How to Use Classes in Ruby
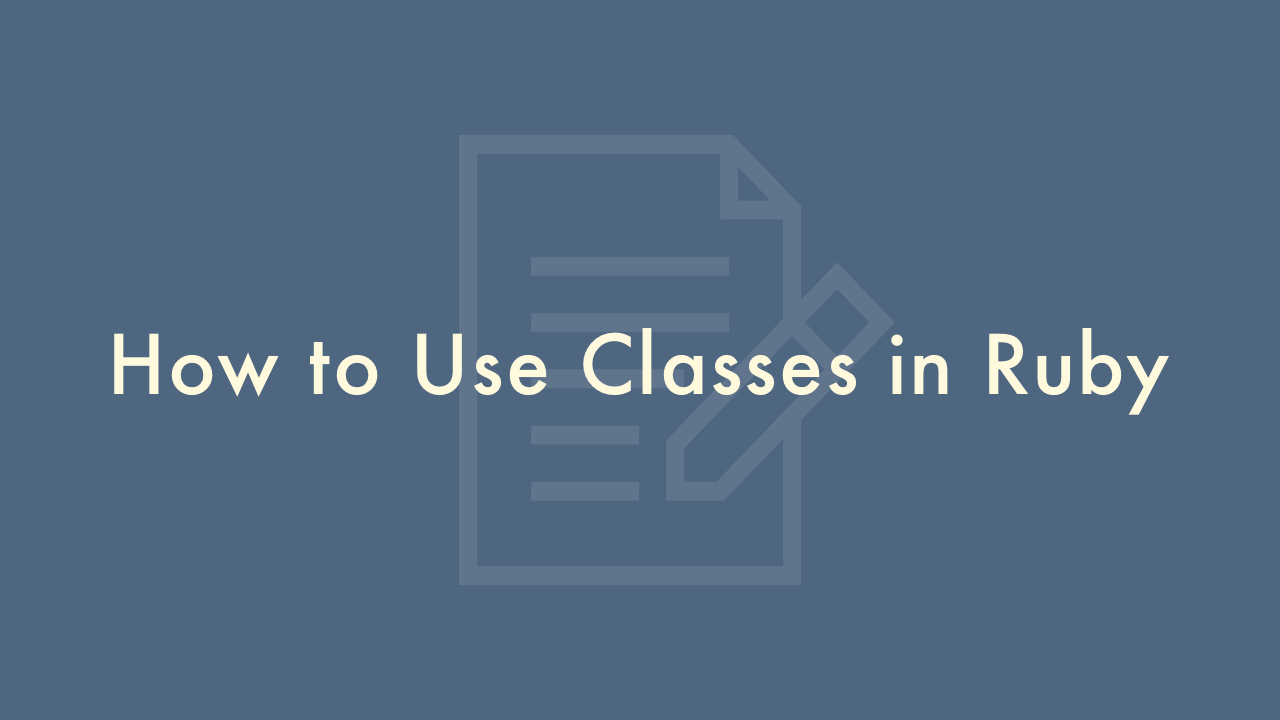
Contents
In this article, you will learn how to use classes in Ruby.
How to use classes
Classes in Ruby are used to define objects that encapsulate data and behavior. Here is a basic example of how to create and use classes in Ruby:
class Person
attr_accessor :name, :age
def initialize(name, age)
@name = name
@age = age
end
def say_hello
puts "Hello, my name is #{@name} and I am #{@age} years old."
end
end
# create a new instance of the Person class
person = Person.new("John", 30)
# access and modify instance variables
person.name = "Jane"
person.age = 35
# call instance methods
person.say_hello
In this example, we define a Person class with instance variables name and age, an initializer method that sets those variables, and a say_hello method that prints a message using those variables. We then create a new instance of the Person class and modify its variables and call its methods.
Here’s a step-by-step breakdown of how to create and use classes in Ruby:
- Define the class using the class keyword and a class name (in this example, Person).
- Define instance variables using the attr_accessor keyword. This allows us to access and modify those variables using methods.
- Define an initializer method using the initialize keyword. This method is called when a new instance of the class is created using the new method.
- Define other methods that operate on the instance variables. In this example, we define a say_hello method that prints a message using the instance variables.
- Create a new instance of the class using the new method and passing any necessary arguments to the initializer method.
- Access and modify instance variables using their respective methods.
- Call instance methods on the object using dot notation.
This is just a basic example of how to use classes in Ruby. Classes can be much more complex and powerful, allowing you to create objects that can interact with each other and with other parts of your code.