How to Use Hashes in Ruby on Rails
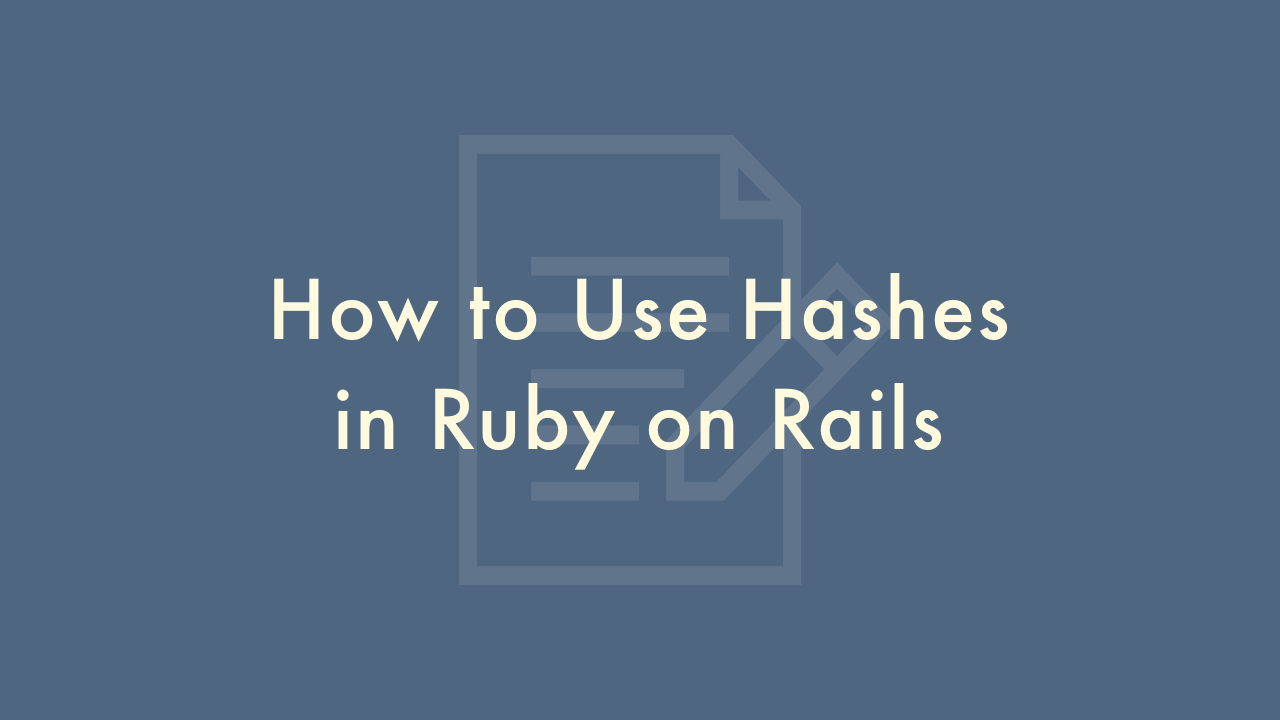
Contents
In this article, you will learn how to use hashes in Ruby on Rails.
Using hashes
In Ruby on Rails, hashes are a commonly used data structure for storing key-value pairs. They are similar to arrays, but instead of indexing elements by integers, they use keys to retrieve values.
Here’s a quick guide on how to use hashes in Ruby on Rails:
Creating a hash
You can create a new hash using curly braces {} or the Hash.new method. Here are some examples:
# Using curly braces
my_hash = { "key1" => "value1", "key2" => "value2" }
# Using the Hash.new method
my_hash = Hash.new
my_hash["key1"] = "value1"
my_hash["key2"] = "value2"
Accessing values
You can access a value in a hash by its key using square brackets [] or the Hash#fetch method. Here are some examples:
# Using square brackets
my_hash["key1"] #=> "value1"
# Using the fetch method
my_hash.fetch("key2") #=> "value2"
Note: If you try to access a key that doesn’t exist in the hash, you will get a nil value. You can also set a default value to be returned if the key doesn’t exist, like this:
my_hash.fetch("key3", "default_value") #=> "default_value"
Iterating over a hash
You can iterate over a hash using the Hash#each method, which yields each key-value pair to a block. Here’s an example:
my_hash.each do |key, value|
puts "#{key}: #{value}"
end
This will output:
key1: value1
key2: value2
Modifying a hash
You can modify a hash by assigning a new value to an existing key or adding a new key-value pair. Here are some examples:
# Assigning a new value to an existing key
my_hash["key1"] = "new_value"
my_hash #=> {"key1"=>"new_value", "key2"=>"value2"}
# Adding a new key-value pair
my_hash["key3"] = "value3"
my_hash #=> {"key1"=>"new_value", "key2"=>"value2", "key3"=>"value3"}