of an array based on a specified condition, which can be provided as a callback function or a flag. In the above example, the callback function strlen is used, which returns the length of a string. The strlen function is passed to array_filter as the second argument.
The array_filter function iterates through each element of the input array, and if the callback function returns true for a given element, the element is included in the filtered array. In this case, the strlen function returns the length of a string. If the length is greater than 0, the element is included in the filtered array, and if the length is 0, the element is excluded.
By using array_filter with the strlen function in this way, you can remove all empty elements (elements with a length of 0) from an array in PHP.
Note that the array_filter function preserves the keys of the input array. If you want to re-index the keys of the filtered array, you can use the array_values function, like this:
<?php
$output = array_values(array_filter($input, 'strlen'));
?>
Remove null values from an array
You can remove all null values from an array in PHP using the array_filter function with a custom callback function or without a callback function.
Here’s an example using a custom callback function:
<?php
$input = array("apple", "banana", null, "cherry");
function remove_null($value) {
return !is_null($value);
}
$output = array_filter($input, 'remove_null');
print_r($output);
?>
The output of the above code will be:
Array
(
[0] => apple
[1] => banana
[3] => cherry
)
In this example, the array_filter function is used to filter out all null values from the $input array. The remove_null callback function is used to determine which elements should be included in the filtered array. The function returns false for null values and true for all other values.
Here’s an example without a callback function:
<?php
$input = array("apple", "banana", null, "cherry");
$output = array_filter($input);
print_r($output);
?>
The output of the above code will be the same as in the previous example:
Array
(
[0] => apple
[1] => banana
[3] => cherry
)
In this case, the array_filter function is used without a callback function. By default, the array_filter function removes all elements that evaluate to false, including null values.