How to Use the case Statement in Ruby
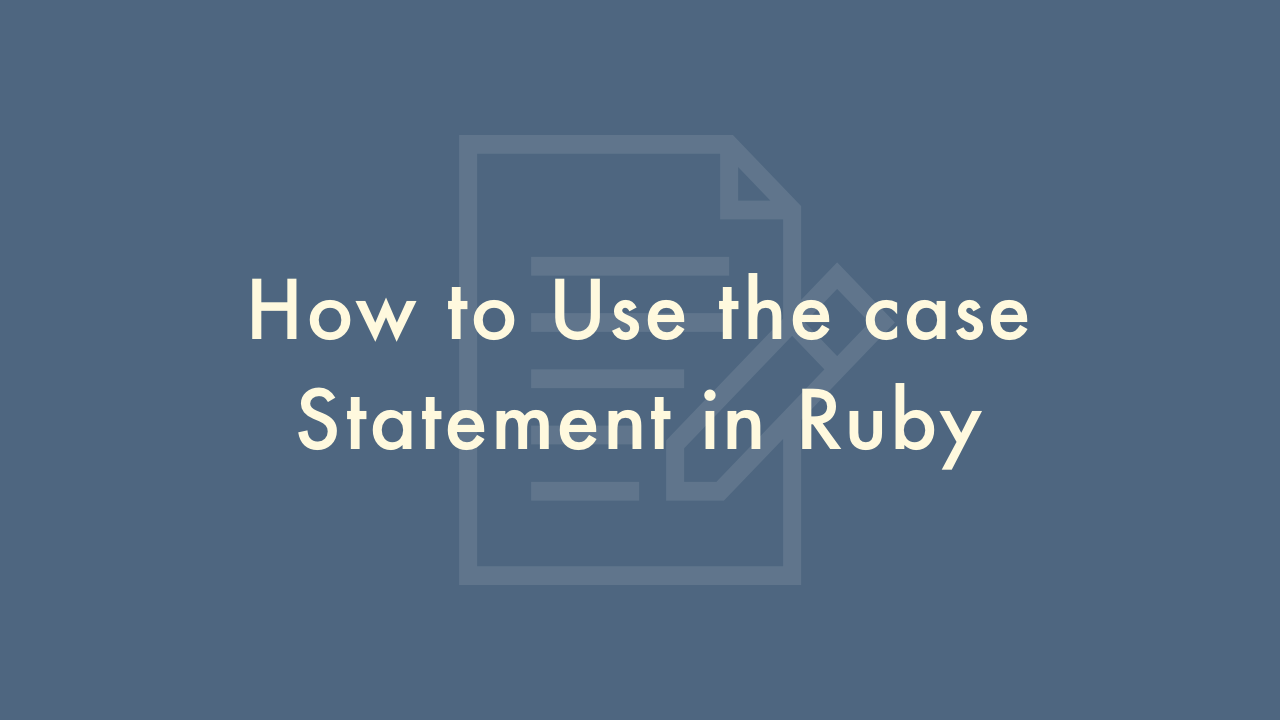
Contents
In this article, you will learn how to use the case statement in Ruby.
Ruby case Statement
In Ruby, the case statement is a powerful tool for implementing conditional logic. It is a cleaner and more concise way to write multiple if statements for checking the value of a variable or an expression against multiple conditions.
Here is an example of how to use the case statement in Ruby:
grade = "B"
case grade
when "A"
puts "Excellent"
when "B"
puts "Good"
when "C"
puts "Average"
when "D"
puts "Poor"
else
puts "Fail"
end
In this example, the case statement checks the value of the variable grade against different conditions. If grade is equal to “A”, the program outputs “Excellent”. If grade is equal to “B”, the program outputs “Good”. If grade is equal to “C”, the program outputs “Average”. If grade is equal to “D”, the program outputs “Poor”. If grade is not equal to any of these values, the program outputs “Fail”.
You can also use the case statement without a variable:
age = 20
case
when age < 18
puts "You are not yet an adult"
when age >= 18 && age < 65
puts "You are an adult"
else
puts "You are a senior"
end
In this example, the case statement checks different conditions without a variable. If the age is less than 18, the program outputs "You are not yet an adult". If the age is between 18 and 65, the program outputs "You are an adult". If the age is greater than or equal to 65, the program outputs "You are a senior".
Note that the case statement in Ruby is not limited to testing equality. You can also use ranges, regular expressions, and other comparisons.