How to Execute a Ping Command in PHP
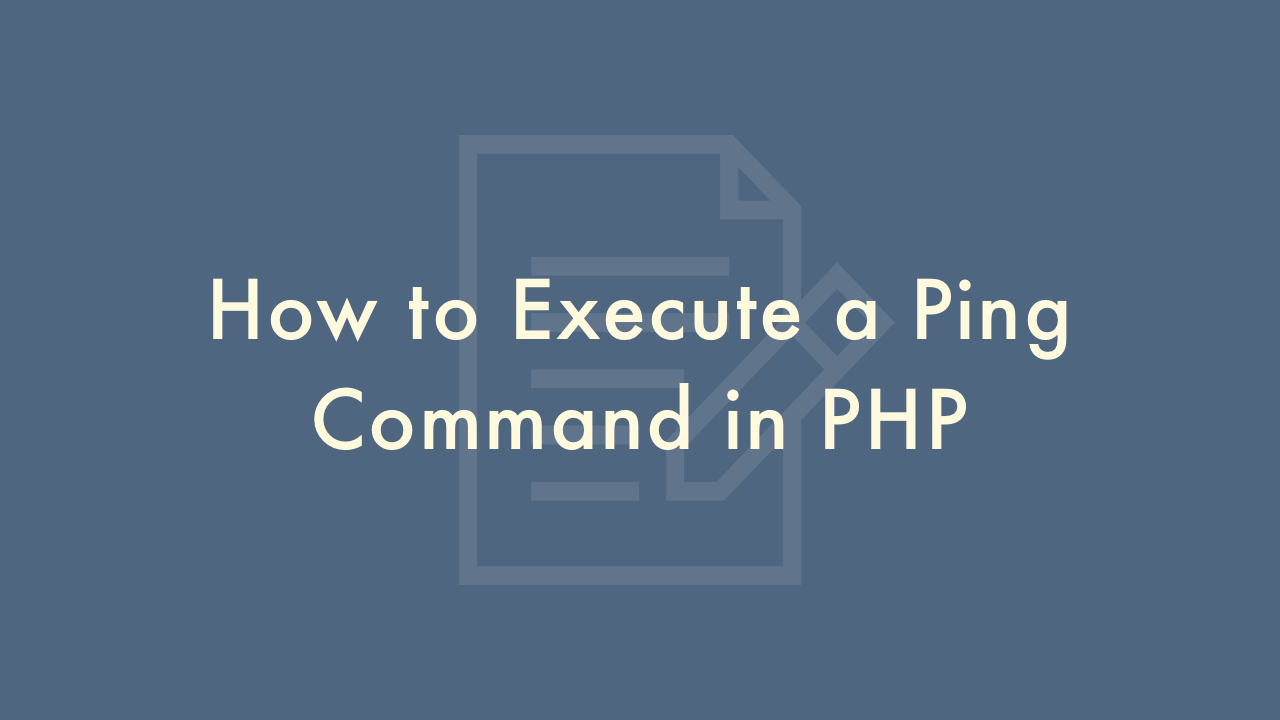
Contents
In this article, you will learn how to execute a ping command in PHP.
PHP exec() Function
You can use the exec() function in PHP to perform a system ping command and retrieve the result.
Here’s an example:
<?php
$host = "www.google.com";
$output = exec("ping -n 1 $host", $result, $status);
if ($status == 0) {
echo "$host is reachable.";
} else {
echo "$host is unreachable.";
}
?>
The exec() function in PHP can be used to run shell commands and return the result as a string. In the example above, the exec() function is used to run the ping command with the hostname or IP address of the target to be pinged. The “-n 1” option is used to specify that only one ping packet should be sent.
The $result variable is an array that contains the output of the command, with each line of the output being an element of the array. The $status variable is an integer that indicates the exit status of the command. A status of 0 indicates success, and a non-zero value indicates failure.
It is important to note that the exec() function should be used with caution, as it can be a security risk if used improperly. For example, if the command being executed is user-specified, it could be possible for an attacker to inject malicious commands that could compromise the system. Always validate and sanitize user input before passing it to the exec() function.