How to Use the select Method in Ruby
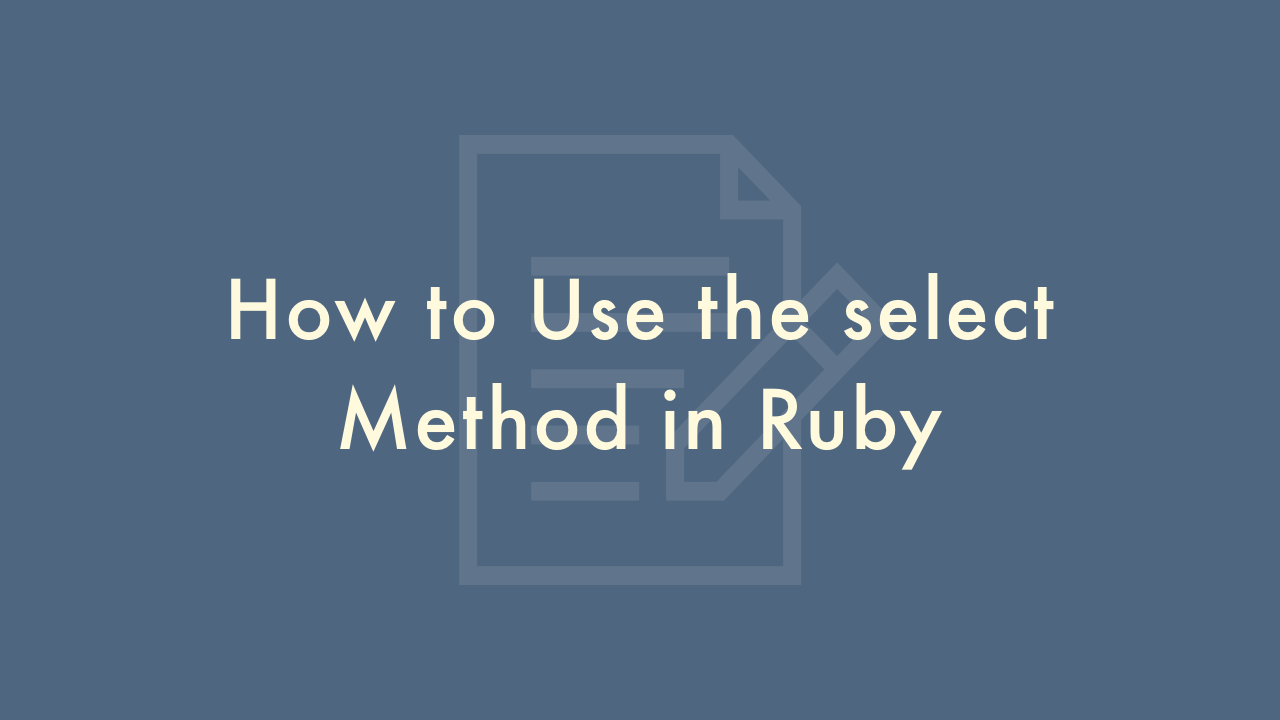
Contents
In this article, you will learn how to use the select method in Ruby.
The select Method
The select method in Ruby is used to filter elements from an array or a hash based on a condition specified in a block. Here’s how you can use the select method in Ruby:
Using select with an array
Suppose you have an array of integers and you want to select only the even numbers from it. You can do that using the select method as follows:
numbers = [1, 2, 3, 4, 5, 6]
even_numbers = numbers.select { |n| n.even? }
puts even_numbers.inspect # prints [2, 4, 6]
In the example above, the block passed to the select method returns true for even numbers (n.even?), and false otherwise. The select method returns a new array that contains only the elements for which the block returns true.
Using select with a hash
Suppose you have a hash containing the ages of people and you want to select only the people who are over 18 years old. You can do that using the select method as follows:
ages = { "Alice" => 25, "Bob" => 17, "Charlie" => 30 }
adults = ages.select { |name, age| age > 18 }
puts adults.inspect # prints {"Alice"=>25, "Charlie"=>30}
In the example above, the block passed to the select method takes two arguments (name and age) representing each key-value pair in the hash. The block returns true for pairs where the age is over 18 (age > 18), and false otherwise. The select method returns a new hash that contains only the key-value pairs for which the block returns true.
Here are some additional details about the select method in Ruby:
- The select method is also known as the filter method in other programming languages.
-
The select method can be chained with other array methods, allowing you to perform multiple operations on the selected elements. For example:
numbers = [1, 2, 3, 4, 5, 6] even_squares = numbers.select { |n| n.even? }.map { |n| n ** 2 } puts even_squares.inspect # prints [4, 16, 36]
In the example above, the select method is used to filter out the even numbers, and then the map method is used to square each of the selected elements.
-
If the block passed to the select method returns a truthy value (i.e., anything except false or nil), the element is considered selected. This means that you can use any condition that evaluates to a truthy or falsy value in the block. For example:
numbers = [1, 2, 3, 4, 5, 6] selected_numbers = numbers.select { |n| n > 3 && n < 6 } puts selected_numbers.inspect # prints [4, 5]
In the example above, the block selects only the numbers between 3 and 6 (exclusive) using the && (and) operator.
-
The select! method is a destructive version of the select method, which modifies the original array or hash instead of creating a new object. For example:
numbers = [1, 2, 3, 4, 5, 6] numbers.select! { |n| n.even? } puts numbers.inspect # prints [2, 4, 6]
In the example above, the select! method modifies the numbers array in place, removing all the odd numbers and leaving only the even numbers.