How to Use the extend Method in Ruby
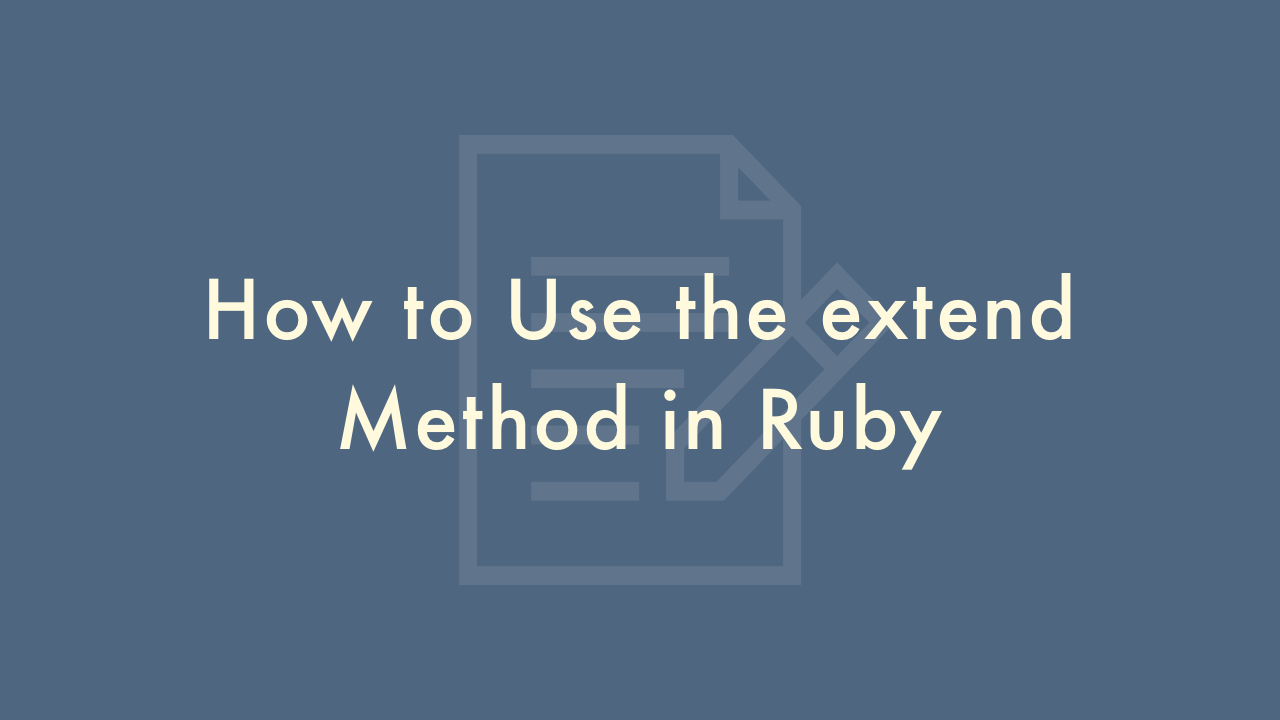
09/21/2021
Contents
In this article, you will learn how to use the extend method in Ruby.
The extend Method
In Ruby, the extend method is used to extend a class with a module’s methods and constants, effectively adding them to the class as if they were defined within the class itself. Here’s how you can use the extend method:
Define a module with the methods and constants you want to add to your class:
module MyModule
def my_method
puts "Hello, World!"
end
MY_CONSTANT = 42
end
Create a class that you want to extend with the module:
class MyClass
end
Use the extend method to add the module’s methods and constants to the class:
MyClass.extend(MyModule)
Now you can call the methods and constants defined in the module as if they were defined within the class:
MyClass.my_method # output: Hello, World!
puts MyClass::MY_CONSTANT # output: 42
Note that the extend method adds the module’s methods and constants to the class itself, not to instances of the class. If you want to add the module’s methods and constants to instances of the class, you should use the include method instead.