The Difference between round(), ceil(), floor() and intval() in PHP
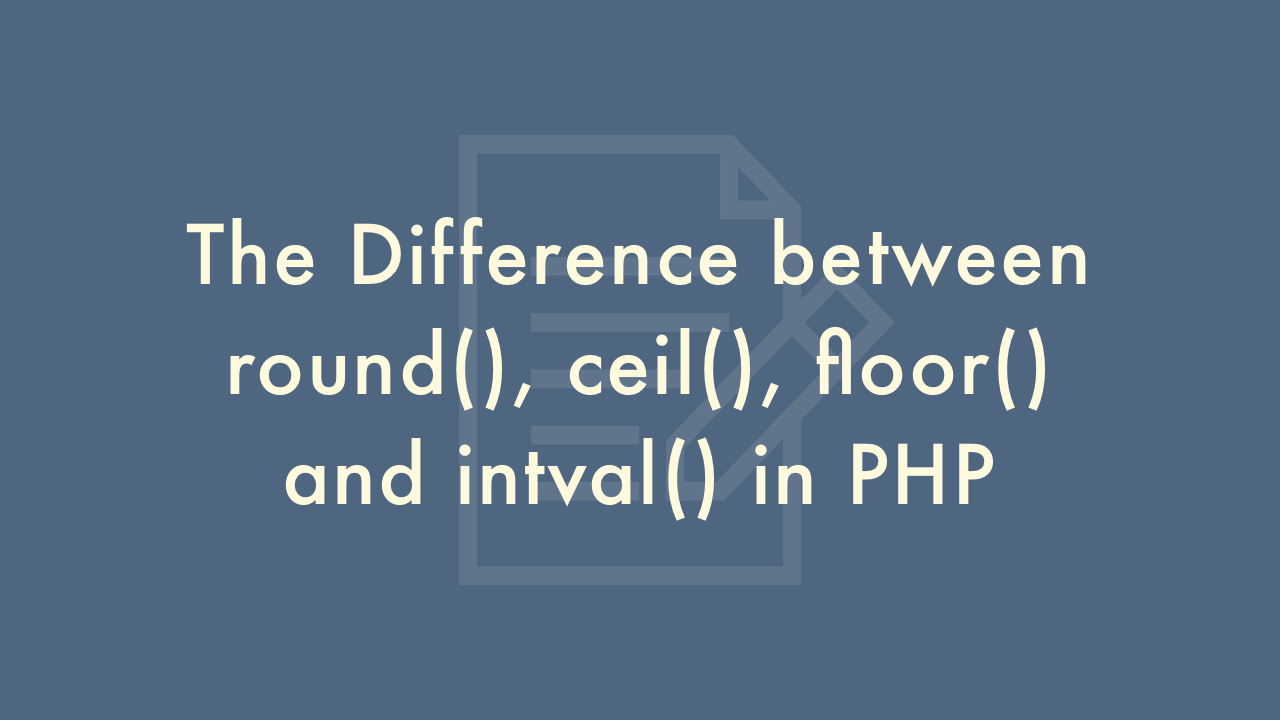
Contents
In this article, you will learn about the difference between round(), ceil(), floor() and intval() in PHP.
PHP round() Function
The round() function in PHP is used to round a floating-point number to the nearest integer or to a specified number of decimal places.
Syntax:
round(float $val, int $precision = 0, int $mode = PHP_ROUND_HALF_UP)
Parameters:
$val
: the number to be rounded.
$precision
: (optional) the number of decimal places to round to.
$mode
: (optional) how to round the number. Can be one of the following constants:
- PHP_ROUND_HALF_UP: rounds up if the fraction is greater than or equal to 0.5
- PHP_ROUND_HALF_DOWN: rounds down if the fraction is less than 0.5
- PHP_ROUND_HALF_EVEN: rounds up or down depending on the nearest even number
- PHP_ROUND_HALF_ODD: rounds up or down depending on the nearest odd number
Examples:
<?php
$num = 2.5;
echo round($num); // 3
$num = 2.4;
echo round($num); // 2
$num = 2.6;
echo round($num, 1, PHP_ROUND_HALF_DOWN); // 2.5
?>
PHP ceil() Function
The ceil() function in PHP rounds a floating-point number up to the next highest integer. It rounds a number up even if it is already a whole number.
Syntax:
ceil(float $value)
$value
: the number to be rounded up.
Examples:
<?php
$num = 2.1;
echo ceil($num); // 3
$num = 2.9;
echo ceil($num); // 3
$num = -2.1;
echo ceil($num); // -2
?>
PHP floor() Function
The floor() function in PHP rounds a floating-point number down to the next lowest integer. It rounds a number down even if it is already a whole number.
Syntax:
floor(float $value)
$value
: the number to be rounded down.
Examples:
<?php
$num = 2.1;
echo floor($num); // 2
$num = 2.9;
echo floor($num); // 2
$num = -2.1;
echo floor($num); // -3
?>
PHP intval() Function
The intval() function in PHP is used to convert a value to an integer. If the value cannot be converted to an integer, it will return 0.
Syntax:
intval(mixed $value, int $base = 10)
$value
: the value to be converted to an integer.
$base
: (optional) the base of the number system to use. If the number is a string, $base
must be between 2 and 36, inclusive.
Examples:
<?php
$num = 2.5;
echo intval($num); // 2
$num = '12345';
echo intval($num, 8); // 5349
$num = '1010';
echo intval($num, 2); // 10
?>