How to Use the Unless Statement in Ruby
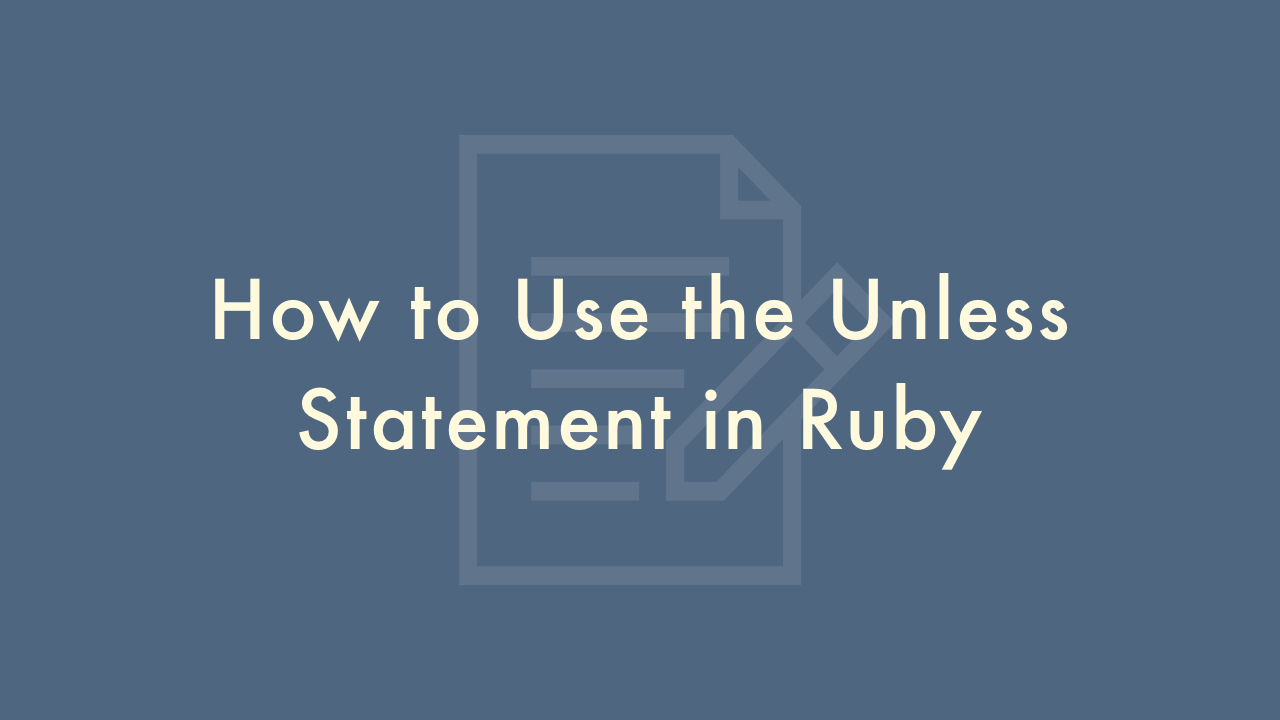
Contents
In this article, you will learn how to use the unless statement in Ruby.
The Unless Statement
In Ruby, the unless statement is used to execute a block of code if a condition is false. Here’s the basic syntax of an unless statement in Ruby:
unless condition
# code to be executed if the condition is false
end
Alternatively, you can use the one-liner syntax, which is useful for simple conditions:
# code to be executed unless the condition is true
code unless condition
Here’s an example of how to use unless in Ruby:
x = 10
unless x > 20
puts "x is not greater than 20"
end
# Output: "x is not greater than 20"
In the above example, the block of code is executed because the condition x > 20 is false.
You can also use else and elsif statements with unless to handle other conditions. Here’s an example:
x = 5
unless x > 10
puts "x is not greater than 10"
elsif x > 5
puts "x is greater than 5"
else
puts "x is less than or equal to 5"
end
# Output: "x is less than or equal to 5"
In the above example, the first condition x > 10 is false, so the code inside the unless block is executed. Since there is an elsif statement, the program checks the next condition x > 5. This condition is also false, so the code inside the else block is executed, which prints “x is less than or equal to 5”.