How to Use the merge Method in Ruby
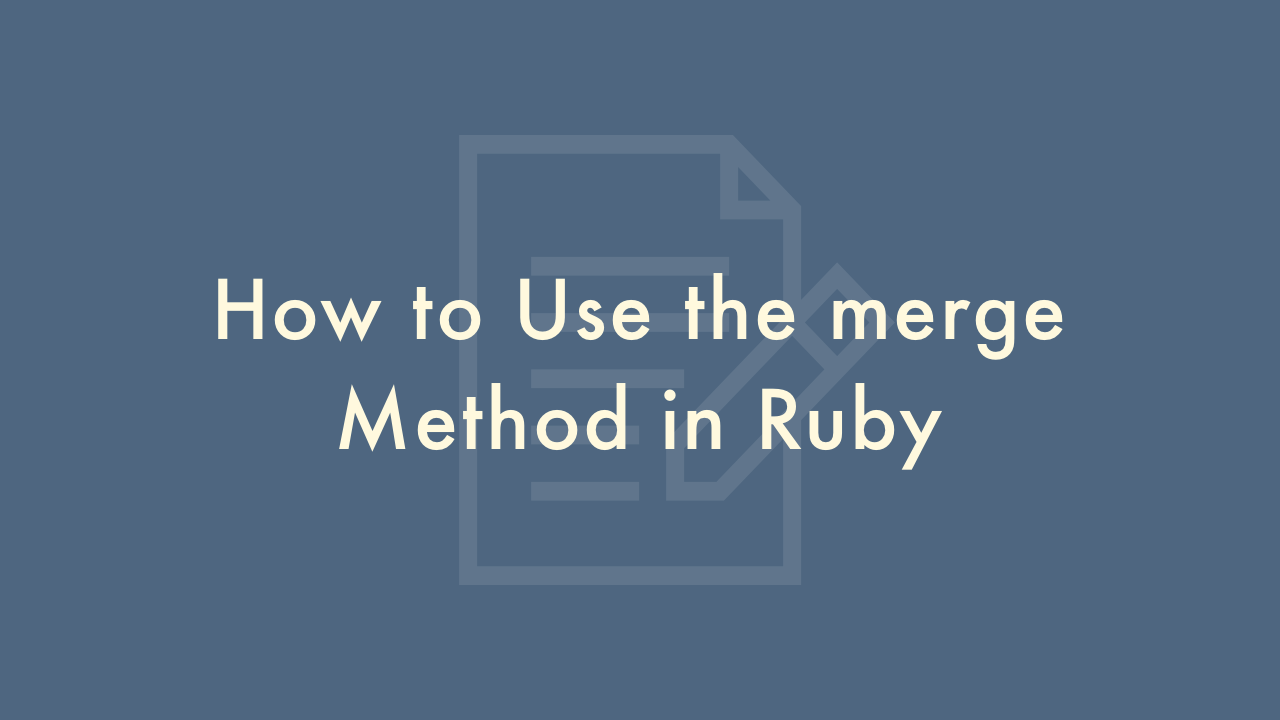
Contents
In this article, you will learn how to use the merge method in Ruby.
The merge Method
In Ruby, the merge method is used to combine two hashes into a new hash. Here is an example of how to use the merge method:
hash1 = { a: 1, b: 2 }
hash2 = { c: 3, d: 4 }
merged_hash = hash1.merge(hash2)
puts merged_hash #=> { a: 1, b: 2, c: 3, d: 4 }
In the example above, the merge method is called on hash1, and hash2 is passed as an argument. The result of the merge is stored in the variable merged_hash. The resulting hash contains all the key-value pairs from both hash1 and hash2.
If there are key-value pairs in both hash1 and hash2 that have the same key, the value from hash2 will overwrite the value from hash1 in the resulting hash. You can also pass a block to the merge method to specify how conflicting values should be handled.
Here is an example of using a block with merge:
hash1 = { a: 1, b: 2 }
hash2 = { b: 3, c: 4 }
merged_hash = hash1.merge(hash2) { |key, old_val, new_val| old_val + new_val }
puts merged_hash #=> { a: 1, b: 5, c: 4 }
In the example above, there is a conflict for the key :b between hash1 and hash2. The block passed to merge specifies that conflicting values should be added together. The resulting hash has a value of 5 for the key :b.