How to Use the PDO::bindValue() Function in PHP
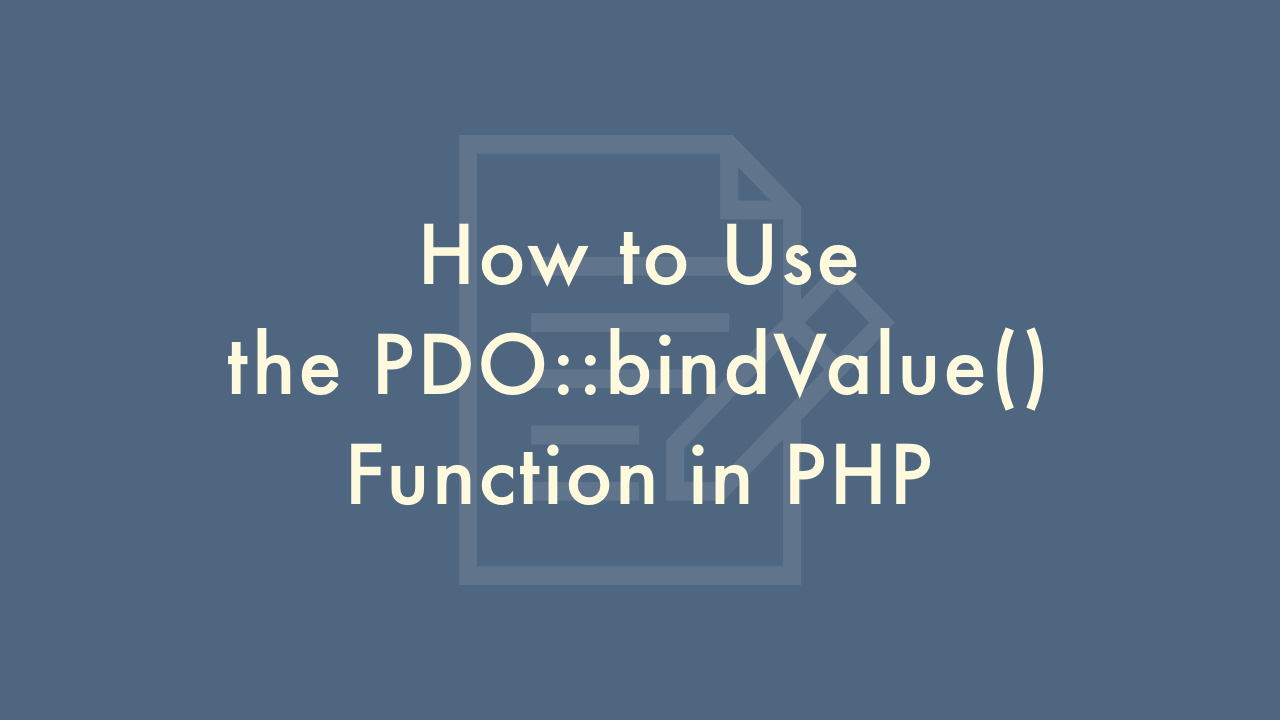
Contents
In this article, you will learn how to use the PDO::bindValue() function in PHP.
PDO::bindValue() Function
The PDO::bindValue() function is used in PHP to bind a value to a named or positional placeholder in a prepared SQL statement.
Syntax
$stmt->bindValue(parameter, value, data_type);
Parameters
$stmt
is a PDO statement object obtained by calling PDO::prepare().parameter
is either a named placeholder (e.g. :name) or a positional placeholder (e.g. ?) in the prepared SQL statement.value
is the value to be bound to the placeholder.data_type
is an optional constant that defines how the value should be treated in the SQL statement. It can be one of the following values:- PDO::PARAM_BOOL: Treat the value as a boolean.
- PDO::PARAM_NULL: Treat the value as a null value.
- PDO::PARAM_INT: Treat the value as an integer.
- PDO::PARAM_STR: Treat the value as a string.
- PDO::PARAM_LOB: Treat the value as a large object (BLOB).
- PDO::PARAM_STMT: Treat the value as a record set (result set).
Example
Here’s an example of how to use the bindValue() function:
<?php
$db = new PDO("mysql:host=localhost;dbname=testdb", "username", "password");
$query = "SELECT * FROM users WHERE username = :username";
$stmt = $db->prepare($query);
$stmt->bindValue(":username", "john_doe", PDO::PARAM_STR);
$stmt->execute();
$result = $stmt->fetchAll();
?>
In this example, the placeholder :username is bound to the value “john_doe” and the data type PDO::PARAM_STR is specified to indicate that the value is a string.
Here’s an example that demonstrates the use of named placeholders and the PDO::PARAM_INT data type:
<?php
$db = new PDO("mysql:host=localhost;dbname=testdb", "username", "password");
$query = "SELECT * FROM users WHERE id = :id";
$stmt = $db->prepare($query);
$stmt->bindValue(":id", 10, PDO::PARAM_INT);
$stmt->execute();
$result = $stmt->fetchAll();
?>
In this example, the named placeholder :id is bound to the value 10 and the data type PDO::PARAM_INT is specified to indicate that the value is an integer.