How to Read RSS Feeds in PHP
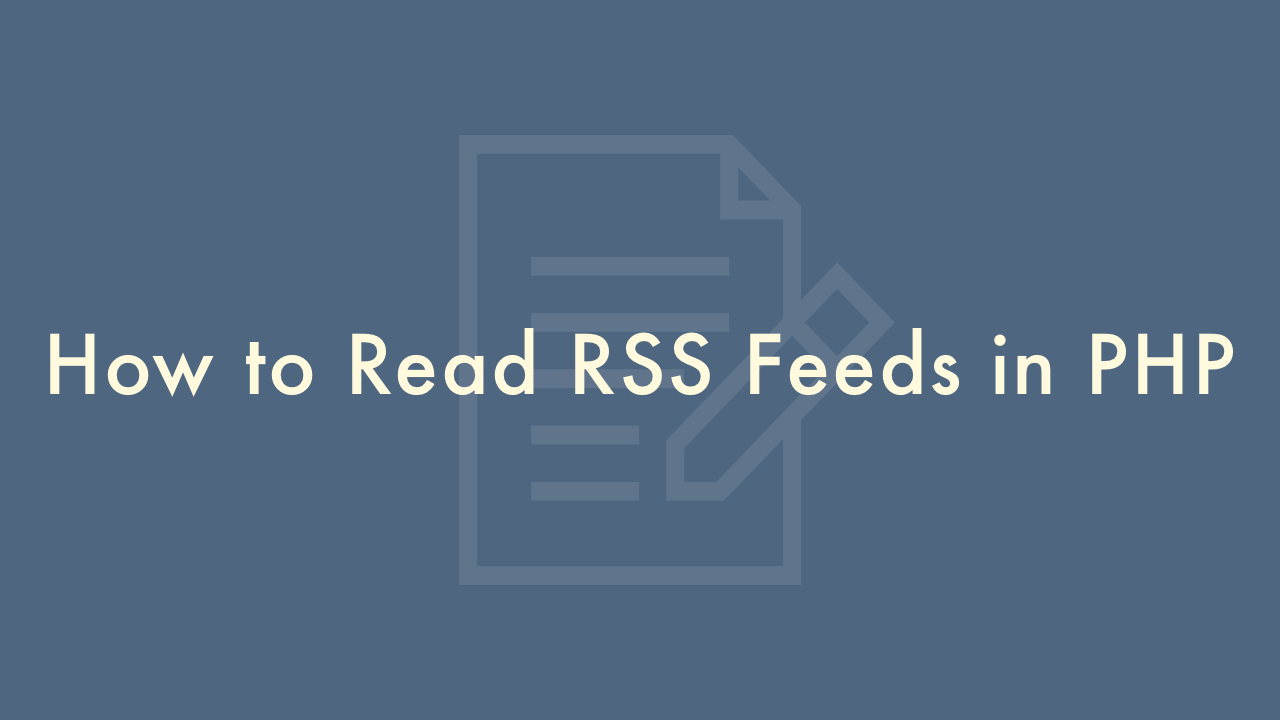
Contents
In this article, you will learn how to read RSS feeds in PHP.
Read RSS Feed
To read RSS feeds in PHP, you can use the SimpleXML extension to parse the RSS feed XML data into a PHP object, which you can then access and manipulate as needed.
Here’s a simple example to get you started:
<?php
// Load the RSS feed into a SimpleXML object
$rss = simplexml_load_file('http://example.com/feed.xml');
// Loop through each item in the feed
foreach ($rss->channel->item as $item) {
// Access the title, link, and description of the item
$title = (string) $item->title;
$link = (string) $item->link;
$description = (string) $item->description;
// Output the title and description of the item
echo "<h2><a href='$link'>$title</a></h2>";
echo "<p>$description</p>";
}
?>
This code uses the simplexml_load_file() function to load the RSS feed from the specified URL, and then loops through each item element in the channel element of the feed, accessing its title, link, and description elements, and outputting them to the page.
Here’s some more information about the example code:
- The simplexml_load_file() is a function that parses an XML file and returns a SimpleXML object that represents the data. This function takes the URL of the RSS feed as its argument, and returns a SimpleXML object that you can use to access and manipulate the data in the feed.
- In the loop, we use the foreach statement to iterate over each item element in the channel element of the feed. The item element represents a single item in the feed, and usually contains information such as the title, link, and description of the item.
- Within the loop, we access the title, link, and description of the item by casting each element to a string. We then use these values to output the title and description of the item, with the title wrapped in an HTML heading tag and linked to the item’s URL.
This is just a basic example, but it should give you a good starting point for reading and processing RSS feeds in PHP. You can use the SimpleXML object to access and manipulate any other data in the feed that you’re interested in.