How to Use BCrypt Gem
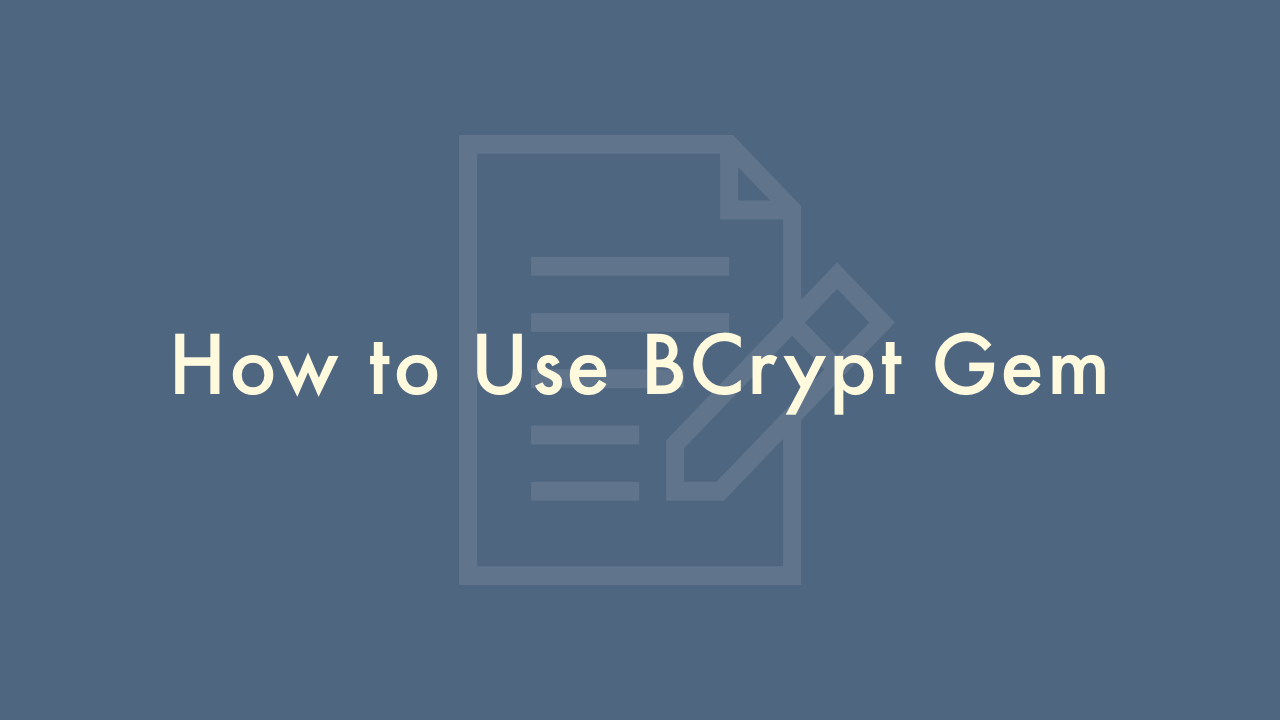
09/25/2021
Contents
In this article, you will learn how to use BCrypt Gem.
Using BCrypt Gem
The BCrypt gem is a popular Ruby gem for hashing and encrypting passwords. Here are the steps to use the BCrypt gem in your Ruby project:
Install the BCrypt gem:
gem install bcrypt
Require the BCrypt gem in your Ruby file:
require 'bcrypt'
To generate a hashed password, you can use the BCrypt::Password.create method:
hashed_password = BCrypt::Password.create("password")
To verify if a password matches a hash, use the == method:
if BCrypt::Password.new(hashed_password) == "password"
# password matches hash
else
# password does not match hash
end
You can also control the level of computational effort required to hash passwords by passing a cost parameter to the create method. The higher the cost, the more secure the hash, but the longer it takes to generate. For example, to use a cost of 12:
hashed_password = BCrypt::Password.create("password", cost: 12)