How to Use the Ruby strip Method
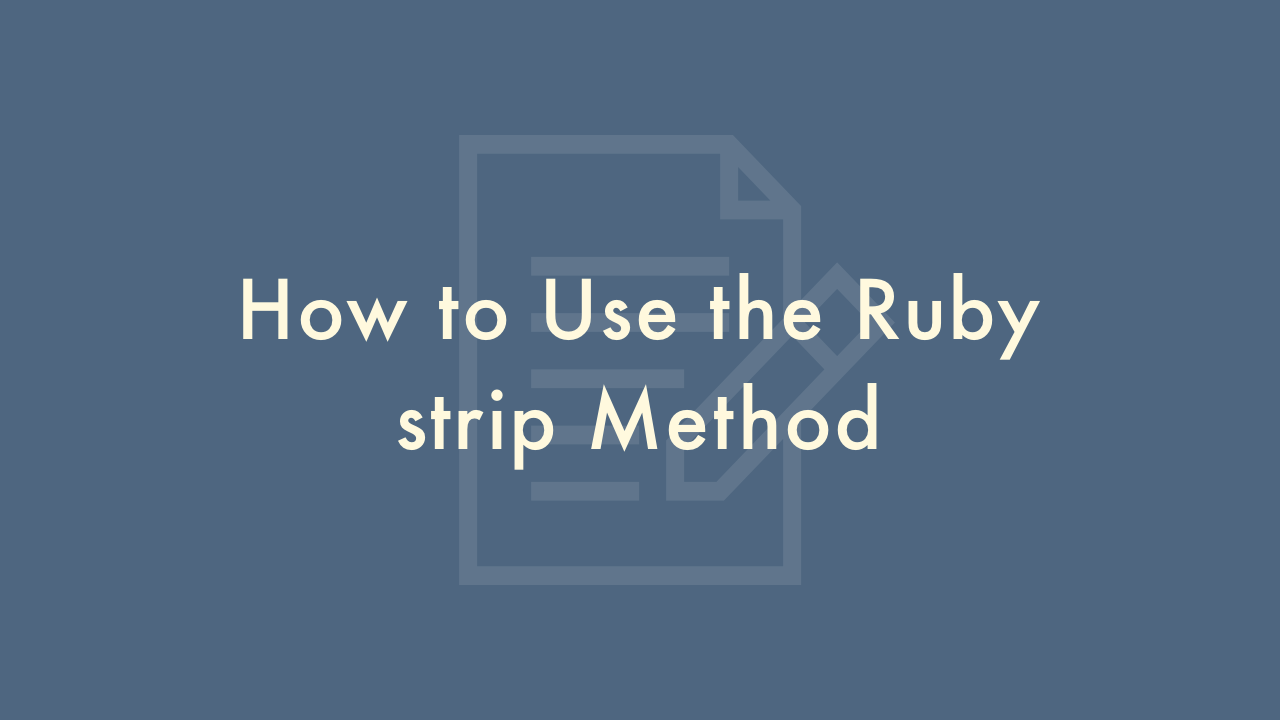
Contents
In this article, you will learn how to use the Ruby strip method.
Using the strip method
The strip method is a string method in Ruby that removes any leading or trailing whitespace from a given string. In other words, it removes any whitespace characters such as spaces, tabs, or newlines from the beginning and end of the string.
The strip method is very useful when you want to clean up user input or remove unwanted whitespace from strings. Here is how you can use the strip method in your Ruby code:
Basic usage
To use the strip method, you simply call it on a string object:
string = " hello, world "
clean_string = string.strip
puts clean_string
In this example, we have a string object string that contains some extra whitespace at the beginning and end. We then call the strip method on this string and assign the result to a new variable clean_string. Finally, we output the cleaned-up string using the puts command.
The output of this code will be:
hello, world
As you can see, the strip method has removed the extra whitespace from the beginning and end of the string.
Chaining methods
You can also chain the strip method with other string methods to perform more complex operations. For example, you can combine strip with gsub to remove all occurrences of a particular character from the string:
string = " h-e-l-l-o, w-o-r-l-d "
clean_string = string.strip.gsub('-', '')
puts clean_string
In this example, we first use strip to remove the whitespace from the beginning and end of the string. We then use gsub to remove all occurrences of the dash character (“-“) from the cleaned-up string. Finally, we output the result using puts.
The output of this code will be:
hello, world
As you can see, the strip method was used to remove the whitespace from the beginning and end of the string, and the gsub method was used to remove the dashes from the resulting string.
Modifying the original string
By default, the strip method does not modify the original string. Instead, it returns a new string object with the whitespace removed. However, if you want to modify the original string, you can use the strip! method instead:
string = " hello, world "
string.strip!
puts string
In this example, we call the strip! method on the original string object string. This modifies the string in place by removing the whitespace from the beginning and end. Finally, we output the modified string using puts.
The output of this code will be:
hello, world
As you can see, the original string has been modified in place to remove the whitespace.