How to Get the Length of an Array in PHP
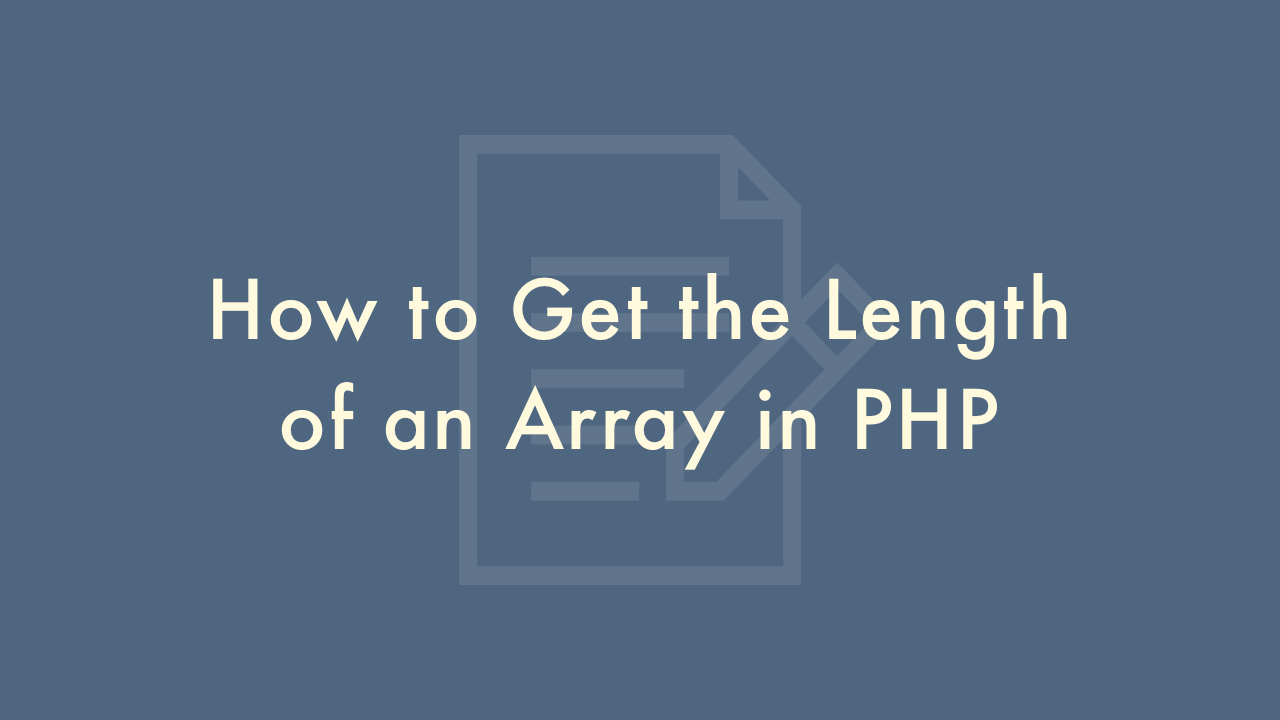
Contents
In this article, you will learn how to get the length of an array in PHP.
Using the count() function
In PHP, the length or size of an array can be obtained using the count() function. The function takes the array as an argument and returns the number of elements in the array.
Here’s an example of using the count() function:
<?php
$fruits = array("apple", "banana", "cherry", "orange");
$num_fruits = count($fruits);
// $num_fruits will be 4
?>
You can also use the count() function to determine the number of elements in an object that implements the Countable interface.
Here’s an example of using the count function on an object:
<?php
class FruitBasket implements Countable {
private $fruits = array("apple", "banana", "cherry", "orange");
public function count()
{
return count($this->fruits);
}
}
$basket = new FruitBasket();
$num_fruits = count($basket);
// $num_fruits will be 4
?>
Using the sizeof() function
The sizeof() function in PHP is an alias for the count() function, so it can be used in the same way as the count() function. It determines the number of elements in an array or the number of elements in an object that implements the Countable interface.
Here’s an example of using the sizeof() function with an array:
<?php
$fruits = array("apple", "banana", "cherry", "orange");
$num_fruits = sizeof($fruits);
// $num_fruits will be 4
?>
And here’s an example of using the sizeof() function with an object:
<?php
class FruitBasket implements Countable {
private $fruits = array("apple", "banana", "cherry", "orange");
public function count()
{
return count($this->fruits);
}
}
$basket = new FruitBasket();
$num_fruits = sizeof($basket);
// $num_fruits will be 4
?>
You can use either the count() function or the sizeof() function in PHP, as they both produce the same results. The count() function is more commonly used, but using sizeof() is also acceptable.
The choice of which function to use is largely a matter of personal preference and coding style. Some developers prefer to use count() because it’s a bit more descriptive, while others prefer sizeof() because it’s shorter and easier to type.
In any case, it’s recommended to stick to one function and use it consistently throughout your code to maintain a consistent style.