How to Get a File Extension in PHP
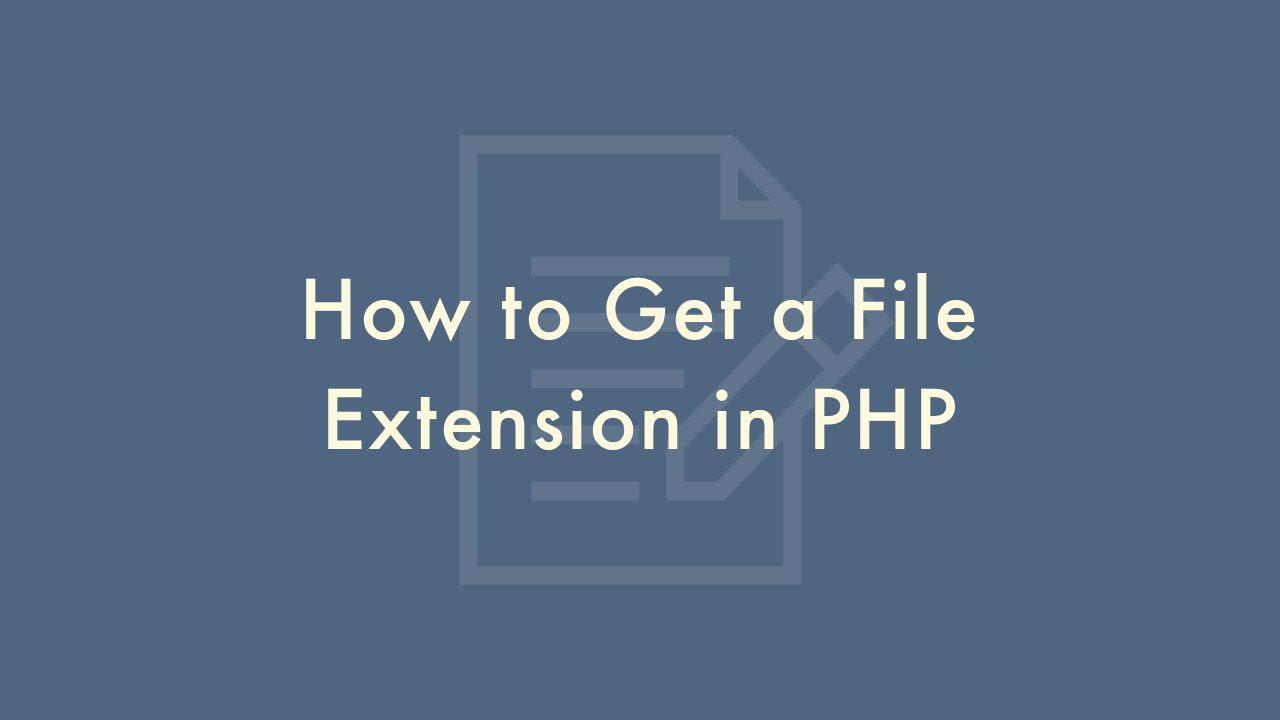
09/05/2021
Contents
In this article, you will learn how to get a file extension in PHP.
PHP pathinfo() Function
In PHP, you can get the file extension of a given file path using the pathinfo() function.
Here’s an example:
<?php
$path = "/path/to/file.txt";
$file_extension = pathinfo($path, PATHINFO_EXTENSION);
?>
The second argument to the pathinfo() function, PATHINFO_EXTENSION, specifies that you want to retrieve the file extension only.
The pathinfo() function in PHP returns an associative array containing information about a file path, including the directory name, base name, and extension.
Here’s an example of how you can use pathinfo() to retrieve all information about a file:
<?php
$path = "/path/to/file.txt";
$file_info = pathinfo($path);
echo "Directory name: " . $file_info['dirname'] . "\n";
echo "Base name: " . $file_info['basename'] . "\n";
echo "Extension: " . $file_info['extension'] . "\n";
?>
The pathinfo() function returns an associative array with the following keys:
- dirname: The directory name of the file
- basename: The base name of the file (i.e., the file name without the extension)
- extension: The file extension (if any)
- filename: The file name without the extension (added in PHP 5.2.0)
Keep in mind that pathinfo() only works with file paths and not actual file contents.