How to Use the Ruby unpack Method
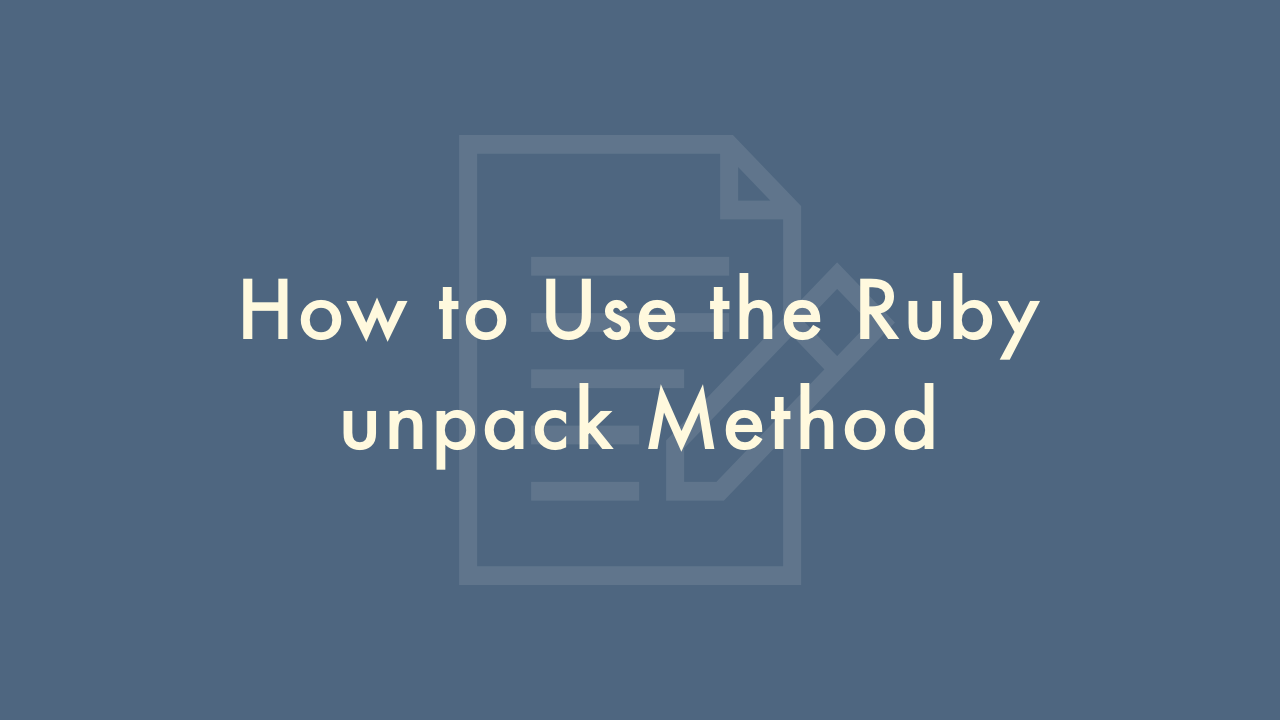
Contents
In this article, you will learn how to use the Ruby unpack method.
Using the unpack method
The unpack method in Ruby is a powerful tool for decoding binary data. It allows you to convert binary data into a series of Ruby objects, such as integers, strings, and floats.
The unpack method is available on Ruby strings, and takes a single argument, which is a string that specifies the format of the data you want to unpack. The format string can include a variety of codes that specify the type and size of each piece of data in the binary string.
Here’s an example of using the unpack method to convert a binary string into an integer:
binary_data = "\x01\x02\x03\x04"
integer_data = binary_data.unpack('N')
In this example, binary_data is a string containing four bytes of binary data. The unpack method is called on this string, with the argument ‘N’, which is a code that specifies that we want to convert the data into a 32-bit unsigned integer. The result of the unpack method is an array containing a single element, which is the integer value of the binary data.
Here are some other codes that can be used in the format string:
- C: 8-bit unsigned integer
- S: 16-bit unsigned integer
- L: 32-bit unsigned integer
- Q: 64-bit unsigned integer
- c: 8-bit signed integer
- s: 16-bit signed integer
- l: 32-bit signed integer
- q: 64-bit signed integer
- f: single-precision float
- d: double-precision float
You can also use these codes in combination with numeric prefixes to specify the size of each piece of data. For example, ‘S4’ would specify a 64-bit unsigned integer.
You can use the unpack method to extract multiple pieces of data from a binary string by including multiple format codes in the format string. For example:
binary_data = "\x01\x02\x03\x04\x05\x06"
integer_data, string_data = binary_data.unpack('N A*')
In this example, binary_data is a string containing six bytes of binary data. The unpack method is called with the argument ‘N A*’, which specifies that we want to extract a 32-bit unsigned integer followed by a string of arbitrary length. The A* code tells unpack to read all remaining bytes in the binary data as a string.