How to Use the Ruby sort Method
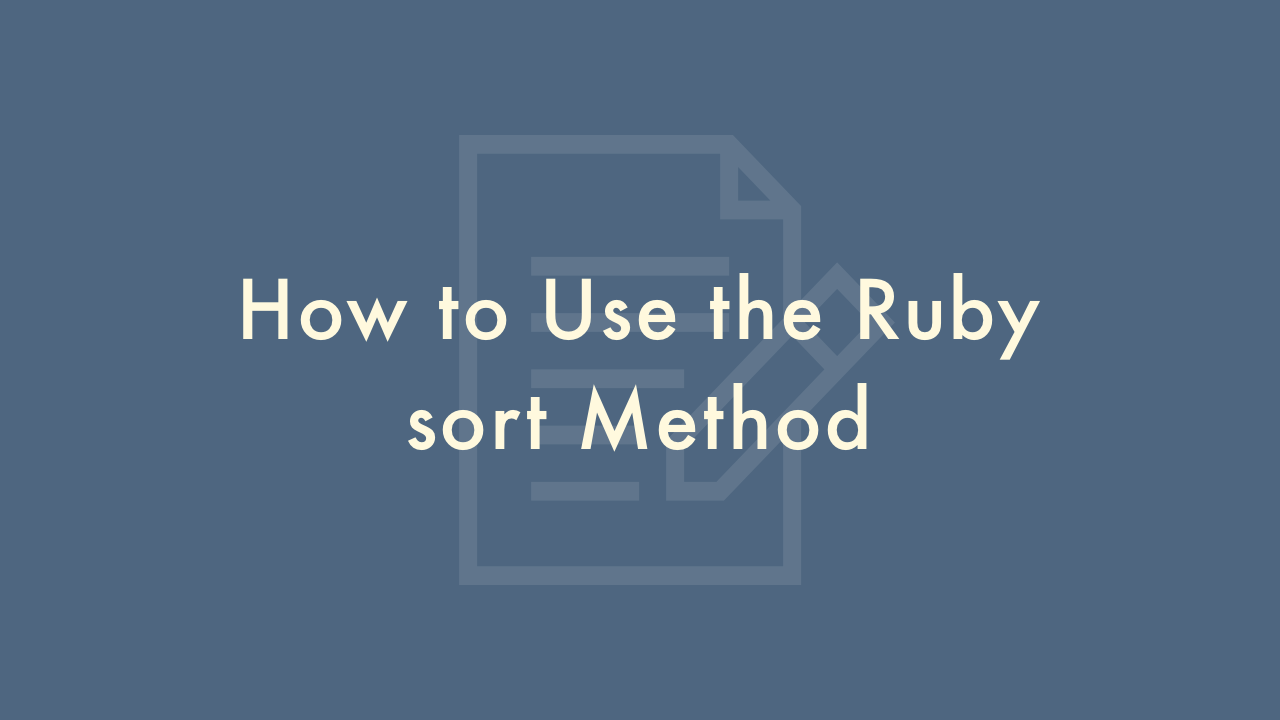
Contents
In this article, you will learn how to use the Ruby sort method.
Using the sort method
The sort method is a built-in Ruby method that can be used to sort arrays in ascending order by default. It can also be used to sort arrays in descending order or by a specific criterion defined by a block. Here’s how to use the sort method in Ruby:
Sorting Arrays in Ascending Order
To sort an array in ascending order using the sort method, simply call the method on the array you want to sort, like this:
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
sorted_numbers = numbers.sort
In this example, the sort method is called on the numbers array, and the sorted result is assigned to a new array called sorted_numbers. The resulting array will be [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9].
Sorting Arrays in Descending Order
To sort an array in descending order using the sort method, you can pass in a block that tells Ruby how to compare the elements of the array. The block should return -1, 0, or 1 depending on whether the first element should come before, at the same position as, or after the second element. Here’s an example:
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
sorted_numbers = numbers.sort { |a, b| b <=> a }
In this example, the block passed to the sort method compares each element to its neighboring element, swapping them if necessary so that the largest element ends up first. The resulting array will be [9, 6, 5, 5, 5, 4, 3, 3, 2, 1, 1].
Sorting Arrays by Specific Criteria
To sort an array by a specific criterion, you can also pass a block to the sort method that compares elements based on the criterion. Here’s an example:
fruits = ["banana", "apple", "pear", "orange", "kiwi"]
sorted_fruits = fruits.sort { |a, b| a.length <=> b.length }
In this example, the block passed to the sort method compares the length of each fruit name, sorting the array by the length of each name. The resulting array will be [“kiwi”, “pear”, “apple”, “banana”, “orange”].
Modifying the Original Array
It’s important to note that the sort method does not modify the original array, but instead returns a new array that is sorted. If you want to modify the original array, you can use the sort! method instead. Here’s an example:
numbers = [3, 1, 4, 1, 5, 9, 2, 6, 5, 3, 5]
numbers.sort!
In this example, the sort! method is called on the numbers array, modifying the array in place so that it is now sorted. The resulting array will be [1, 1, 2, 3, 3, 4, 5, 5, 5, 6, 9].