How to Use the PHP mktime() Function
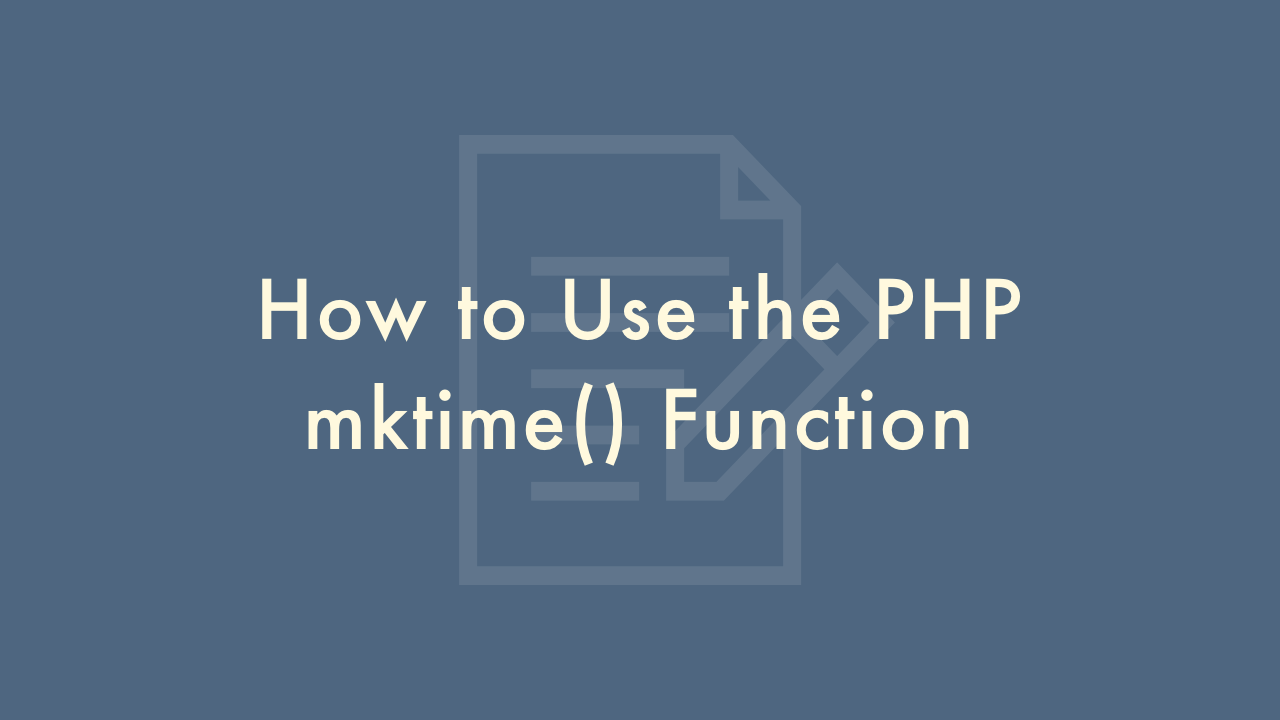
Contents
In this article, you will learn how to use the PHP mktime() function.
PHP mktime() Function
The mktime() function in PHP is used to create a timestamp based on the parameters passed to it.
Syntax:
mktime($hour, $minute, $second, $month, $day, $year)
Parameters:
- $hour: The hour of the time.
- $minute: The minute of the time.
- $second: The second of the time.
- $month: The month of the year (from 0 to 11).
- $day: The day of the month (from 1 to 31).
- $year: The year (4 digits).
Example:
For example, to create a timestamp for January 1st, 2022 at 12:00:00 PM:
<?php
$timestamp = mktime(12, 0, 0, 0, 1, 2022);
?>
Here are some additional details and examples to help you better understand the mktime() function in PHP:
- If you pass -1 for the hour, minute, second, month, day, or year argument, the current local time value will be used for that argument.
- The mktime() function returns the number of seconds elapsed since January 1, 1970, 00:00:00 UTC.
- The returned timestamp can be used in various date and time related functions such as date(), strftime(), getdate(), and date_create().
Here is an example to demonstrate how to use the mktime() function to create a timestamp, and then format it using the date() function:
<?php
$timestamp = mktime(12, 0, 0, 0, 1, 2022);
$formatted_date = date("l, F d, Y h:i:s A", $timestamp);
echo $formatted_date;
?>
Output:
Sunday, January 01, 2022 12:00:00 PM
In this example, we first created a timestamp using mktime() and stored it in the $timestamp variable. We then used the date() function to format the timestamp into a human-readable string, which we stored in the $formatted_date variable. Finally, we echoed the formatted date.