How to Use AJAX in PHP
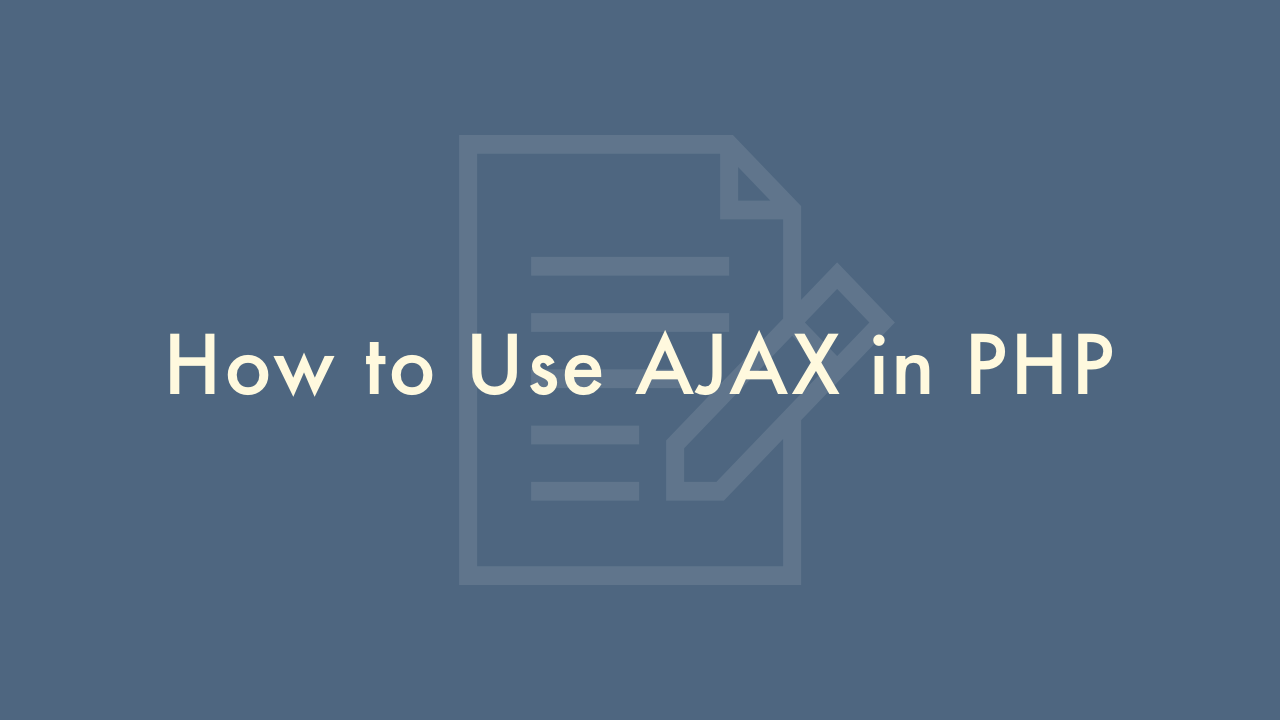
Contents
In this article, you will learn how to use AJAX in PHP.
How to use AJAX in PHP and JavaScript
AJAX (Asynchronous JavaScript and XML) can be used with PHP to dynamically update a web page without reloading the entire page.
Here’s how to use AJAX with PHP and JavaScript:
- Create an HTML form with inputs for the user.
- Attach an event handler to the form submit event to trigger an AJAX request.
- Use JavaScript to send an AJAX request to a PHP script on the server.
- In the PHP script, perform the necessary processing (e.g., fetch data from a database) and return a response.
- Use JavaScript to process the response from the PHP script and update the contents of the web page.
Here is an example of the JavaScript code to send an AJAX request:
var xhr = new XMLHttpRequest();
xhr.open("POST", "process.php", true);
xhr.setRequestHeader("Content-Type", "application/x-www-form-urlencoded");
xhr.onreadystatechange = function() {
if (this.readyState === XMLHttpRequest.DONE && this.status === 200) {
// process the response from the PHP script
}
};
xhr.send("name=" + encodeURIComponent(name) + "&email=" + encodeURIComponent(email));
And here is an example of the PHP code to process the AJAX request:
<?php
if ($_SERVER["REQUEST_METHOD"] === "POST") {
$name = $_POST["name"];
$email = $_POST["email"];
// perform the necessary processing
echo json_encode(["message" => "Hello, $name"]);
}
?>
How to use AJAX in PHP and jQuery
jQuery is a popular JavaScript library that makes it easier to perform AJAX requests and manipulate the Document Object Model (DOM).
Here’s how to use AJAX in PHP and jQuery:
- Create an HTML form with inputs for the user.
- Attach an event handler to the form submit event using jQuery.
- Use the jQuery $.ajax method to send an AJAX request to a PHP script on the server.
- In the PHP script, perform the necessary processing (e.g., fetch data from a database) and return a response.
- Use jQuery to process the response from the PHP script and update the contents of the web page.
Here is an example of the jQuery code to send an AJAX request:
$("#form").submit(function(event) {
event.preventDefault();
var name = $("input[name='name']").val();
var email = $("input[name='email']").val();
$.ajax({
type: "POST",
url: "process.php",
data: { name: name, email: email },
dataType: "json",
success: function(response) {
// process the response from the PHP script
$("#message").text(response.message);
}
});
});
And here is an example of the PHP code to process the AJAX request:
<?php
if ($_SERVER["REQUEST_METHOD"] === "POST") {
$name = $_POST["name"];
$email = $_POST["email"];
// perform the necessary processing
echo json_encode(["message" => "Hello, $name"]);
}
?>
In this example, the form with an ID of “form” is submitted using the jQuery submit method. The preventDefault method is used to prevent the form from being submitted in the traditional manner. Instead, an AJAX request is sent to the PHP script using the jQuery $.ajax method. The PHP script processes the request and returns a JSON response, which is processed by the success function and used to update the contents of a div element with an ID of “message”.
Using jQuery makes it easier to perform AJAX requests and manipulate the DOM, as it provides a simpler syntax and supports a wide range of features. By combining jQuery with PHP, you can create dynamic, interactive web applications that provide a better user experience.