How to Use the PHP date() Function
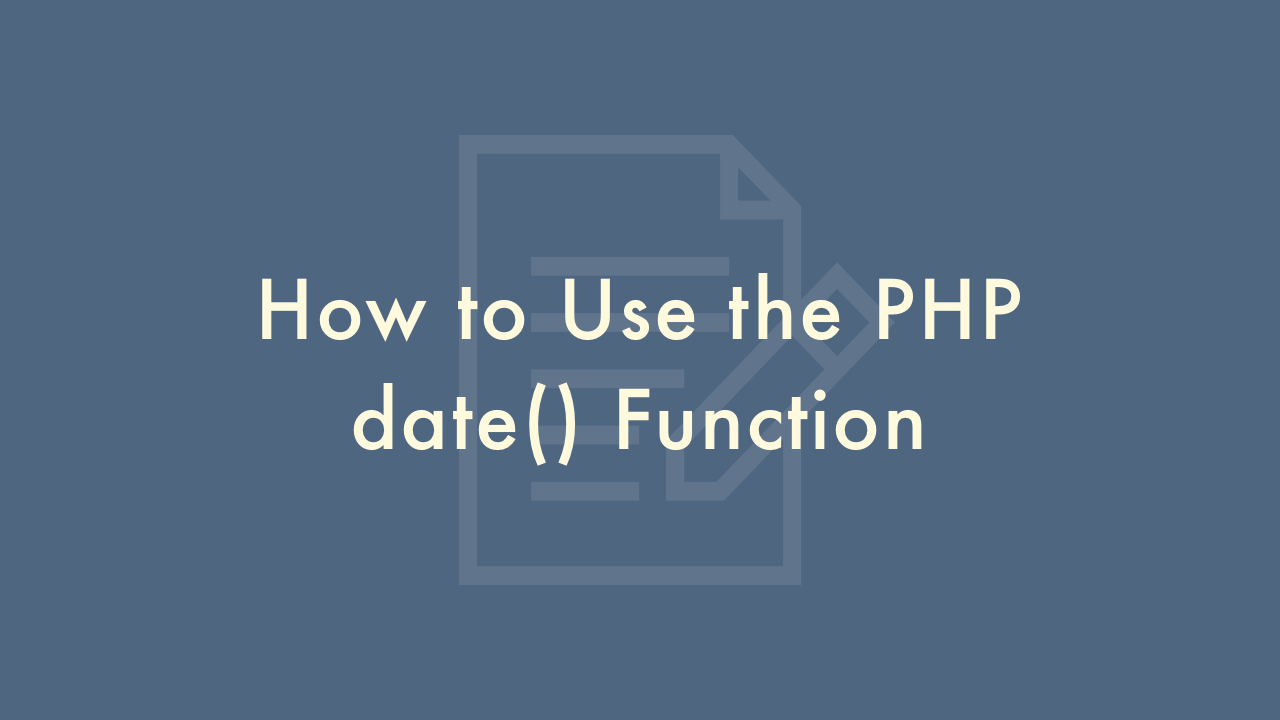
Contents
In this article, you will learn how to use the PHP date() function.
PHP date() Function
The date() function in PHP is used to format a date and time.
Syntax:
date(format, timestamp)
Parameters:
format
is a string that specifies the format of the outputted date.timestamp
is an optional integer that represents the number of seconds since January 1, 1970 (the Unix timestamp). If no timestamp is provided, the current date and time is used.
Here are some commonly used format characters:
- d – day of the month (01 to 31)
- m – month (01 to 12)
- Y – year (example: 2021)
- H – hour (00 to 23)
- i – minutes (00 to 59)
- s – seconds (00 to 59)
Example:
<?php
echo date("Y-m-d H:i:s");
?>
This would output the current date and time in the format 2021-06-17 09:00:00.
The date() function in PHP is a powerful tool for formatting dates and times. You can use it to display the date and time in a variety of formats.
Here are some additional format characters:
- l (lowercase ‘L’) – day of the week (Sunday to Saturday)
- F – full textual representation of the month (January to December)
- j – day of the month without leading zeros (1 to 31)
- n – numeric representation of a month, without leading zeros (1 to 12)
- a – lowercase ante/post meridiem (am/pm)
- A – uppercase ante/post meridiem (AM/PM)
You can also use the date() function with a Unix timestamp to format a date and time in the past or future. The Unix timestamp is the number of seconds that have elapsed since January 1, 1970, so you can use it to calculate dates and times.
Example:
<?php
echo date("l, F j, Y", strtotime("2021-06-17"));
?>
This would output Thursday, June 17, 2021.
In addition to formatting dates and times, the date() function can also be used to perform arithmetic on dates and times. For example, you can add or subtract days, weeks, months, or years from a date using the strtotime() function.
Example:
<?php
$nextWeek = strtotime("+1 week");
echo date("l, F j, Y", $nextWeek);
?>
This would output the date one week from today.