How to Use the Ruby map Method
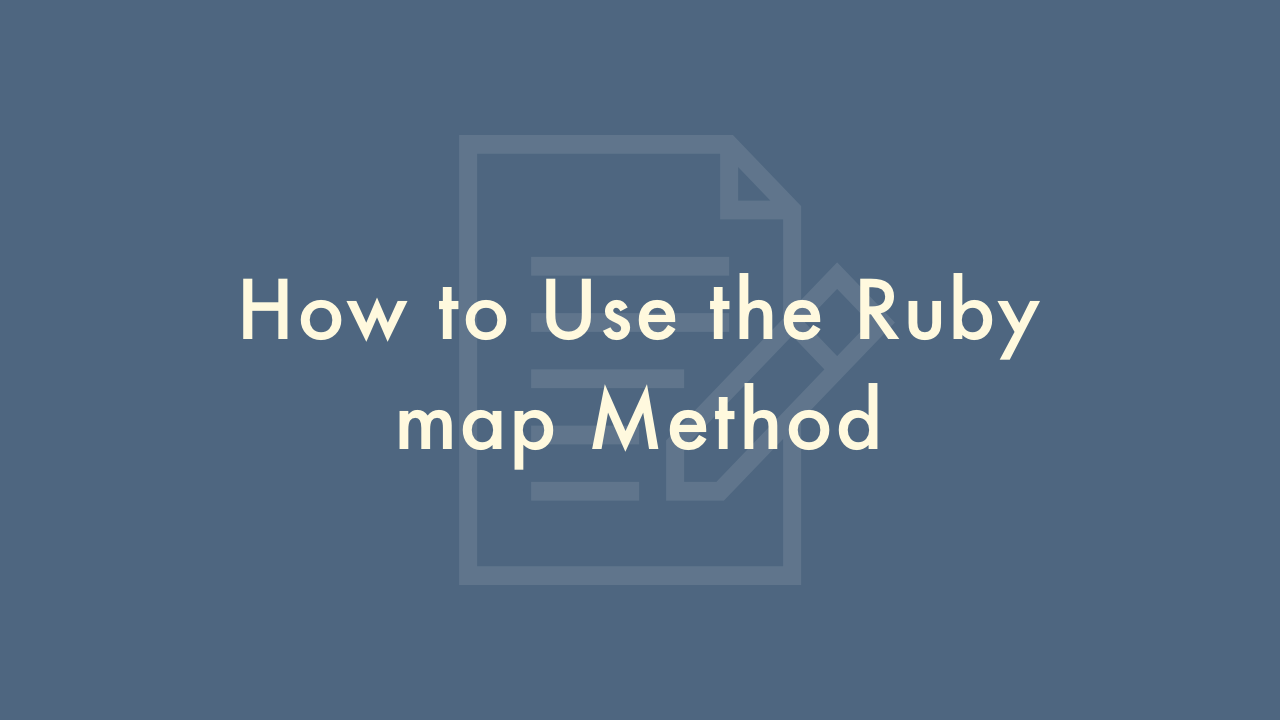
Contents
In this article, you will learn how to use the Ruby map method.
Using the map method
The Ruby map method is a built-in method that allows you to apply a transformation to every element of an enumerable object, such as an array or a hash. The map method takes a block as an argument and returns a new array with the transformed values.
Here’s how to use the map method:
Start by defining an enumerable object, such as an array:
array = [1, 2, 3, 4, 5]
Call the map method on the enumerable object and pass in a block that defines the transformation you want to apply to each element:
new_array = array.map { |element| element * 2 }
In this example, we’re multiplying each element of the array by 2.
The map method returns a new array with the transformed values:
puts new_array # Output: [2, 4, 6, 8, 10]
You can also use the shorthand syntax for blocks that consist of a single expression:
new_array = array.map { |element| element * 2 }
This is equivalent to:
new_array = array.map do |element|
element * 2
end
You can use the map method with any enumerable object, including hashes:
hash = { a: 1, b: 2, c: 3 }
new_hash = hash.map { |key, value| [key.to_s, value * 2] }.to_h
puts new_hash # Output: {"a" => 2, "b" => 4, "c" => 6}
In this example, we’re transforming each value of the hash by multiplying it by 2 and converting the keys to strings.