How to Use the replace Method in Ruby
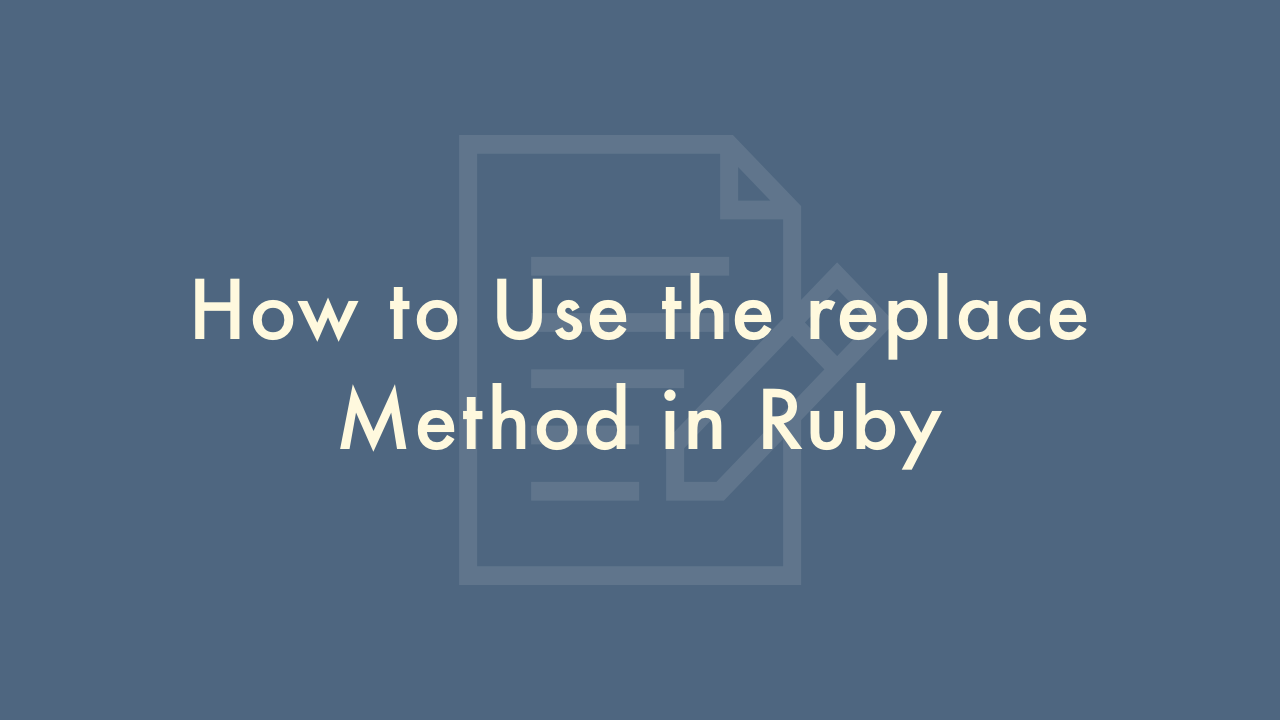
Contents
In this article, you will learn how to use the replace method in Ruby.
The replace method
In Ruby, the replace method is used to replace the contents of a string or array with another string or array.
Syntax
The replace method can be called on a string or array object and takes one argument, which is either a string or array.
For a string, the syntax is:
string.replace(new_string)
For an array, the syntax is:
array.replace(new_array)
Return Value
The replace method returns the modified object.
Using replace with a string
string = "Hello, World!"
string.replace("Hi, there!")
puts string
# Output: Hi, there!
In the above example, the replace method replaces the contents of the string variable with the new string “Hi, there!”.
Using replace with an array
array = [1, 2, 3, 4, 5]
array.replace([6, 7, 8])
puts array
# Output: [6, 7, 8]
In the above example, the replace method replaces the contents of the array variable with a new array [6, 7, 8].
Using replace to clear an array
One common use of the replace method is to clear the contents of an array.
array = [1, 2, 3, 4, 5]
array.replace([])
puts array
# Output: []
In the above example, we replace the contents of array with an empty array, effectively clearing its contents.
Using replace to convert a string to an array and vice versa
You can also use the replace method to convert a string to an array and vice versa.
string = "1,2,3,4,5"
array = string.split(",")
puts array
# Output: [1, 2, 3, 4, 5]
array.replace(array.join("-"))
puts string
# Output: "1-2-3-4-5"
In the above example, we first split the string into an array using the split method, and then replace the contents of the array with a new string created by joining the elements of the array with a hyphen.
Modifying the original object
The replace method modifies the original object instead of creating a new one. This means that if you have multiple references to the same object, all of them will be affected by the replace method.
string1 = "Hello"
string2 = string1
string1.replace("Hi")
puts string2
# Output: Hi
In the above example, both string1 and string2 refer to the same string object. When we call the replace method on string1, it modifies the contents of the original string object, which is also reflected in string2.