How to Use the Ruby encode Method
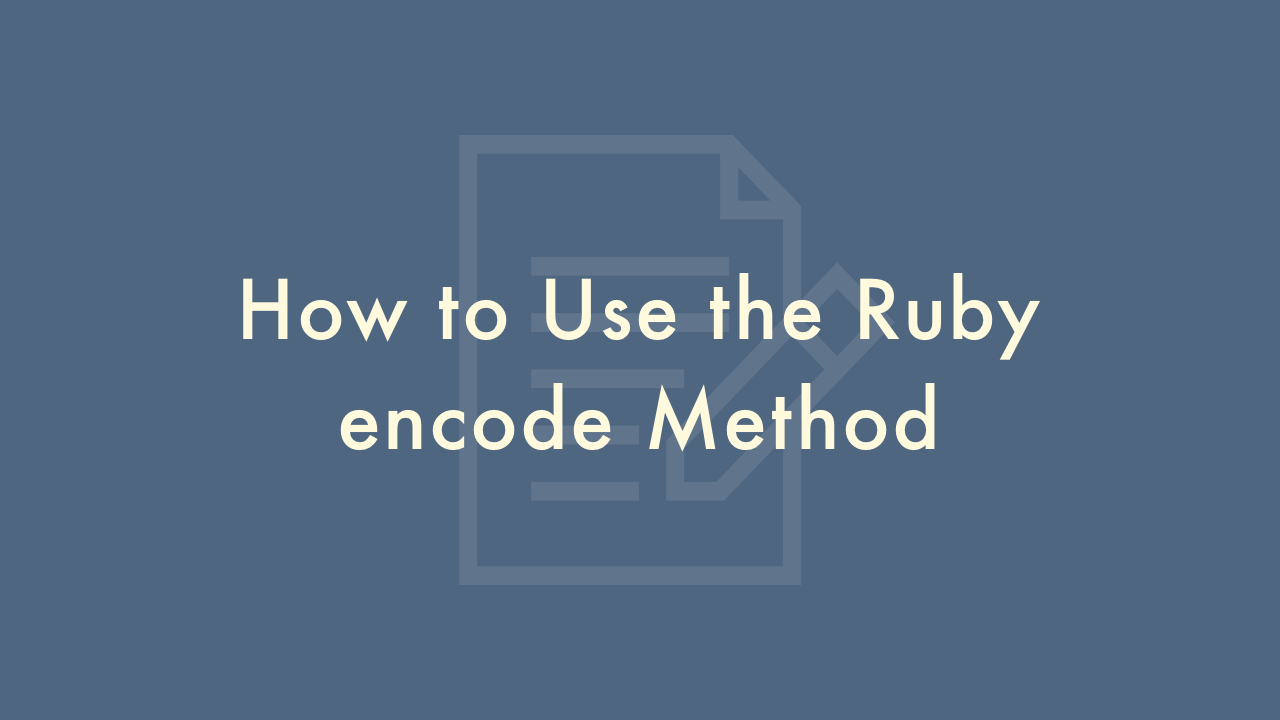
Contents
In this article, you will learn how to use the Ruby encode method.
Using the encode Method
The Ruby encode method is used to convert strings between different character encodings. It can be used to convert from one character encoding to another, or to validate if a string is valid in a certain encoding.
Here’s an example of how to use the encode method:
# encoding: UTF-8
str = "こんにちは" # Japanese "hello"
# Convert the string to UTF-16 encoding
utf16_str = str.encode("UTF-16")
# Convert the string to ASCII encoding, replacing any characters that cannot be represented
ascii_str = str.encode("ASCII", :replace => "_")
# Validate if the string is valid in UTF-8 encoding
valid_utf8 = str.encode("UTF-8", :invalid => :replace).valid_encoding?
puts utf16_str
puts ascii_str
puts valid_utf8
In this example, the string “こんにちは” is first encoded as UTF-8. Then, it is converted to UTF-16 encoding using the encode method. The resulting string is stored in the utf16_str variable.
Next, the string is converted to ASCII encoding using the encode method. Any characters that cannot be represented in ASCII are replaced with an underscore. The resulting string is stored in the ascii_str variable.
Finally, the encode method is used to validate if the string is valid in UTF-8 encoding. The valid_encoding? method is called on the resulting string to check if it is valid.
It’s important to note that the encode method does not modify the original string. Instead, it returns a new string with the specified encoding. If you want to modify the original string, you can assign the result back to the original variable.
# encoding: UTF-8
str = "こんにちは" # Japanese "hello"
# Convert the string to UTF-16 encoding and modify the original variable
str = str.encode("UTF-16")
puts str
This will output the string “こんにちは” encoded as UTF-16.