How to Use Includes in Ruby on Rails
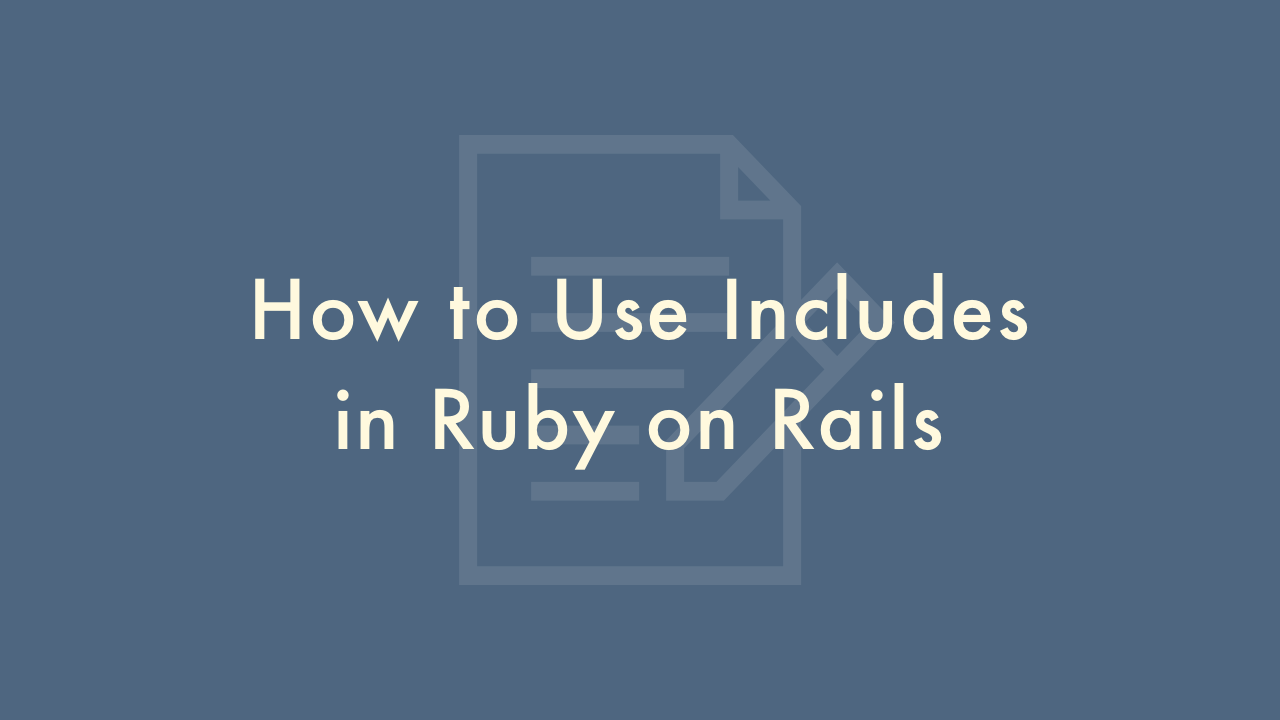
Contents
In this article, you will learn how to use includes in Ruby on Rails.
How to use includes
In Ruby on Rails, includes is a method that allows you to include associated objects in your database queries to improve performance. Here’s how to use includes in Ruby on Rails:
Define associations
Before you can use includes, you need to define associations between your models. For example, if you have a “User” model and a “Post” model, you might define a has_many association in the User model and a belongs_to association in the Post model.
Use includes in queries
Once you have defined your associations, you can use includes to load associated objects in your queries. For example, if you wanted to load all the posts for a particular user and include the user object in the query, you could use the following code:
@user = User.includes(:posts).find(params[:id])
This code will load the user with the specified ID and also load all the associated posts for that user in a single query. By using includes, you can avoid the N+1 query problem where Rails makes multiple queries to the database to load associated objects.
Access associated objects
Once you have included associated objects in your query, you can access them like any other object. For example, if you wanted to display the title of a user’s first post, you could use the following code:
@user.posts.first.title
This code will access the first post in the user’s posts collection and return its title.