How to Use the Ruby first Method
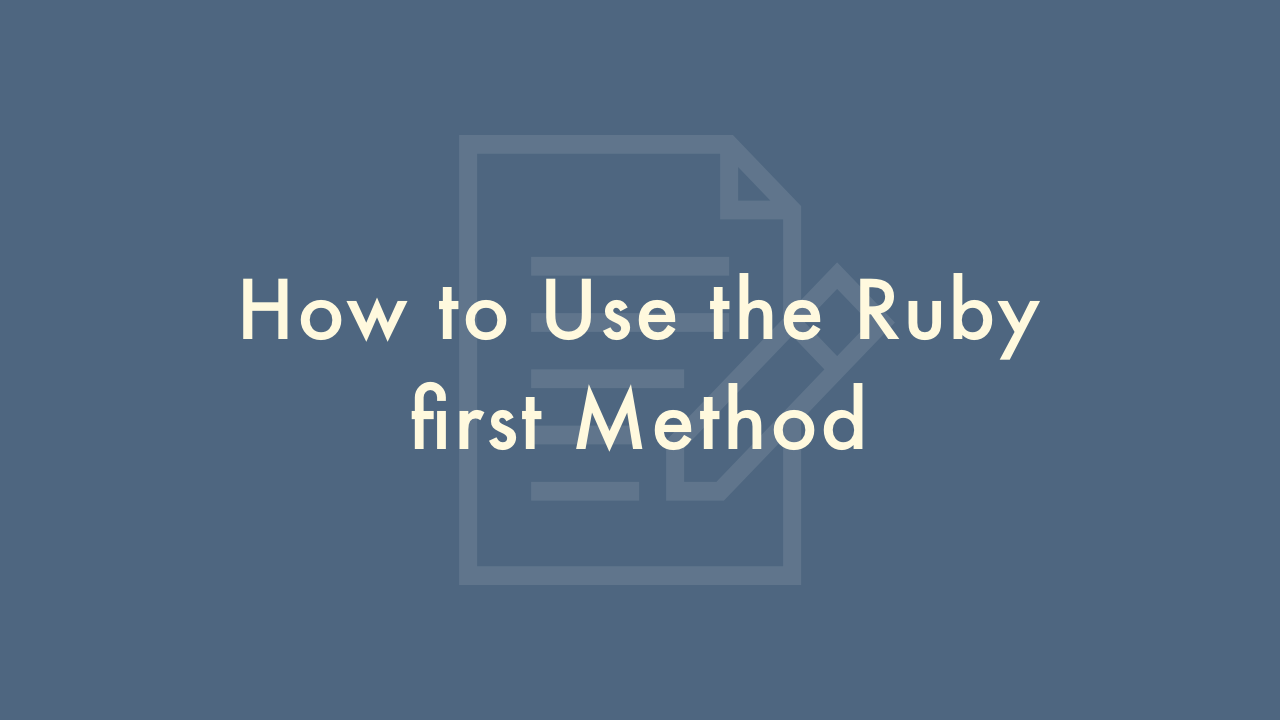
Contents
In this article, you will learn how to use the Ruby first method.
Using the first method
The first method in Ruby is a built-in method that is used to retrieve the first element of an array or the first n elements of an array.
Using the first method with arrays
The first method can be used to retrieve the first element or the first n elements of an array. Here are some examples:
# Retrieve the first element of an array
fruits = ["apple", "banana", "orange"]
puts fruits.first # Output: "apple"
# Retrieve the first two elements of an array
numbers = [1, 2, 3, 4, 5]
puts numbers.first(2) # Output: [1, 2]
In the first example, the first method is called on the fruits array without an argument, which retrieves the first element of the array (“apple”). In the second example, the first method is called with an argument of 2, which retrieves the first two elements of the numbers array ([1, 2]).
Using the first method with block
The first method can also be used with a block to find the first element that satisfies a condition. Here’s an example:
# Find the first even number in an array
numbers = [1, 3, 4, 5, 7, 8]
puts numbers.first { |n| n.even? } # Output: 4
In this example, the first method is called on the numbers array with a block that checks if the element is even. The first method returns the first element that satisfies the condition, which is 4.
Using the first method with an empty array
If the array is empty and the first method is called without an argument, it returns nil. If the first method is called with an argument, it returns an empty array. Here are some examples:
# Retrieve the first element of an empty array
empty_array = []
puts empty_array.first # Output: nil
# Retrieve the first two elements of an empty array
puts empty_array.first(2) # Output: []
In the first example, the first method is called on an empty array, which returns nil. In the second example, the first method is called with an argument of 2 on an empty array, which returns an empty array.