How to Use the Ruby byteslice Method
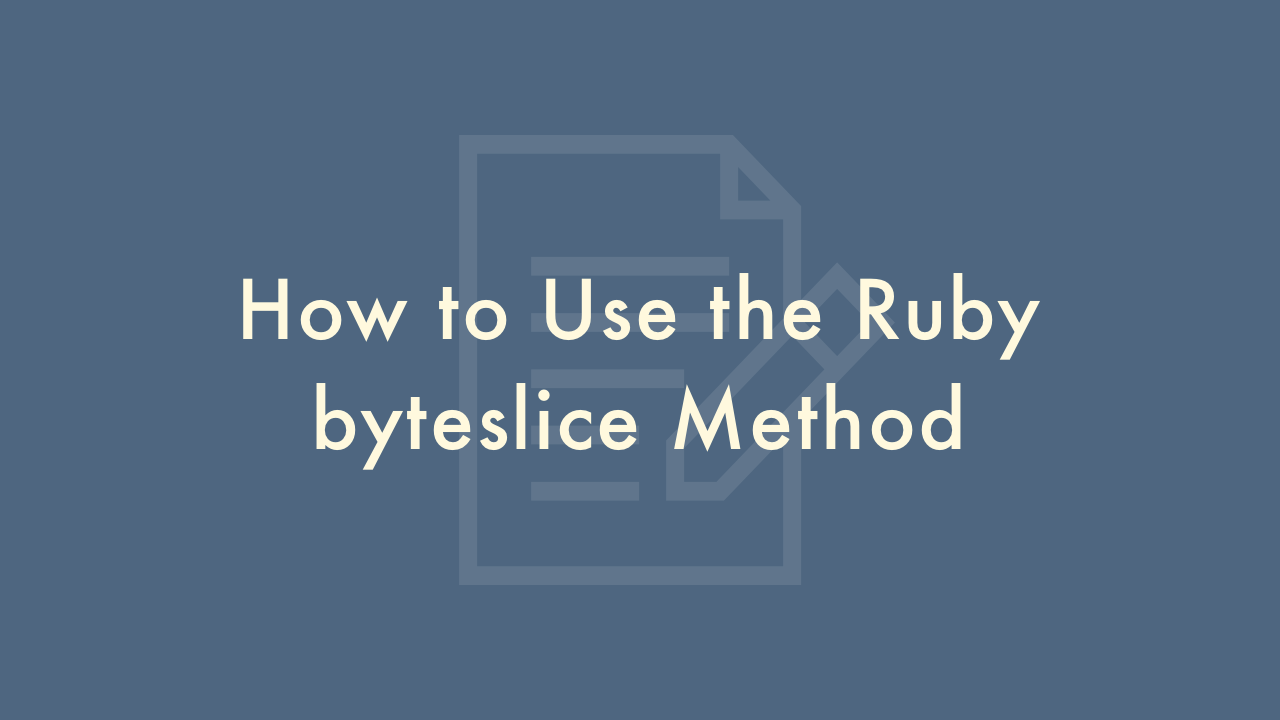
09/23/2021
Contents
In this article, you will learn how to use the Ruby byteslice method.
Using the byteslice method
The Ruby byteslice method is a useful tool for extracting a slice of bytes from a string. This method takes one or two arguments, and returns a new string containing the specified slice of bytes.
Syntax
str.byteslice(index)
str.byteslice(start, length)
The first argument specifies the index of the byte to extract. The second argument specifies the length of the slice to extract, starting at the byte with the specified index.
Example
str = "Hello, world!"
# Extract the byte at index 1
str.byteslice(1) #=> "e"
# Extract a slice of 3 bytes, starting at index 7
str.byteslice(7, 3) #=> "wor"
Here are some additional tips for using the byteslice method:
- The byteslice method works with both ASCII and Unicode strings.
-
If you pass a negative index as the first argument, it will count backwards from the end of the string:
str = "Hello, world!" # Extract the last byte str.byteslice(-1) #=> "!" # Extract a slice of 3 bytes, starting at the second-to-last byte str.byteslice(-2, 3) #=> "ld!"
-
If the specified index is out of bounds, the method returns nil:
str = "Hello, world!" # Try to extract a byte that is out of bounds str.byteslice(100) #=> nil
- The byteslice method is useful when working with binary data, such as when parsing network packets or reading/writing files in binary mode.