How to Use the For Loop in Ruby
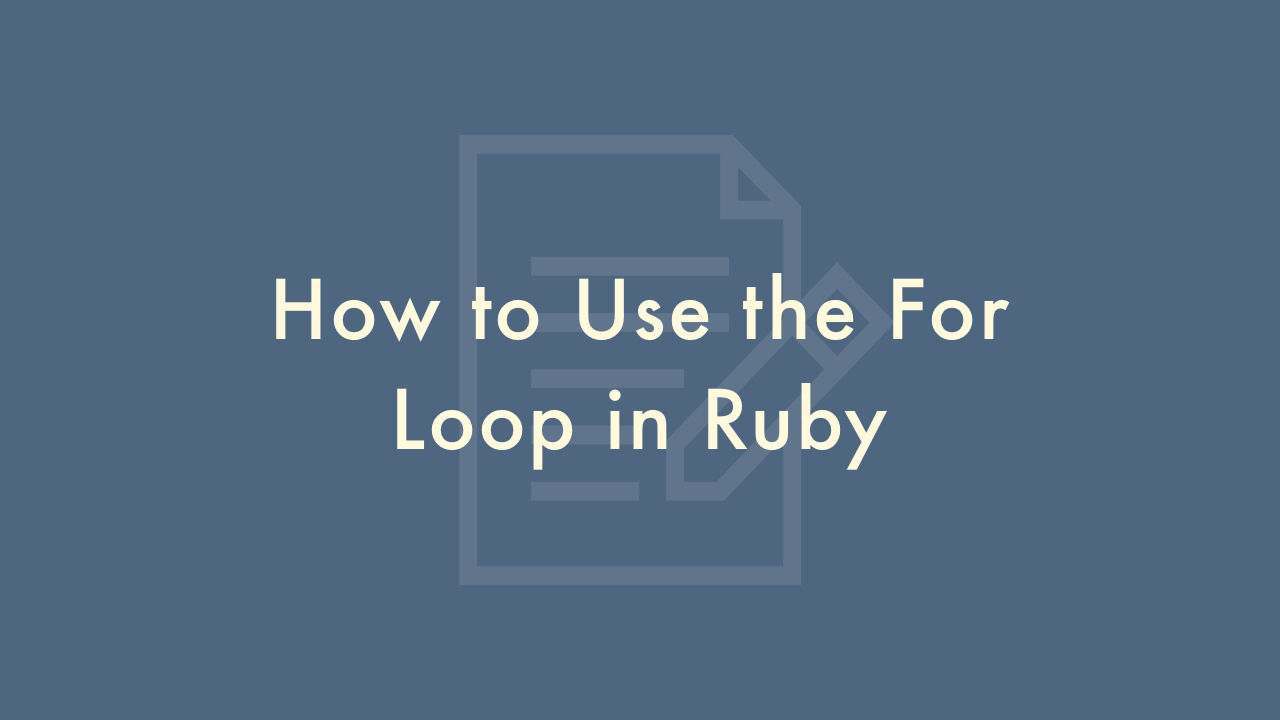
Contents
In this article, you will learn how to use the for loop in Ruby.
Ruby For Loop
In Ruby, a for loop is used to iterate over a collection of objects, such as an array or a range. Here’s an example of how to use a for loop in Ruby:
# iterate over an array
fruits = ["apple", "banana", "orange"]
for fruit in fruits
puts fruit
end
# iterate over a range
for i in 1..5
puts i
end
In the first example, the for loop is used to iterate over an array of fruits. The loop will run for each element in the fruits array, and the variable fruit will be set to the current element in each iteration. The puts method is then used to output the value of fruit to the console.
In the second example, the for loop is used to iterate over a range of numbers from 1 to 5. The loop will run for each number in the range, and the variable i will be set to the current number in each iteration. The puts method is then used to output the value of i to the console.
Note that while for loops are commonly used in other programming languages, in Ruby, it’s more common to use iterators such as each to loop over collections. Here’s an example of how to iterate over an array using the each method:
fruits = ["apple", "banana", "orange"]
fruits.each do |fruit|
puts fruit
end
This achieves the same result as the previous for loop example, but using a more idiomatic Ruby syntax.