How to Sort Hash in Ruby
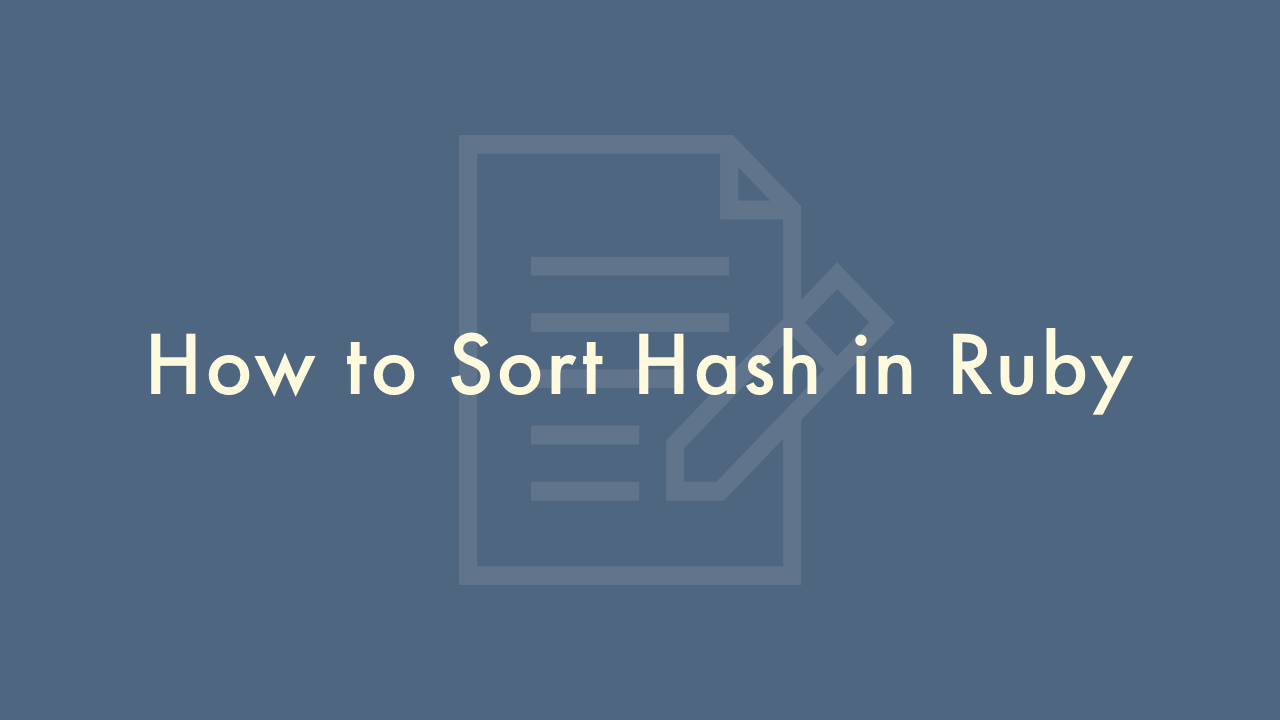
Contents
In this article, you will learn how to sort hash in Ruby.
Sorting hash in Ruby
In Ruby, hashes are unordered collections of key-value pairs. However, you can sort the keys or values of a hash in different ways. Here are some ways to sort a hash in Ruby:
Sorting by key
hash = { "b" => 3, "a" => 1, "c" => 2 }
sorted_hash = hash.sort.to_h
# => {"a"=>1, "b"=>3, "c"=>2}
In this example, the sort method is called on the hash, which returns an array of key-value pairs sorted by key. The to_h method is called on the resulting array to convert it back to a hash.
Sorting by value
hash = { "b" => 3, "a" => 1, "c" => 2 }
sorted_hash = hash.sort_by { |k, v| v }.to_h
# => {"a"=>1, "c"=>2, "b"=>3}
In this example, the sort_by method is called on the hash with a block that sorts the key-value pairs by value. The resulting array is converted back to a hash using the to_h method.
Sorting by both key and value
hash = { "b" => 3, "a" => 1, "c" => 2 }
sorted_hash = hash.sort_by { |k, v| [k, v] }.to_h
# => {"a"=>1, "b"=>3, "c"=>2}
In this example, the sort_by method is called on the hash with a block that sorts the key-value pairs by an array of key and value. The resulting array is converted back to a hash using the to_h method.