How to Use the Ruby start_with? Method
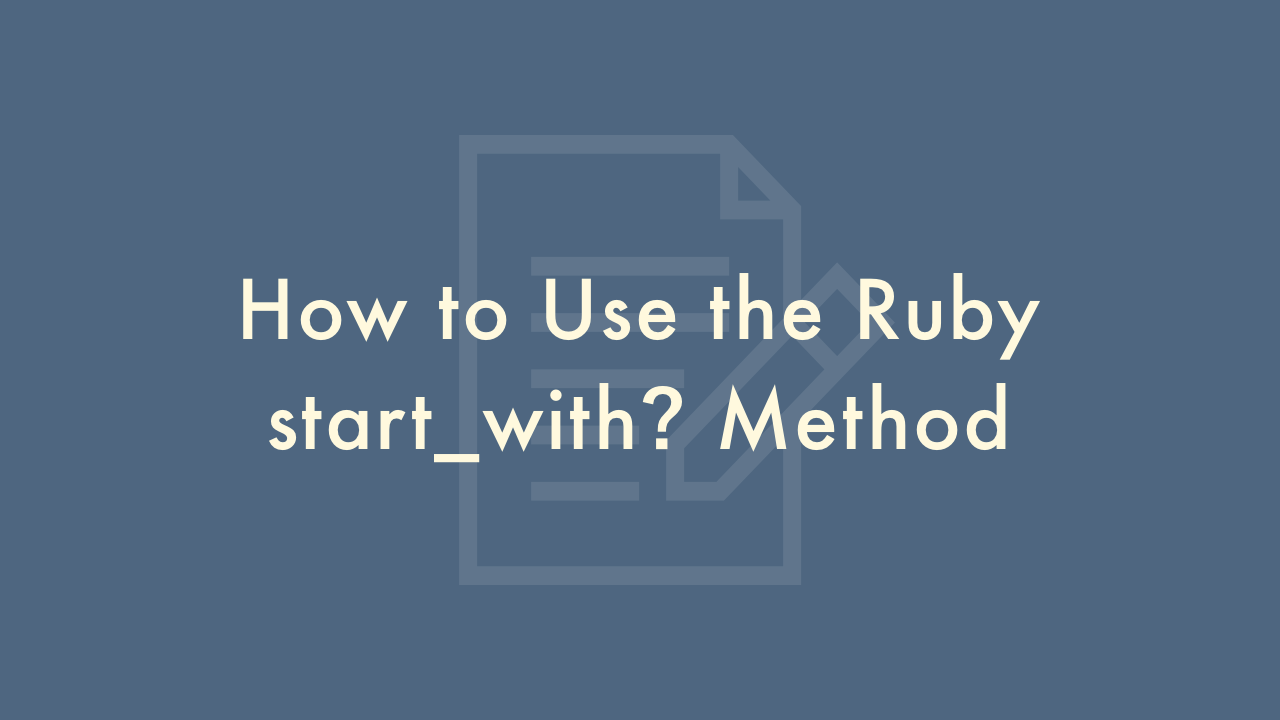
Contents
In this article, you will learn how to use the Ruby start_with? method.
Using the start_with? method
The start_with? method in Ruby is a useful method that allows you to check whether a given string starts with a particular prefix or not. It returns a Boolean value of true if the given string starts with the specified prefix, and false otherwise.
Here’s how you can use the start_with? method in your Ruby programs:
Syntax
The syntax for using the start_with? method is as follows:
string.start_with?(prefix1, prefix2, ...)
The start_with? method takes one or more arguments, which are the prefixes that you want to check for. You can specify multiple prefixes by passing them as separate arguments to the method.
Examples
Here are some examples of how you can use the start_with? method:
string = "Hello, World!"
# Check if the string starts with "Hello"
string.start_with?("Hello") # returns true
# Check if the string starts with "Hi"
string.start_with?("Hi") # returns false
# Check if the string starts with "Hello" or "Hi"
string.start_with?("Hello", "Hi") # returns true
# Check if the string starts with "world" (case-sensitive)
string.start_with?("world") # returns false
# Check if the string starts with "world" (case-insensitive)
string.downcase.start_with?("world") # returns true
In the first example, we’re checking if the string starts with the prefix “Hello”. Since the string does start with “Hello”, the method returns true.
In the second example, we’re checking if the string starts with the prefix “Hi”. Since the string does not start with “Hi”, the method returns false.
In the third example, we’re checking if the string starts with either “Hello” or “Hi”. Since the string starts with “Hello”, the method returns true.
In the fourth example, we’re checking if the string starts with the prefix “world”. However, since the prefix is in lowercase, and the string is in uppercase, the method returns false.
In the fifth example, we first convert the string to lowercase using the downcase method, and then we check if it starts with the prefix “world”. Since the string now starts with “world”, the method returns true.