How to Use Pluck in Ruby on Rails
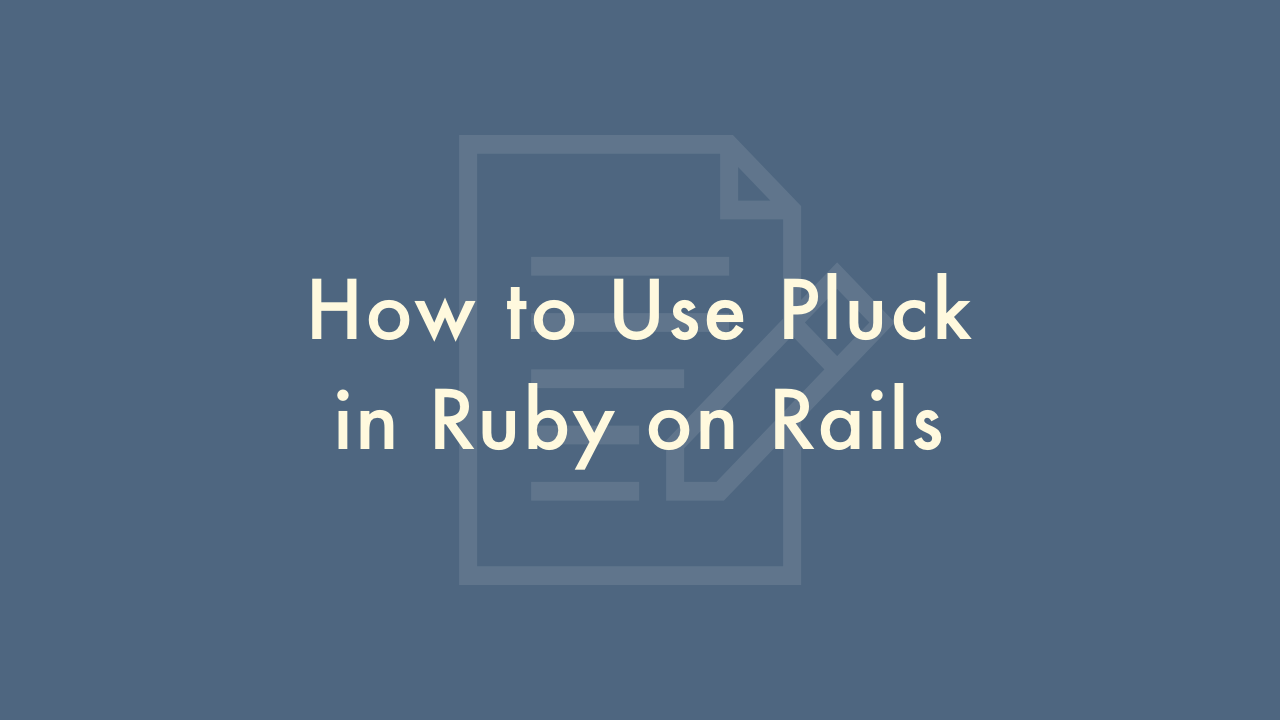
Contents
In this article, you will learn how to use pluck in Ruby on Rails.
How to use pluck
In Ruby on Rails, the pluck method is used to retrieve specific data from a database table. It returns an array of values for a specified column or set of columns in the database table.
Here’s an example of how to use pluck in Ruby on Rails:
Assume we have a model called Book and it has columns id, title, author, and published_date.
To retrieve an array of titles of all books in the books table, we can use pluck as follows:
titles = Book.pluck(:title)
This will return an array of all the titles in the books table.
To retrieve an array of both the title and author columns for all books, we can use pluck as follows:
title_author = Book.pluck(:title, :author)
This will return an array of arrays where each sub-array contains the title and author of a book.
Additionally, pluck can be used with conditions to retrieve data that meets specific criteria. For example, to retrieve an array of titles of books published before a certain date, we can use:
titles = Book.where('published_date < ?', some_date).pluck(:title)
This will return an array of titles of books published before some_date.
Overall, pluck is a useful method for retrieving specific data from a database table in Ruby on Rails.