How to Use the Ruby step Method
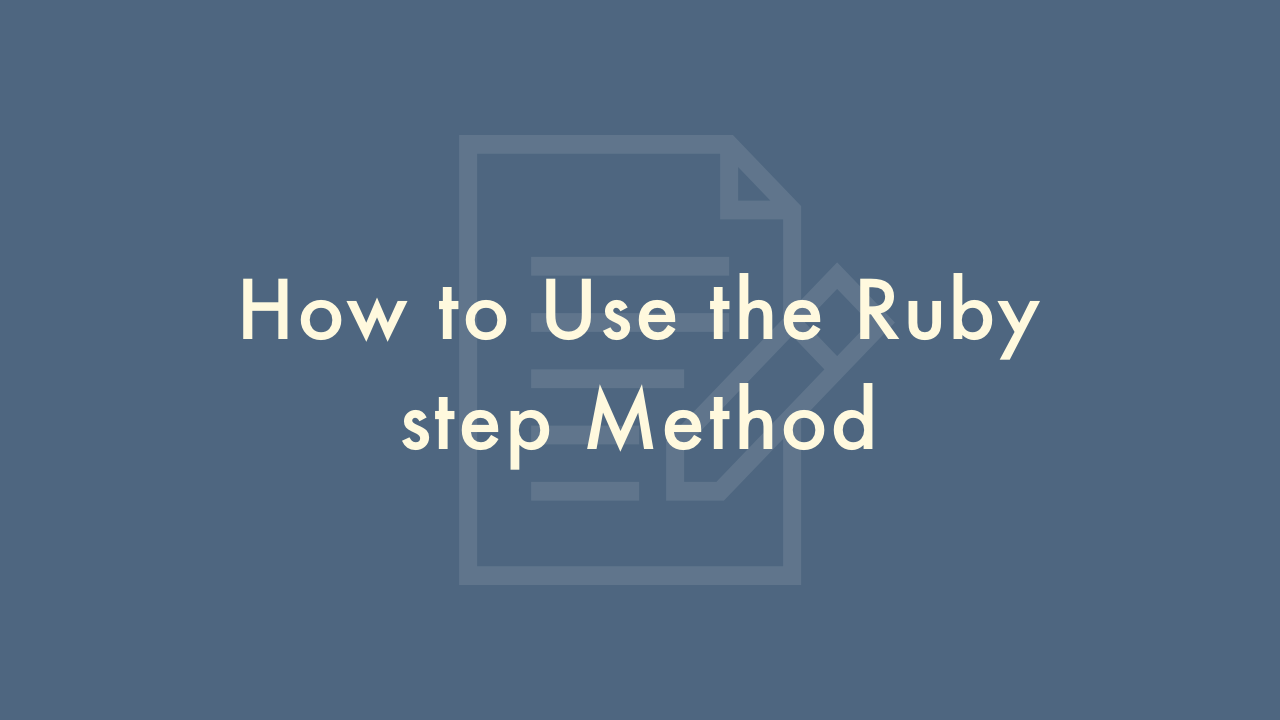
Contents
In this article, you will learn how to use the Ruby step method.
Using the step method
The step method is a built-in method in Ruby that allows you to iterate over a range of numbers by specifying a step value. Here’s how you can use the step method in your Ruby code:
Syntax
range.step(step_value) { |value| block }
Parameters
range
: The range of numbers to iterate over.step_value
: The value to increment the iteration by.value
: The current value of the iteration.
Examples
(1..10).step(2) { |i| puts i }
This code will print out the numbers 1, 3, 5, 7, and 9. In this example, the step method is called on a range of numbers (1..10) with a step value of 2. The block passed to the step method will be executed for each value in the range, starting with the first value (1) and incrementing by the step value (2) until the end of the range is reached.
You can also use the step method on arrays and other enumerables in Ruby. Here’s an example:
array = [1, 2, 3, 4, 5]
array.step(2) { |i| puts i }
This code will print out the numbers 1, 3, and 5. In this example, the step method is called on an array with a step value of 2. The block passed to the step method will be executed for each value in the array, starting with the first value (1) and incrementing by the step value (2) until the end of the array is reached.
You can also use negative step values to iterate backwards. Here’s an example:
(10..1).step(-2) { |i| puts i }
This code will print out the numbers 10, 8, 6, 4, and 2. In this example, the step method is called on a range of numbers (10..1) with a step value of -2. The block passed to the step method will be executed for each value in the range, starting with the first value (10) and decrementing by the step value (-2) until the end of the range is reached.