How to Handle Exceptions in PHP
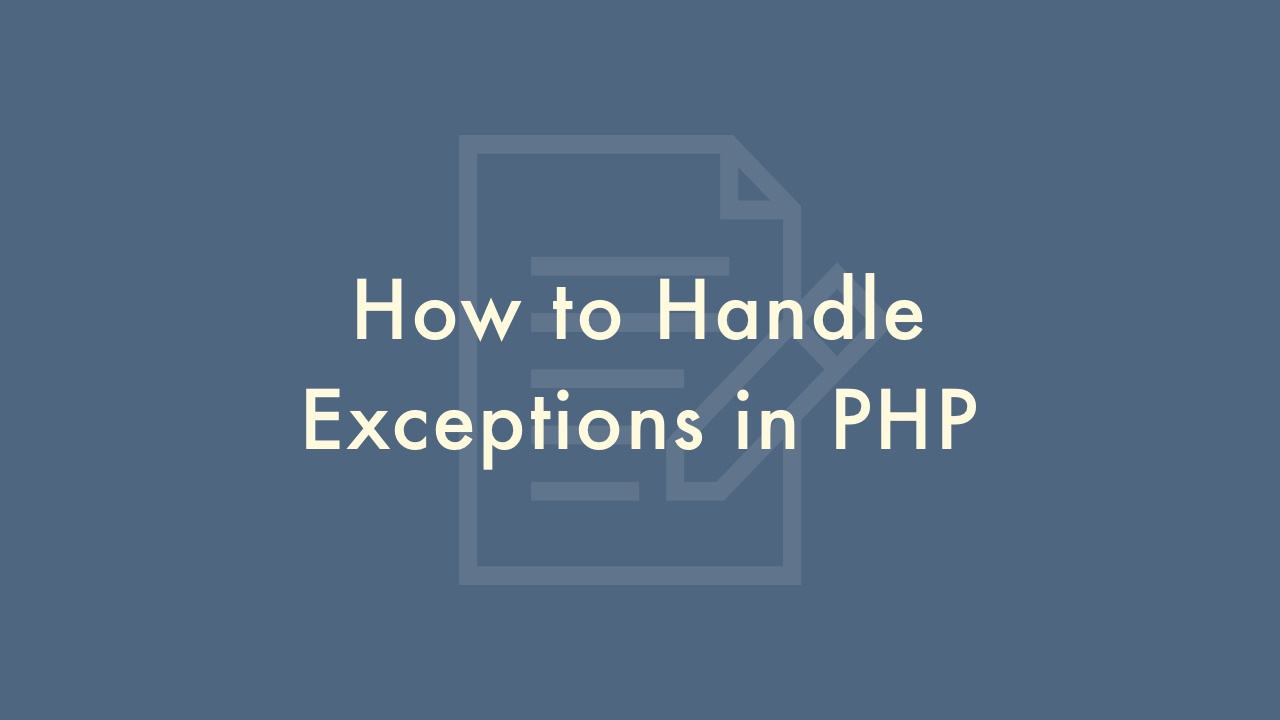
09/05/2021
Contents
In this article, you will learn how to handle exceptions in PHP.
PHP Exception Handling
Exceptions in PHP can be handled using a try-catch block. The try block contains the code that may throw an exception and the catch block contains the code to handle the exception.
Here’s a simple example:
<?php
try {
// code that may throw an exception
if (some_error_condition) {
throw new Exception('Error Message');
}
} catch (Exception $e) {
// code to handle the exception
echo 'Caught exception: ', $e->getMessage(), "\n";
}
?>
In this example, if some_error_condition is true, an exception will be thrown with the message Error Message. The catch block will catch this exception and display its message using the getMessage() method.
There are a few things you should know when working with exceptions in PHP:
- You can throw any object that implements the Throwable interface as an exception. Most commonly, the Exception class is used, but you can also create custom exception classes by extending the Exception class.
- The try block should only contain code that might throw an exception. If an exception is thrown, the rest of the code in the try block will be skipped and the control will immediately transfer to the first catch block that matches the type of the thrown exception.
- If an exception is not caught, it will be propagated up the call stack until it is caught by an outer try-catch block or until it reaches the top-level script, in which case it will terminate the script and display a backtrace.
- You can have multiple catch blocks to handle different types of exceptions. The order of the catch blocks is important because the first matching catch block will be executed.
- You can use the finally block to specify code that will be executed regardless of whether an exception was thrown or not. This can be useful for cleaning up resources, such as closing database connections or releasing file handles.
Here’s an example that demonstrates the use of multiple catch blocks and a finally block:
<?php
try {
// code that may throw an exception
if (some_error_condition) {
throw new Exception('Error Message');
} else if (some_other_error_condition) {
throw new CustomException('Custom Error Message');
}
} catch (CustomException $e) {
// code to handle the custom exception
echo 'Caught custom exception: ', $e->getMessage(), "\n";
} catch (Exception $e) {
// code to handle the general exception
echo 'Caught exception: ', $e->getMessage(), "\n";
} finally {
// code that will be executed regardless of whether an exception was thrown or not
echo "Finally block executed\n";
}
?>