How to Extract a Substring from a String in PHP
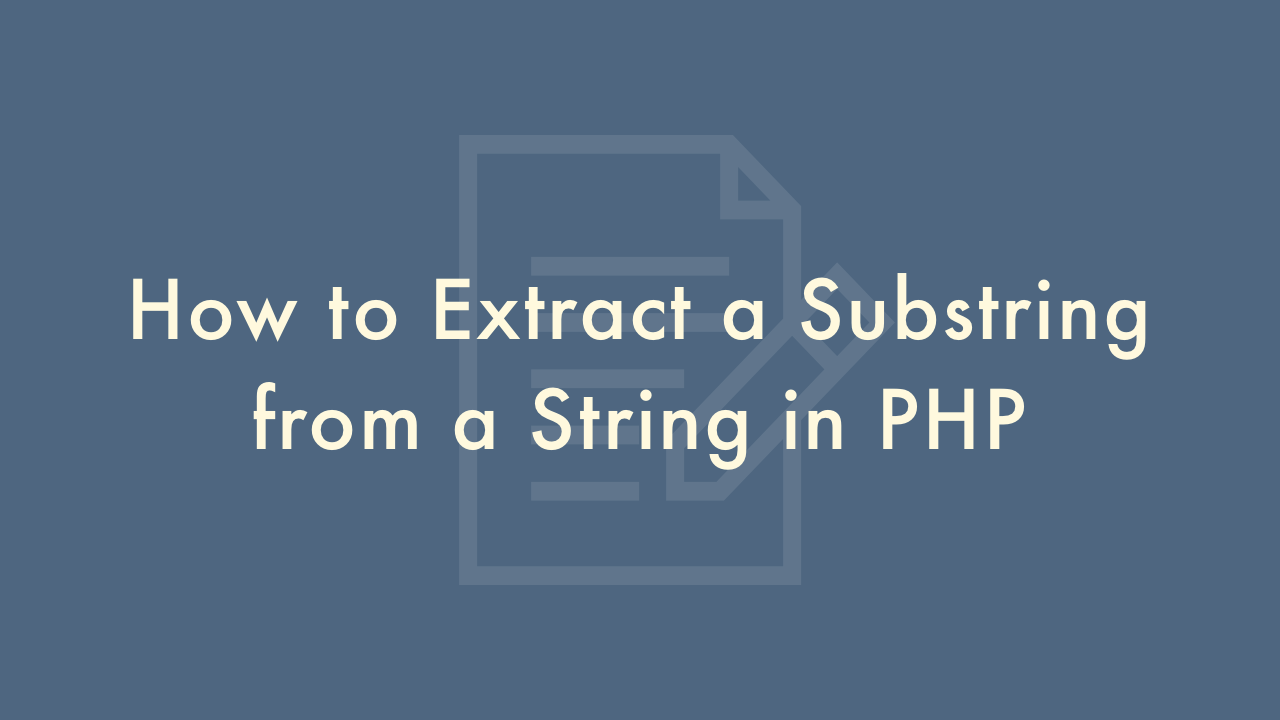
09/06/2021
Contents
In this article, you will learn how to get a substring from a string in PHP.
PHP substr() Function
In PHP, you can extract a substring from a string using the substr() function.
The substr() is a built-in function in PHP that is used to extract a portion of a string.
Syntax
The syntax for the substr() function is as follows:
substr(string $string, int $start [, int $length ]);
Parameters
The substr() function takes three arguments:
$string
: The string from which you want to extract the substring.$start
: The starting position of the substring. If $start is positive, the substring will start from the $start-th character counting from the left. If $start is negative, the substring will start from the $start-th character counting from the right.$length
(optional): The length of the substring you want to extract. If $length is not specified, the function will extract the substring from $start to the end of the string. If $length is negative, the function will extract the substring up to that many characters from the end of the string.
Example
Here are some examples of using the substr() function:
<?php
$string = "Hello, World!";
// Extract the substring starting from the 7th character and of length 5
$substring = substr($string, 7, 5);
// Output: "World"
echo $substring;
// Extract the substring starting from the 7th character to the end of the string
$substring = substr($string, 7);
// Output: "World!"
echo $substring;
// Extract the substring of length 5 counting from the end of the string
$substring = substr($string, -5, 5);
// Output: "World"
echo $substring;
?>