How to Validate an Email Address in PHP
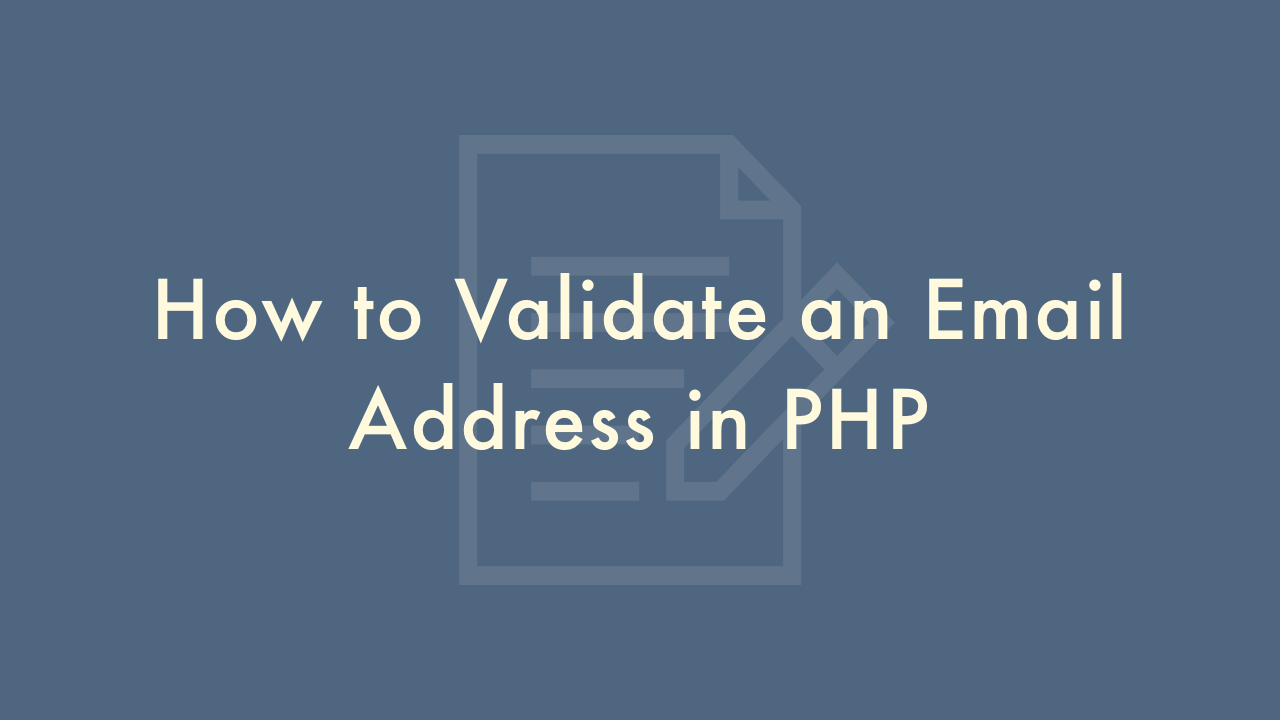
Contents
In this article, you will learn how to validate an email address in PHP.
Using FILTER_VALIDATE_EMAIL
The filter_var() function in PHP can be used to validate an email address using the FILTER_VALIDATE_EMAIL filter. This function returns true if the email address is valid and false if it’s not.
Here’s an example of how to use filter_var to validate an email address:
<?php
$email = 'example@example.com';
if (filter_var($email, FILTER_VALIDATE_EMAIL)) {
echo 'Valid email address';
} else {
echo 'Invalid email address';
}
?>
The filter_var() function is considered to be more reliable and accurate than using a regular expression for email validation, as it checks the email address against a variety of criteria, including the format of the email address, the existence of the domain, and more.
Keep in mind that filter_var will return false for some valid email addresses that use newer TLDs (top-level domains) that are not yet recognized by PHP. In those cases, you may need to update the list of recognized TLDs in your PHP installation.
Using preg_match() and Regex
In PHP, you can validate an email address using a regular expression pattern and the preg_match() function.
Here is an example function to validate an email address:
<?php
function isValidEmail($email) {
$pattern = '/^([a-z0-9\+_\-]+)(\.[a-z0-9\+_\-]+)*@([a-z0-9\-]+\.)+[a-z]{2,6}$/ix';
return preg_match($pattern, $email);
}
?>
You can then use the function like this:
<?php
$email = 'example@example.com';
if (isValidEmail($email)) {
echo 'Valid email address';
} else {
echo 'Invalid email address';
}
?>
A regular expression is a pattern that can be used to match strings. The pattern used in the above example is a common one for matching valid email addresses. It checks for the presence of a few key elements in the email address, such as an @ symbol, a domain name with a valid TLD (top-level domain), and text before and after the @ symbol.
The preg_match() function is used to match a regular expression pattern against a string. In this case, it’s used to match the email address against the pattern for a valid email address. If the function returns 1, it means the email address is valid. If it returns 0, it means the email address is not valid.