How to Use the Ruby upcase Method
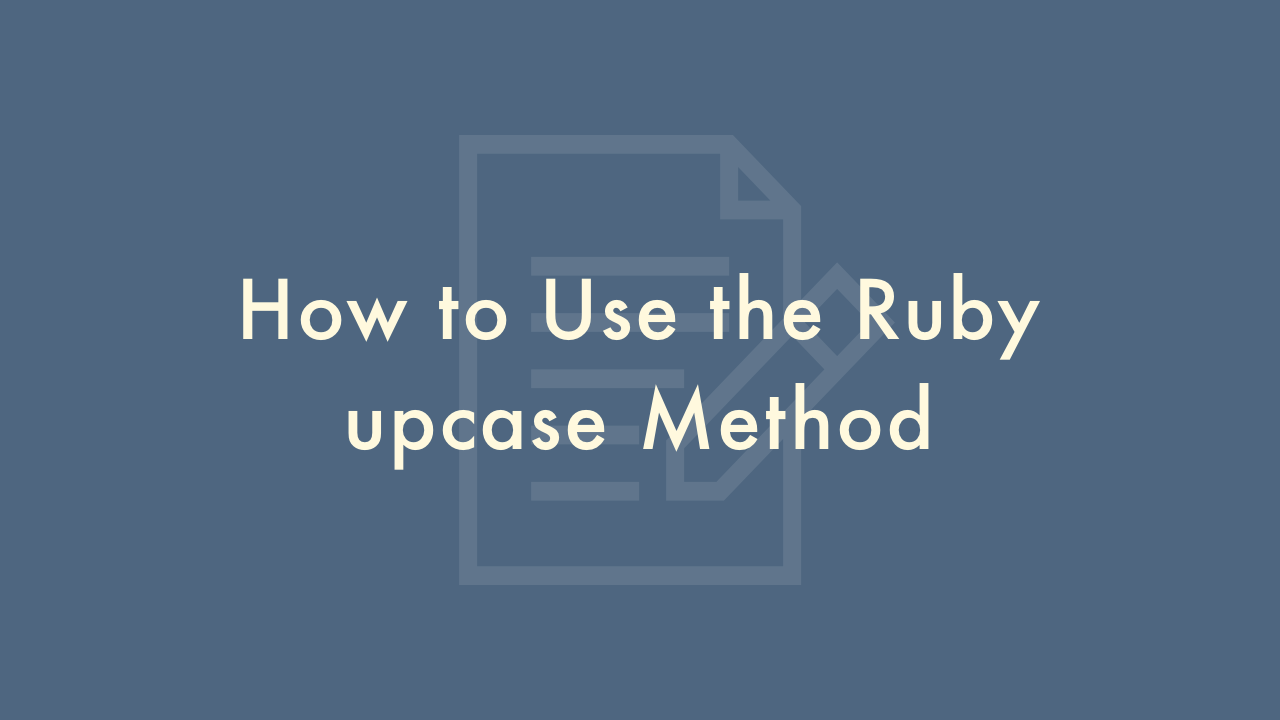
Contents
In this article, you will learn how to use the Ruby upcase method.
Using the upcase method
The upcase method in Ruby is used to convert all the lowercase characters in a string to uppercase. This method is very useful when you need to standardize the capitalization of text.
Syntax
The syntax of the upcase method is as follows:
string.upcase
Here, string is the variable or object that contains the string that you want to convert to uppercase.
Example
Let’s see an example of how to use the upcase method in Ruby:
name = "john doe"
puts name.upcase
In this example, we have a string “john doe”, which contains lowercase characters. The upcase method is called on the string variable name. The result of the method call is printed to the console using puts. The output of this code will be:
JOHN DOE
As you can see, all the lowercase characters in the string have been converted to uppercase.
Modifying the Original String
By default, the upcase method returns a new string with all the lowercase characters converted to uppercase. It does not modify the original string. If you want to modify the original string, you can use the upcase! method instead:
name = "john doe"
name.upcase!
puts name
In this example, we have used the upcase! method instead of upcase. This method modifies the original string instead of returning a new one. The output of this code will be:
JOHN DOE
Handling Non-ASCII Characters
The upcase method works correctly with ASCII characters, but it may not work as expected with non-ASCII characters. If you need to handle non-ASCII characters, you can use the unicode_normalize and upcase methods together. Here’s an example:
name = "Mañana"
puts name.unicode_normalize(:nfd).upcase.unicode_normalize(:nfkc)
In this example, we have a string “Mañana”, which contains a non-ASCII character (ñ). We first use the unicode_normalize method with the :nfd option to normalize the string to its decomposed form. We then call the upcase method to convert all the lowercase characters to uppercase. Finally, we use unicode_normalize again with the :nfkc option to normalize the string back to its composed form. The output of this code will be:
MAÑANA
As you can see, the non-ASCII character has been converted to uppercase correctly.