How to Read a File Line by Line in PHP
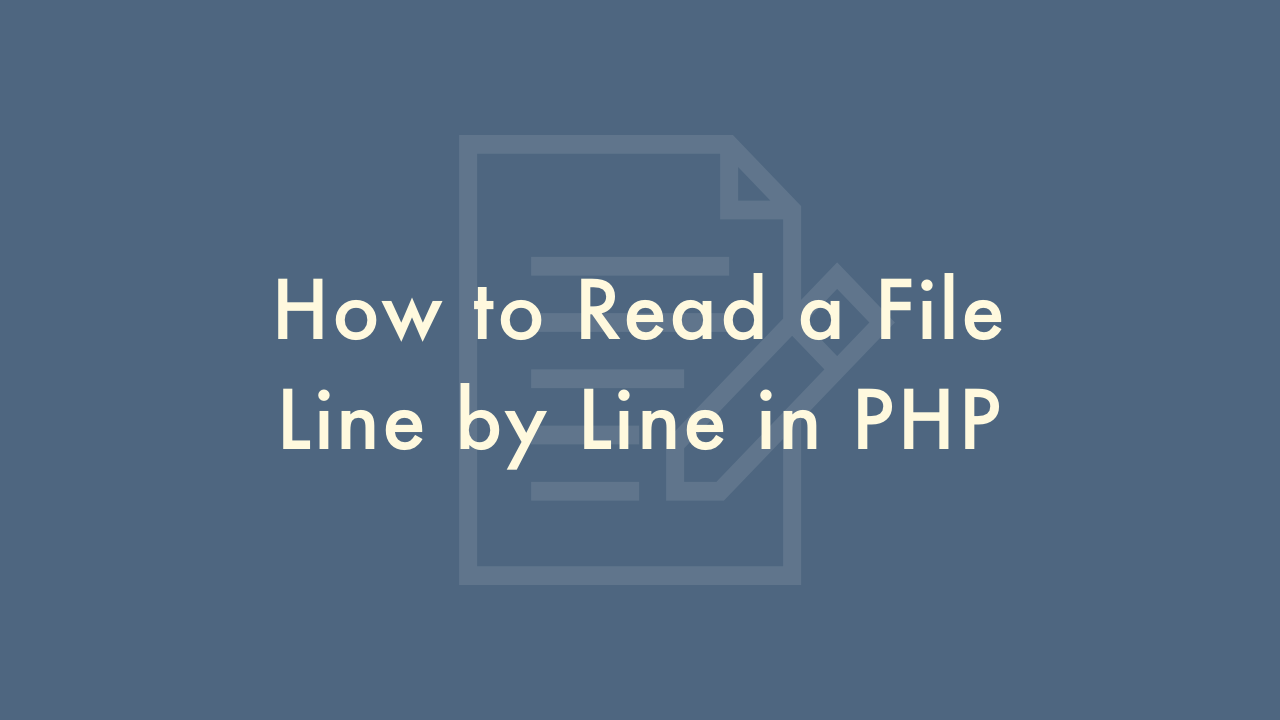
09/05/2021
Contents
In this article, you will learn how to read a file line by line in PHP.
PHP fgets() Function
You can use the fgets() function to read a file line by line in PHP.
Here’s an example:
<?php
$file = fopen("example.txt", "r");
while(!feof($file)) {
$line = fgets($file);
echo $line;
}
fclose($file);
?>
Here’s a more detailed explanation of this example:
- The first line of the code opens the file “example.txt” in read mode using the fopen() function. The file pointer is assigned to the variable $file.
- The while loop starts, and the feof() function is used to check whether the end of the file has been reached. If the end of the file has not been reached, the loop will continue to execute.
- Inside the while loop, the fgets() function reads the next line of the file, and assigns it to the variable $line.
- The current line is then printed to the screen using the echo command.
- The while loop continues to execute until the end of the file is reached. Once the end of the file is reached, the loop will exit, and the program will continue to the next line of code.
- The last line of the code uses the fclose() function to close the file. This is important because it releases the resources used by the file and allows other operations to be performed on the file.
It’s worth noting that the fgets() function has an optional parameter that allows you to specify the maximum number of characters to be read from the file on each iteration. For example, fgets($file, 1024) will read a maximum of 1024 characters per line. Additionally, you can use the file() function to read the entire file into an array, with one element per line.