How to Use the Ruby downto Method
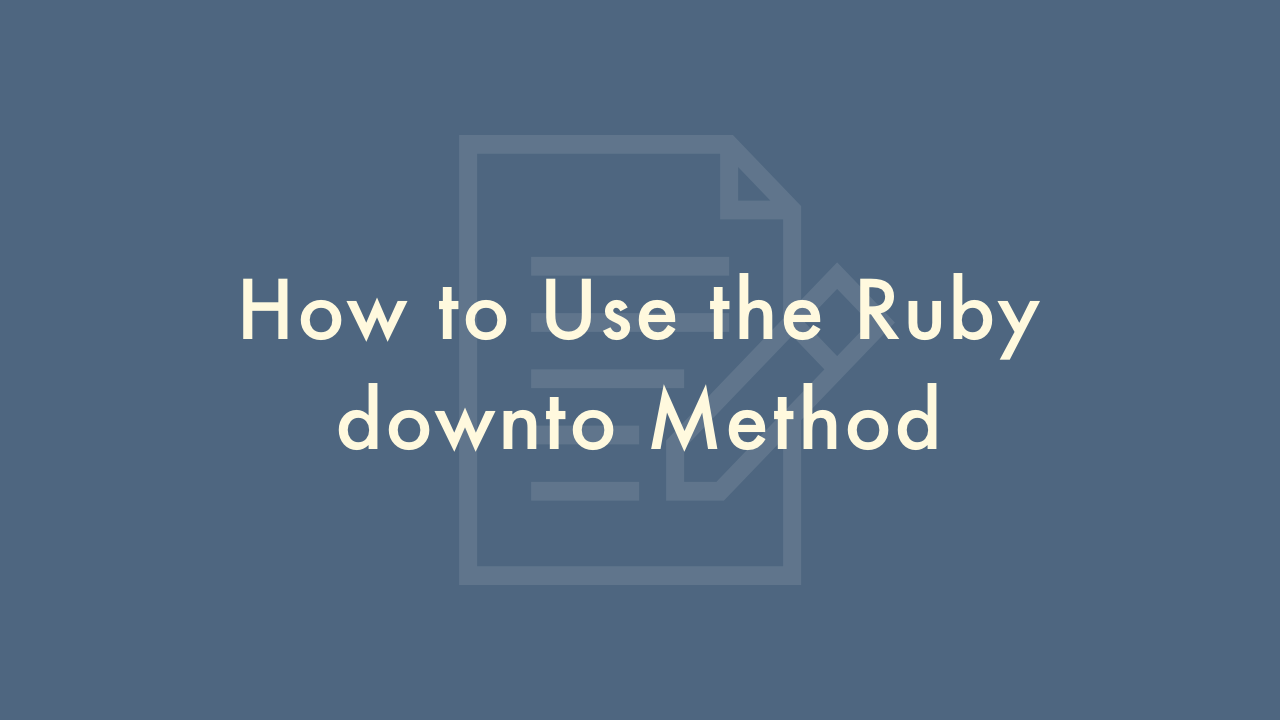
Contents
In this article, you will learn how to use the Ruby downto method.
Using the downto Method
The downto method is a built-in method in the Ruby programming language. It is used to iterate downwards from a given number to a specified limit, performing a block of code at each step.
Syntax
The syntax for using the downto method is as follows:
start.downto(stop) do |variable|
# Code to be executed
end
Parameters
start
: The starting number.stop
: The stopping number.variable
: The variable used to represent the current iteration value.
Examples
Printing numbers from 10 to 1
10.downto(1) do |i|
puts i
end
Output:
10
9
8
7
6
5
4
3
2
1
In the above example, we start at 10 and iterate downwards until we reach 1. At each step, we print the current value of the iterator variable i.
Summing up numbers from 1 to 10
sum = 0
10.downto(1) do |i|
sum += i
end
puts sum
Output:
55
In the above example, we start at 10 and iterate downwards until we reach 1. At each step, we add the current value of the iterator variable i to the variable sum. After the loop has completed, we print the value of sum.
Using a range object with downto
(1..10).downto(1) do |i|
puts i
end
Output:
10
9
8
7
6
5
4
3
2
1
In the above example, we use a range object (1..10) as the starting value for the downto method. This allows us to iterate over the range of values from 1 to 10 in a descending order.