How to Use form_with in Ruby on Rails
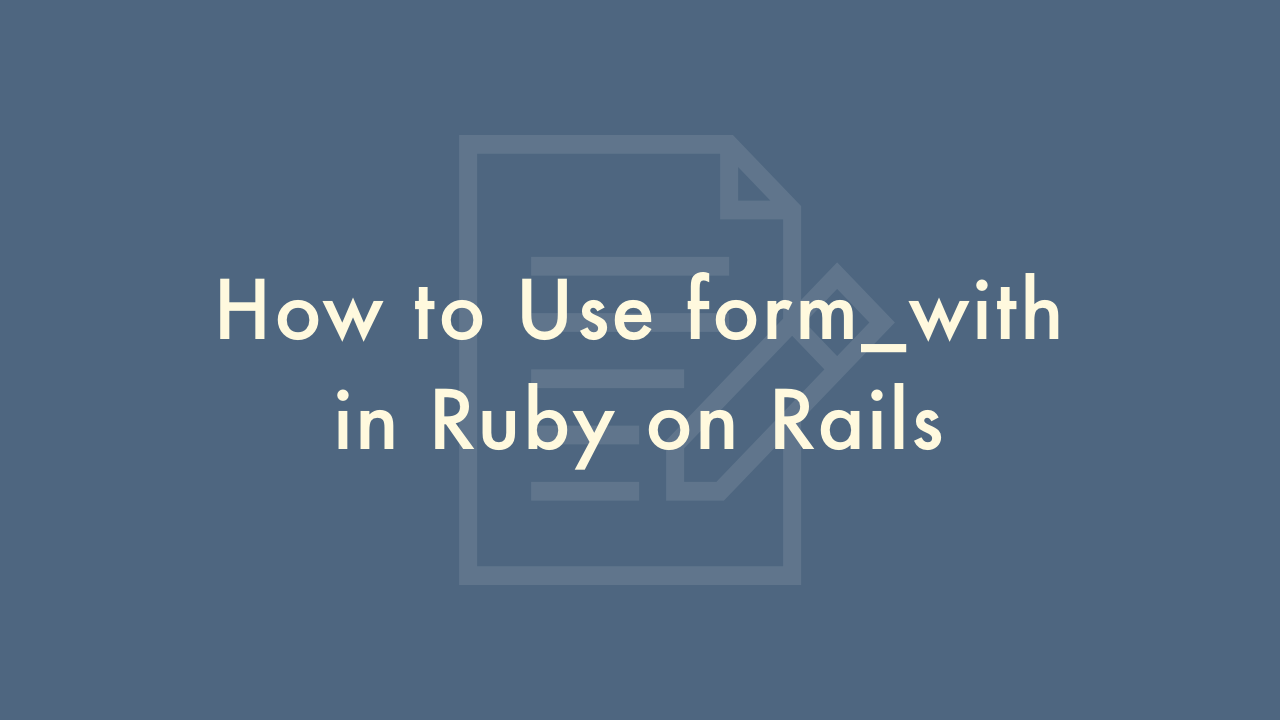
Contents
In this article, you will learn how to use form_with in Ruby on Rails.
Using form_with in Ruby on Rails
form_with is a helper method in Ruby on Rails that generates an HTML form tag that can be used to submit data to a server. Here’s how you can use it:
-
In your Rails view file (usually with an .html.erb extension), create a form using the form_with method. For example:
<%= form_with url: '/users', method: :post do |form| %> <!-- form fields go here --> <% end %>
-
The url parameter specifies the URL that the form data will be submitted to, and the method parameter specifies the HTTP method that will be used to submit the form data (e.g. POST or PUT).
-
Inside the form block, you can add form fields using Rails form helpers, such as form.label, form.text_field, and form.submit. For example:
<%= form_with url: '/users', method: :post do |form| %> <%= form.label :name %> <%= form.text_field :name %> <%= form.label :email %> <%= form.email_field :email %> <%= form.submit 'Create User' %> <% end %>
-
When the form is submitted, the data will be sent to the specified URL using the specified HTTP method. In your Rails controller, you can access the form data using the params hash. For example:
class UsersController < ApplicationController def create @user = User.new(user_params) if @user.save # redirect to success page else # render the form again with errors end end private def user_params params.require(:user).permit(:name, :email) end end
Here, the user_params method uses the params hash to extract the submitted form data and permit only the specified attributes (name and email). This helps to protect against mass assignment vulnerabilities.