How to Use the While Loop in Ruby
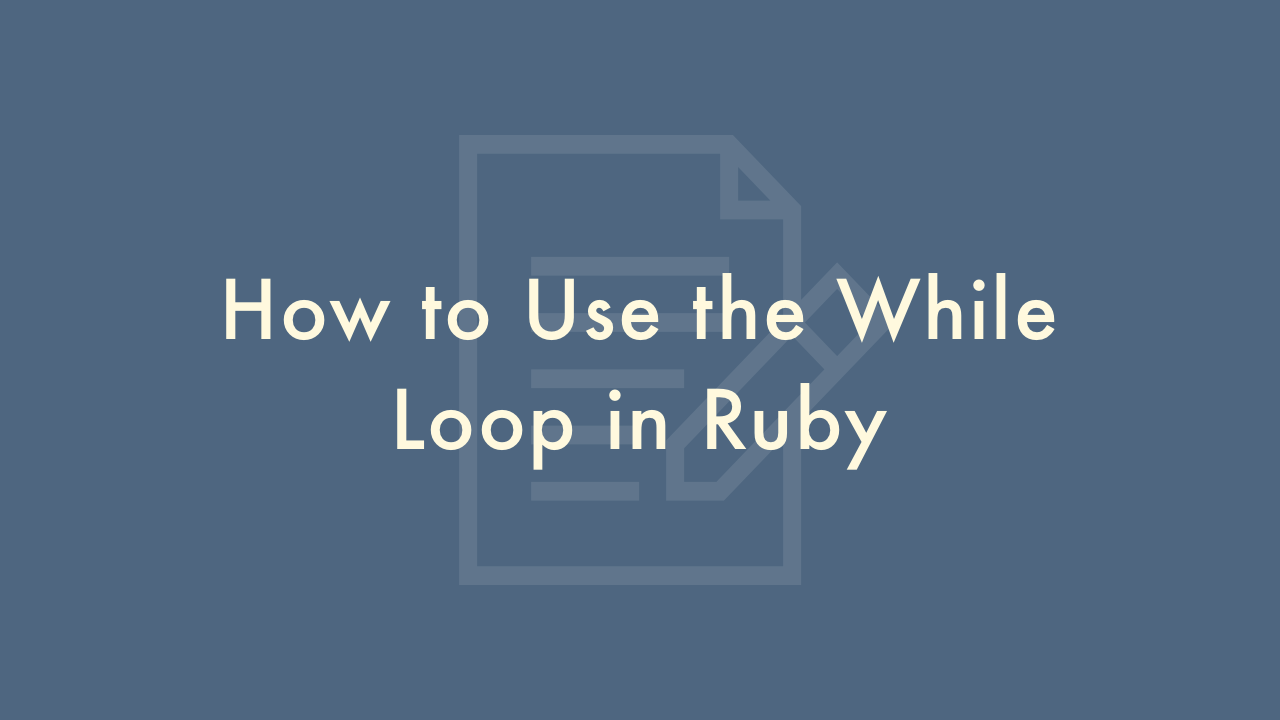
Contents
In this article, you will learn how to use the while loop in Ruby.
Ruby While Loop
In Ruby, the while loop is used to execute a block of code repeatedly as long as a certain condition is true. The syntax for the while loop in Ruby is:
while condition do
# Code to be executed repeatedly
end
Here, condition is an expression that returns either true or false. The block of code within the loop will be executed repeatedly as long as the condition remains true.
Let’s see an example:
i = 1
while i <= 10 do
puts i
i += 1
end
In this example, the loop will continue to execute as long as i is less than or equal to 10. The puts statement will output the current value of i, and then i is incremented by 1 using the += operator. This loop will output the numbers 1 through 10.
It's important to note that the condition within the while loop must eventually become false, or else the loop will execute indefinitely (also known as an infinite loop). To avoid this, you can use a break statement within the loop to exit early if a certain condition is met.
Here's an example of using a break statement within a while loop:
i = 1
while true do
puts i
i += 1
if i > 10
break
end
end
In this example, the loop will execute indefinitely because the condition true is always true. However, we use an if statement within the loop to check if i is greater than 10, and if it is, we use the break statement to exit the loop.