How to Get Selected Option Value in PHP
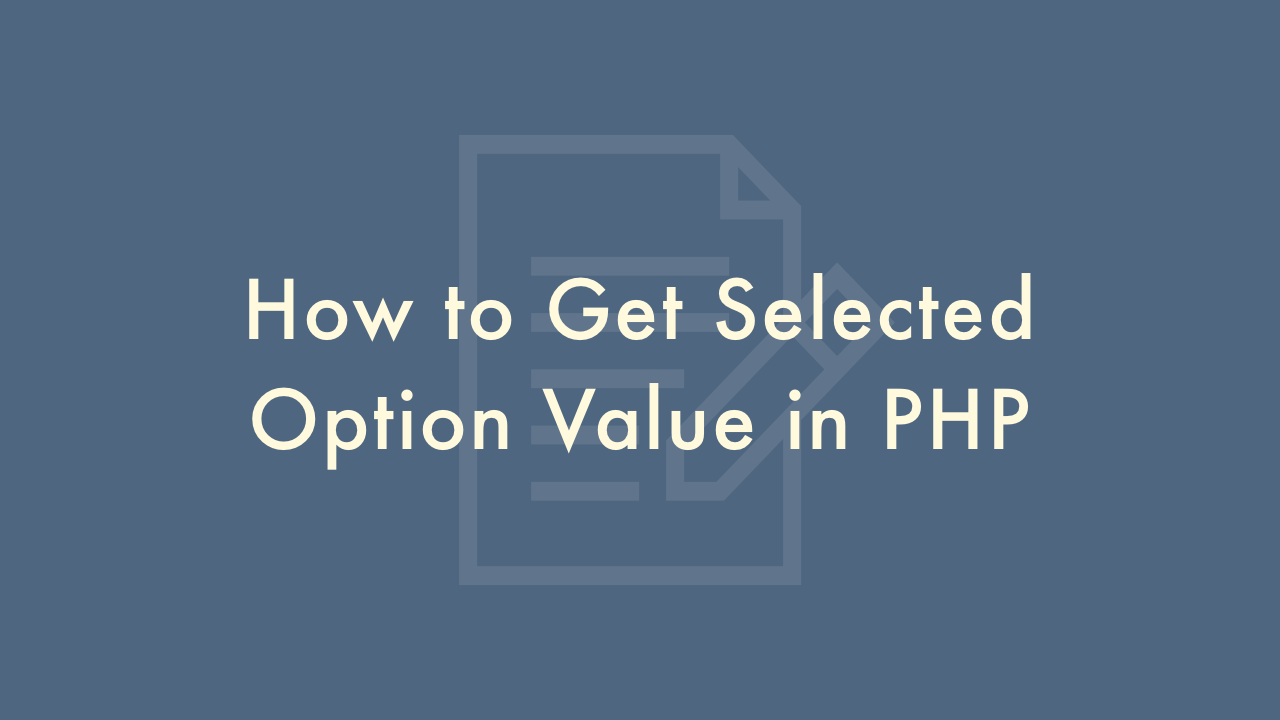
Contents
In this article, you will learn how to get selected option value in PHP .
Using the $_POST or $_GET superglobal array
To get the selected value of a dropdown list in PHP, you need to use the $_POST or $_GET superglobal array to get the value of the selected option when the form is submitted.
Here’s an example:
<form action="your-php-script.php" method="post">
<select name="color">
<option value="red">Red</option>
<option value="green">Green</option>
<option value="blue">Blue</option>
</select>
<input type="submit" value="Submit">
</form>
And in your-php-script.php:
<?php
if (isset($_POST['color'])) {
$selected_color = $_POST['color'];
echo "You selected: " . $selected_color;
}
?>
You can also use the $_GET superglobal array in the same way if you change the form’s method attribute to “get”.
In the example code, the dropdown list is created with a
When the form is submitted, the value of the selected option is sent to the server as part of the form data. In PHP, you can access this value using the $_POST or $_GET array, depending on the method used in the form. In this example, the form uses the POST method, so the selected value is accessed using $_POST[‘color’].
It’s important to check if the value has been set before using it. This can be done with the isset() function, which returns true if the specified variable is set and not null. If the value has been set, you can then use it as needed, such as storing it in a variable or using it in a database query.
In this example, the selected value is stored in a variable $selected_color and then displayed using echo.