How to Use the Ruby loop Method
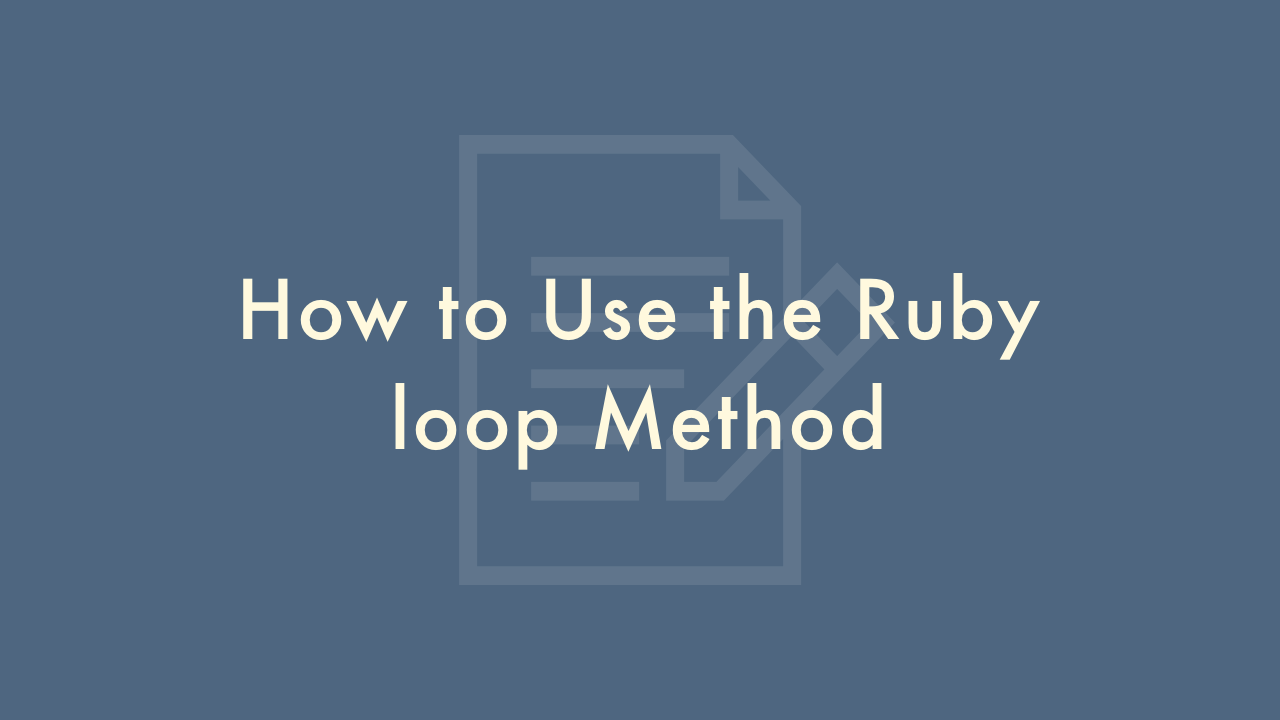
Contents
In this article, you will learn how to use the Ruby loop method.
Using the loop method
In Ruby, the loop method is used to create an infinite loop that will continue running until it is explicitly stopped using a break statement. Here’s how to use the loop method:
Basic loop example
loop do
puts "Hello, world!"
end
In this example, the loop method is used to create an infinite loop that continuously prints “Hello, world!” to the console. To stop the loop, you can use Ctrl+C to interrupt the execution of the program.
Loop with a condition
i = 0
loop do
i += 1
puts i
break if i == 10
end
In this example, we use the loop method to count from 1 to 10. The loop continues until i equals 10, at which point the break statement is used to exit the loop.
Loop with a block
loop {
print "Enter your name: "
name = gets.chomp
break if name == "quit"
puts "Hello, #{name}!"
}
In this example, the loop method is used to prompt the user to enter their name. The loop continues until the user enters “quit”, at which point the break statement is used to exit the loop.