How to Use the chop Method in Ruby
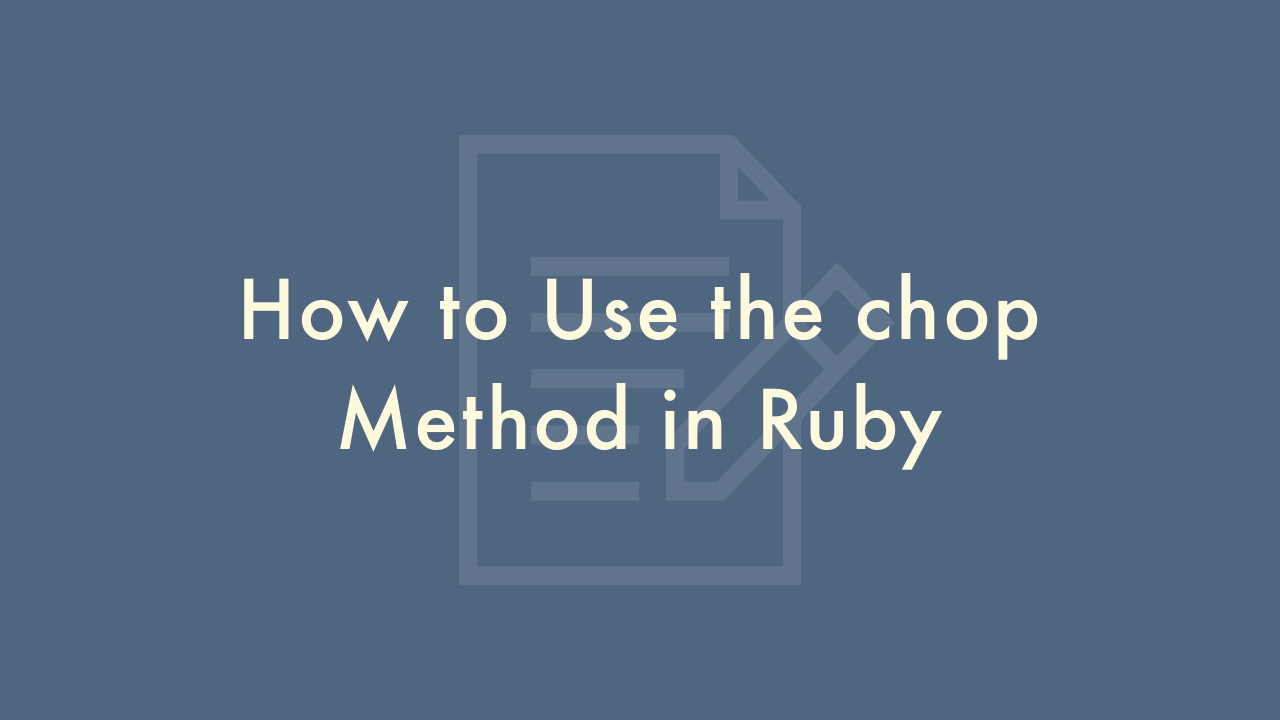
Contents
In this article, you will learn how to use the chop method in Ruby.
The chop Method
The chop method in Ruby removes the last character from a string and returns the modified string. Here’s how you can use it:
string = "Hello, world!\n"
chopped_string = string.chop
puts chopped_string
In the above code, the string variable holds the value “Hello, world!\n”, which includes a newline character (\n) at the end of the string.
The chop method is called on the string variable, which removes the last character (the newline character in this case) from the string and returns the modified string. The modified string is then stored in the chopped_string variable.
Finally, the puts method is used to output the modified string to the console, which results in the string “Hello, world!” being printed without the newline character.
Here are a few more things to keep in mind when using the chop method in Ruby:
- The chop method only removes the last character of a string. If you want to remove multiple characters from the end of a string, you can use the slice method or string slicing syntax with negative indices.
- If the last character of the string is a newline character (\n), the chop method will remove it. If the last character is not a newline character, the chop method will remove the last character regardless.
-
The chop method modifies the original string object in place. If you want to create a new string object with the last character removed, you can use the chop method in combination with string interpolation:
string = "Hello, world!\n" new_string = "#{string.chop} Goodbye, world!" puts new_string
In the above code, the chop method is used to remove the newline character from the end of the string variable, and the resulting modified string is used in a new string created with string interpolation. The new string “Hello, world! Goodbye, world!” is then output to the console.
-
If you want to remove a specific character from a string, rather than just the last character, you can use the gsub method with an empty replacement string:
string = "Hello, world!" new_string = string.gsub("o", "") puts new_string
In the above code, the gsub method is used to remove all occurrences of the letter “o” from the string variable, resulting in the new string “Hell, wrld!”.