How to Use the Ruby is_a? Method
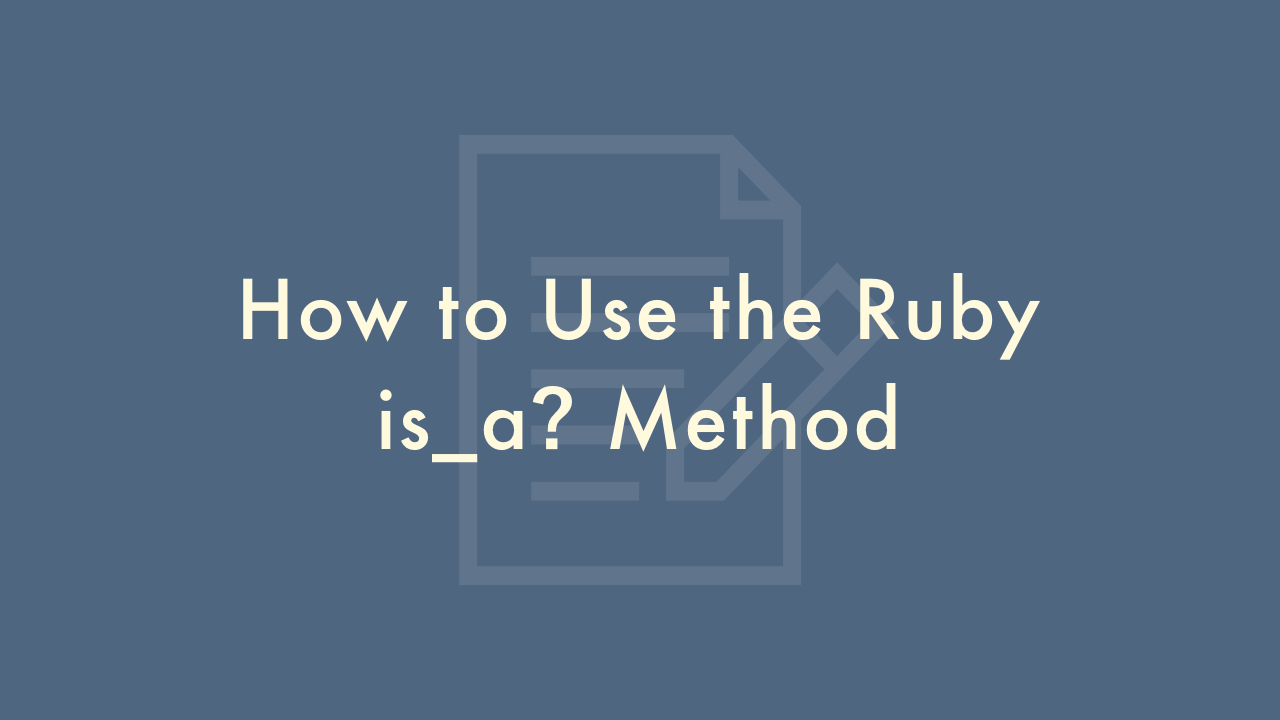
Contents
In this article, you will learn how to use the Ruby is_a? method.
Using the is_a? method
The is_a? method in Ruby is used to check if an object belongs to a particular class or its subclass. It returns a boolean value true if the object belongs to the specified class and false otherwise.
Syntax
The basic syntax for using is_a? method is as follows:
object.is_a?(class_name)
Here, object is the object to be checked and class_name is the name of the class to which the object is compared.
Examples
For example, to check if an object is a string, we can use the is_a? method as follows:
string = "Hello, World!"
puts string.is_a?(String) # Output: true
In this example, the is_a? method returns true because the string object belongs to the String class.
We can also use is_a? to check if an object is an instance of a subclass. For example, consider the following code:
class Animal
end
class Dog < Animal
end
dog = Dog.new
puts dog.is_a?(Animal) # Output: true
In this example, we have two classes, Animal and Dog. The Dog class is a subclass of Animal. We create an object of the Dog class and then use the is_a? method to check if the dog object belongs to the Animal class. Since Dog is a subclass of Animal, the is_a? method returns true.
We can also use is_a? method to check if an object belongs to multiple classes. For example:
module Swim
end
class Fish
include Swim
end
fish = Fish.new
puts fish.is_a?(Fish) # Output: true
puts fish.is_a?(Swim) # Output: true
In this example, we define a module Swim and a class Fish which includes the Swim module. We create an object of the Fish class and then use the is_a? method to check if the fish object belongs to the Fish class and the Swim module. Since Fish includes Swim, the is_a? method returns true for both the classes.